Utilizing PHP’s Built-in Server: A Basic Tutorial
For PHP developers, local development and testing are crucial stages of the software development lifecycle. Setting up a development environment usually involves configuring a web server like Apache or Nginx. However, PHP comes with a built-in server that offers a quick and convenient alternative for local development. In this tutorial, we’ll explore PHP’s built-in server, understand its benefits, and learn how to utilize it effectively.
1. Understanding PHP’s Built-in Server
The PHP built-in server, introduced in PHP 5.4, is a command-line tool designed to simplify the process of testing and developing PHP applications on a local machine. It’s lightweight, easy to use, and eliminates the need for a full-fledged web server for basic development tasks.
2. Getting Started
Before we dive into the details, ensure you have PHP installed on your machine. Open a terminal or command prompt and type:
bash php -v
If PHP is installed, you’ll see the version number displayed. If not, download and install PHP from the official PHP website.
3. Running the Built-in Server
Starting PHP’s built-in server is straightforward. Open your terminal or command prompt, navigate to the root directory of your PHP project, and run the following command:
bash php -S localhost:8000
Here, we use the -S flag to specify the server and port we want to use. In this case, we’re using localhost as the server address and 8000 as the port. You can choose a different port number if needed.
4. Accessing Your Application
Once the server is up and running, open your web browser and navigate to http://localhost:8000. You should see your PHP application’s index page (usually index.php) if it exists in the root directory. The built-in server will serve the application directly without the need for additional server configurations.
5. Specifying a Different Document Root
By default, the PHP built-in server uses the current working directory as the document root. However, if your application’s entry point is in a different directory, you can specify a custom document root using the -t flag:
bash php -S localhost:8000 -t path/to/document/root
Replace path/to/document/root with the actual path to your desired document root.
6. Handling PHP INI Settings
In most cases, the built-in server works seamlessly with your PHP configuration. However, there might be situations where you need to adjust specific PHP settings. You can use the -c flag to point to a custom php.ini file:
bash php -S localhost:8000 -c path/to/php.ini
This is especially useful when you want to enable or disable certain PHP extensions or set custom values for PHP variables during local development.
7. Enabling Error Reporting
During development, it’s essential to see PHP errors and warnings to fix potential issues. By default, the built-in server doesn’t display errors in the browser. You can enable error reporting by setting the display_errors and error_reporting directives in your custom php.ini file:
ini display_errors = On error_reporting = E_ALL
8. Handling URL Rewriting
If your PHP application relies on URL rewriting (e.g., using .htaccess rules in Apache), you need to configure the built-in server to handle them. Unfortunately, the built-in server does not support .htaccess files. Instead, you can use a router script to handle URL rewriting. Create a router.php file in your document root and add the following content:
php <?php if (preg_match('/\.(?:png|jpg|jpeg|gif|css|js)$/', $_SERVER["REQUEST_URI"])) { return false; } else { include 'index.php'; }
This script checks if the requested URL matches common file types (images, CSS, or JavaScript) and serves them directly. Otherwise, it includes your index.php file to handle the URL rewriting.
9. Handling PHP Sessions
The built-in server supports PHP sessions without additional configurations. It automatically manages session handling and allows you to test session-related functionalities seamlessly.
10. Limitations and Security Considerations
While PHP’s built-in server is a handy tool for local development, it’s important to note its limitations. The built-in server is not intended for production use and lacks some features available in full-fledged web servers like Apache or Nginx. Additionally, it may not handle complex or specific server configurations required for some applications.
When using the built-in server for local development, always ensure that your application is secure. Avoid exposing it to the public internet and restrict access to authorized users. For production deployment, opt for a suitable web server with the necessary security measures.
Conclusion
PHP’s built-in server is an invaluable tool for developers seeking a quick and easy way to test and develop PHP applications locally. Its simplicity and convenience make it an ideal choice for small projects and rapid prototyping. However, remember its limitations and avoid using it in a production environment.
In this tutorial, we explored how to get started with PHP’s built-in server, customized its configurations, handled URL rewriting, and addressed security considerations. Armed with this knowledge, you can now harness the power of PHP’s built-in server to streamline your local development workflow and boost your productivity. Happy coding!
Table of Contents
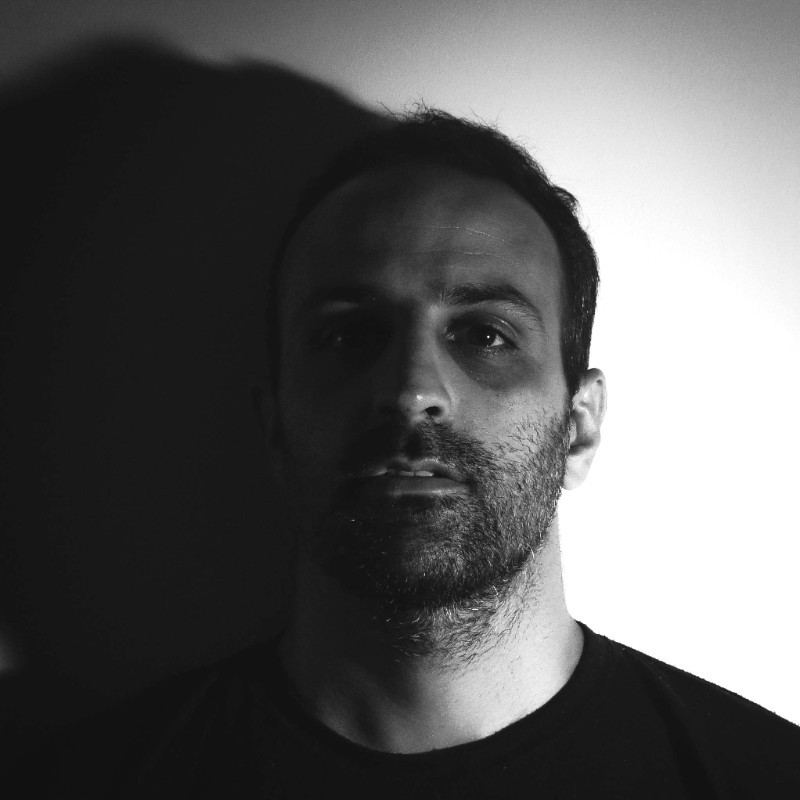
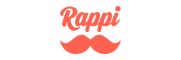