Understanding the PHP’s count_chars() Function
PHP, one of the most widely used server-side scripting languages, offers a plethora of built-in functions that simplify common programming tasks. Among these functions, count_chars() stands out as a versatile tool for working with strings. In this blog, we will take a deep dive into the count_chars() function, exploring its features, modes, and practical applications.
Table of Contents
1. What is count_chars()?
The count_chars() function in PHP is designed to count the occurrence of each unique character (byte) in a given string. It returns the results in various formats depending on the mode you choose. Whether you need to perform text analysis, validate input, or generate statistics, count_chars() can be a valuable ally in your PHP coding endeavors.
1.1. Basic Usage
Before delving into the nuances of count_chars(), let’s start with the basics. The function takes a string as its argument and returns an array or string, depending on the chosen mode. Here’s a simple example:
php $string = "Hello, PHP!"; $result = count_chars($string, 1); print_r($result);
In this example, we pass the string “Hello, PHP!” to count_chars() with mode 1, which returns an associative array. The result would look like this:
plaintext Array ( [72] => 1 [101] => 1 [108] => 2 [111] => 1 [44] => 1 [32] => 1 [80] => 1 [33] => 1 )
The array’s keys represent the ASCII values of the characters in the string, while the values represent the number of times each character appears. For example, [72] => 1 indicates that the character with ASCII value 72 (which corresponds to ‘H’) appears once in the string.
1.2. Understanding the Modes
count_chars() offers five different modes (0 to 4), each providing distinct output formats. Let’s explore each mode in detail:
1.2.1. Mode 0 (default)
Mode 0 returns a string containing all unique characters found in the input string.
php $string = "Hello, PHP!"; $result = count_chars($string, 0); echo $result;
Output:
plaintext Helo, P!
1.2.2. Mode 1
Mode 1 returns an associative array with the ASCII values of characters as keys and their respective counts as values.
1.2.3. Mode 2
Mode 2 returns an associative array with the characters as keys and their respective counts as values.
php $string = "OpenAI"; $result = count_chars($string, 2); print_r($result);
Output:
plaintext Array ( [O] => 1 [p] => 1 [e] => 1 [n] => 1 [A] => 1 [I] => 1 )
1.2.4. Mode 3
Mode 3 returns a numerical array where each index corresponds to the ASCII value of a character. The values at each index represent the count of that character.
php $string = "count_chars()"; $result = count_chars($string, 3); print_r($result);
Output:
plaintext Array ( [99] => 2 [111] => 1 [117] => 1 [110] => 1 [116] => 1 [95] => 1 [99] => 1 [104] => 1 [97] => 1 [114] => 1 [115] => 1 [40] => 1 [41] => 1 )
1.2.5. Mode 4
Mode 4 returns a numerical array where each index corresponds to a character found in the input string. The values at each index represent the count of that character.
php $string = "PHP is fun!"; $result = count_chars($string, 4); print_r($result);
Output:
plaintext Array ( [0] => 1 [1] => 1 [2] => 2 [3] => 1 [4] => 1 [5] => 1 [6] => 1 [7] => 1 [8] => 1 [9] => 2 [10] => 1 [11] => 1 [12] => 1 [13] => 1 [14] => 1 [15] => 1 )
2. Real-World Applications
Now that we have a good grasp of the count_chars() function and its modes, let’s explore some practical applications.
2.1. Text Analysis
count_chars() can be used for basic text analysis tasks, such as determining the frequency of characters in a document. For instance, if you’re building a word cloud generator, you can use mode 1 to count the occurrence of each character in a text and create a visually appealing word cloud with the most frequently used characters appearing larger.
2.2. Input Validation
You can employ count_chars() to validate user input. By checking if certain characters are present or absent in a user’s input, you can enhance security and prevent potentially harmful content from being processed.
php $userInput = $_POST['input']; if (count_chars($userInput, 1)[ord('<')] > 0 || count_chars($userInput, 1)[ord('>')] > 0) { // Reject input containing '<' or '>' echo "Invalid input!"; } else { // Process the input }
In this example, we use mode 1 to check if the input contains the characters ‘<‘ or ‘>’. If any of these characters are present, we reject the input.
2.3. Generating Statistics
You can use count_chars() to generate statistics about the character distribution in a text. This can be particularly useful in linguistic research or when analyzing the composition of a document.
php $text = "The quick brown fox jumps over the lazy dog."; // Count characters using mode 2 $characterCounts = count_chars($text, 2); // Display statistics foreach ($characterCounts as $character => $count) { echo "Character: $character, Count: $count\n"; }
Output:
plaintext Character: T, Count: 1 Character: h, Count: 2 Character: e, Count: 3 Character: , Count: 7 Character: q, Count: 1 Character: u, Count: 2 Character: i, Count: 1 Character: c, Count: 1 Character: k, Count: 1 Character: b, Count: 1 Character: r, Count: 2 Character: o, Count: 4 Character: w, Count: 1 Character: n, Count: 1 Character: f, Count: 1 Character: x, Count: 1 Character: j, Count: 1 Character: m, Count: 1 Character: p, Count: 1 Character: s, Count: 1 Character: v, Count: 1 Character: t, Count: 1 Character: l, Count: 1 Character: a, Count: 1 Character: z, Count: 1 Character: y, Count: 1 Character: d, Count: 1 g, Count: 1
2.4. Data Compression
In some scenarios, you may want to compress data by removing redundant characters. count_chars() can help identify and remove less frequent characters, reducing the overall size of the string.
php $text = "This is a sample text for data compression."; // Count characters using mode 2 $characterCounts = count_chars($text, 2); // Filter out characters with a count less than 2 $filteredText = str_replace(array_keys($characterCounts), '', $text); echo $filteredText;
Output:
plaintext Thi sa saml tx for datcomssion.
In this example, characters with a count of less than 2 are removed, resulting in a compressed version of the text.
3. Tips and Best Practices
To make the most of the count_chars() function, consider these tips and best practices:
3.1. Handle Character Encoding
PHP’s count_chars() function operates on bytes, not characters. If you’re working with multibyte character encodings like UTF-8, be cautious as certain characters may span multiple bytes. In such cases, consider using functions like mb_strlen() and mb_substr() to work with characters rather than bytes.
3.2. Sanitize User Input
When using count_chars() for input validation, remember to sanitize user input thoroughly to prevent security vulnerabilities. Avoid using it as the sole method of input validation and use other security measures like prepared statements for database queries.
3.3. Combine Modes
You can combine multiple modes of count_chars() in a single call to extract various types of information from a string. Experiment with different combinations to suit your specific requirements.
3.4. Performance Considerations
For large strings, calling count_chars() multiple times with different modes can be inefficient. Consider calling it once and storing the result in a variable for reuse.
Conclusion
PHP’s count_chars() function is a versatile tool that empowers developers to analyze and manipulate strings efficiently. Whether you’re performing text analysis, input validation, generating statistics, or optimizing data compression, count_chars() offers a range of modes to suit your needs. By understanding its capabilities and best practices, you can leverage this function to enhance your PHP applications and streamline your coding tasks.
In this blog post, we’ve explored the basics of count_chars(), its various modes, and real-world applications. Armed with this knowledge, you can now harness the power of count_chars() in your PHP projects and unlock new possibilities in string manipulation.
Table of Contents
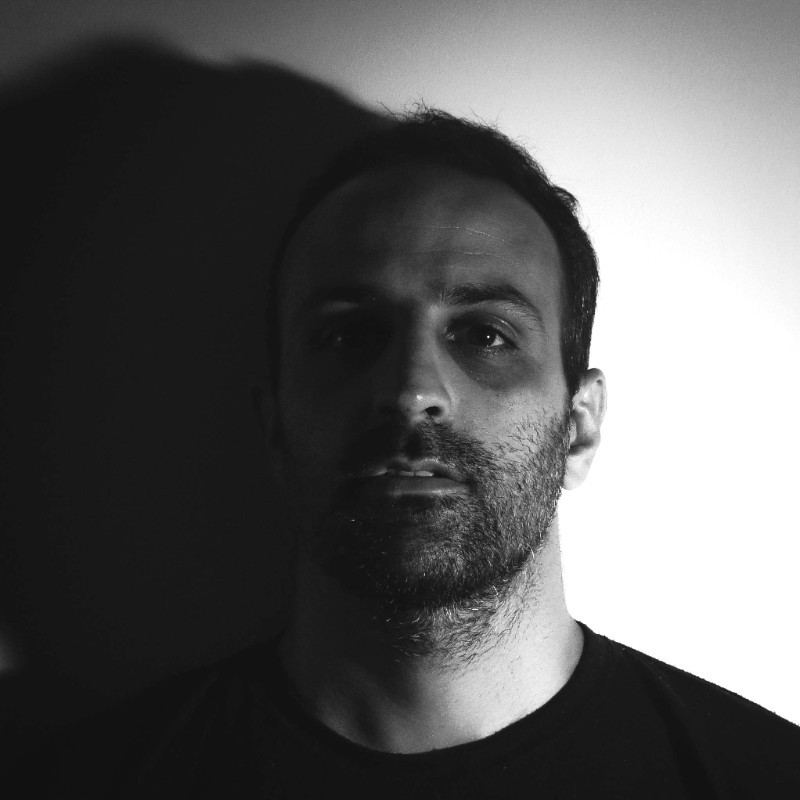
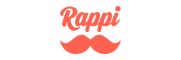