Creating a PHP Contact Form: A Detailed Tutorial
In today’s digital age, maintaining effective communication with your website visitors is crucial. One of the most common ways to achieve this is by incorporating a contact form on your website. A contact form not only provides a user-friendly interface for visitors to reach out to you, but it also helps in streamlining your communication process. In this tutorial, we will guide you through the process of creating a PHP contact form from scratch.
Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites in place:
- Web Server: You’ll need access to a web server with PHP support. You can use popular options like Apache, Nginx, or even local development environments like XAMPP and WAMP.
- Text Editor: Choose a text editor or integrated development environment (IDE) for writing your code. Some recommended options include Visual Studio Code, Sublime Text, or PhpStorm.
1. HTML Form Structure
Let’s start by creating the HTML structure for our contact form. Open a new file and name it contact.php. Here’s a basic structure to get you started:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Contact Us</title> </head> <body> <h1>Contact Us</h1> <form action="process_form.php" method="POST"> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="message">Message:</label> <textarea id="message" name="message" required></textarea> <button type="submit">Submit</button> </form> </body> </html>
In the code above, we’ve created a simple HTML form with fields for the user’s name, email, and message. The form’s action attribute points to a PHP script that will process the form data.
2. PHP Form Handling
Now, let’s create the PHP script that will handle the form submission and send the email. Create a new file named process_form.php and add the following code:
php <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST["name"]; $email = $_POST["email"]; $message = $_POST["message"]; $to = "your@email.com"; // Replace with your email address $subject = "New Contact Form Submission"; $headers = "From: $email"; if (mail($to, $subject, $message, $headers)) { echo "Thank you for contacting us!"; } else { echo "Oops! Something went wrong."; } } ?>
In this PHP script, we first check if the form has been submitted using the $_SERVER[“REQUEST_METHOD”] variable. If it’s a POST request, we retrieve the form data using the $_POST superglobal and assign it to variables. Then, we set up the email parameters, including the recipient’s email address, subject, and headers. The mail() function is used to send the email, and based on its success or failure, an appropriate message is displayed to the user.
3. Adding Form Validation
Form validation is essential to ensure that the data submitted is accurate and complete. Let’s enhance our PHP script to include basic form validation. Update the process_form.php file as follows:
php <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST["name"]; $email = $_POST["email"]; $message = $_POST["message"]; $errors = array(); if (empty($name)) { $errors[] = "Name is required"; } if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $errors[] = "Invalid email format"; } if (empty($message)) { $errors[] = "Message is required"; } if (empty($errors)) { $to = "your@email.com"; // Replace with your email address $subject = "New Contact Form Submission"; $headers = "From: $email"; if (mail($to, $subject, $message, $headers)) { echo "Thank you for contacting us!"; } else { echo "Oops! Something went wrong."; } } else { foreach ($errors as $error) { echo $error . "<br>"; } } } ?>
In this updated script, we’ve introduced an array called $errors to store validation error messages. We’ve added checks for empty fields and an invalid email format using the filter_var() function. If the $errors array is empty, the email is sent as before. However, if there are validation errors, they are displayed to the user.
4. Styling and Further Customization
While the contact form is now functional, you can enhance its appearance and functionality by adding CSS styles and additional features. Here’s a quick example of how you could style the form:
css /* Add this to your CSS file */ body { font-family: Arial, sans-serif; margin: 0; padding: 0; background-color: #f4f4f4; } .container { max-width: 600px; margin: auto; padding: 20px; background-color: #fff; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); } form { margin-top: 20px; } label { display: block; margin-bottom: 5px; font-weight: bold; } input[type="text"], input[type="email"], textarea { width: 100%; padding: 10px; margin-bottom: 10px; border: 1px solid #ccc; border-radius: 4px; } button { padding: 10px 20px; background-color: #007bff; color: #fff; border: none; border-radius: 4px; cursor: pointer; }
By adding this CSS code to your stylesheets, you can give your contact form a clean and professional appearance. You can also consider adding features like CAPTCHA verification, file uploads, or saving form submissions to a database for future reference.
Conclusion
Congratulations! You’ve successfully created a functional PHP contact form from scratch. This tutorial covered the HTML structure, PHP scripting for form handling and validation, and a basic styling example. Remember, a well-designed contact form enhances user experience and facilitates communication between you and your website visitors. Feel free to further customize and expand upon this tutorial to suit your specific needs. Happy coding!
Table of Contents
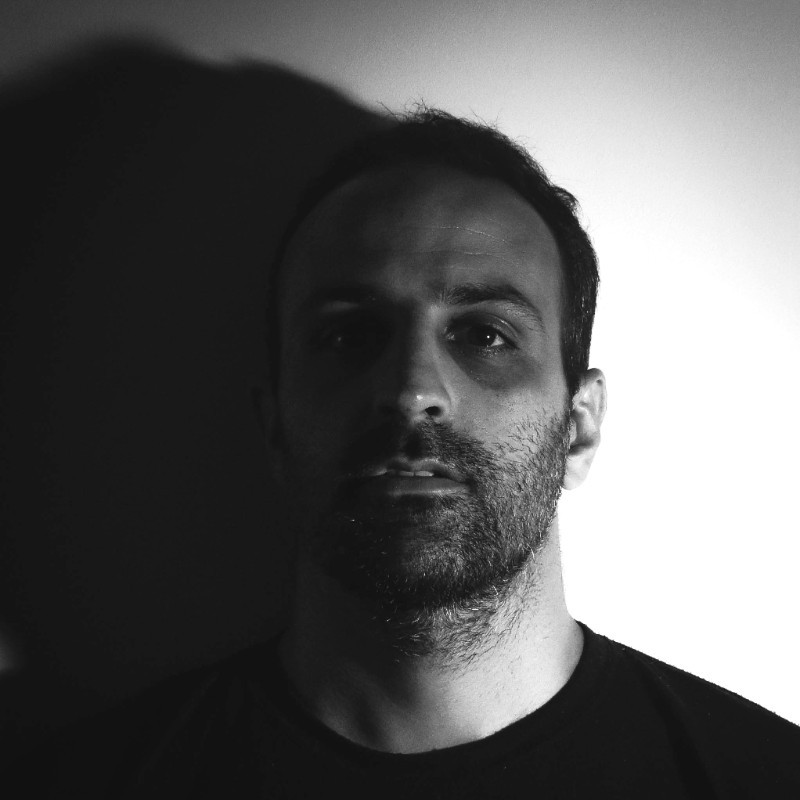
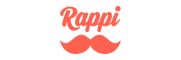