Creating Dynamic Web Pages with PHP
The backbone of the internet is its websites, and the foundation of these websites is the code that creates them. A static webpage suffices for basic information, but modern web users crave websites that are dynamic, changing, and interactive. PHP, a robust and widely-used language, is the key to building such dynamic web pages. Whether you’re an aspiring coder looking to delve into the realm of PHP or a business owner seeking to hire professional PHP developers to enhance your website’s interactivity, today’s exploration into the world of PHP will guide you. We will explore how PHP can be effectively utilized to create dynamic web pages that truly engage visitors.
What is PHP?
PHP, an acronym for “PHP: Hypertext Preprocessor,” is a server-side scripting language designed specifically for web development. This open-source language allows developers to create dynamic content that interacts with databases, making it an ideal language for building web-based software applications.
How PHP Works
Unlike HTML, which your browser reads and then displays, PHP scripts are executed on the server. The PHP interpreter installed on the server processes the PHP scripts. The results of the script execution, usually HTML or XML, are then sent back to the client’s browser for display. Because PHP is server-side, the client’s browser never sees the actual PHP code, only the output it produces. This distinction makes PHP a powerful tool for creating dynamic and secure websites. For businesses not equipped to handle PHP in-house, hiring professional PHP developers can provide the expertise needed to leverage this powerful language and maximize your website’s potential.
Creating a Dynamic Web Page with PHP
1. Setting Up:
To get started with PHP, you need a server with PHP and MySQL installed. You can use an online web host, or for development purposes, you can set up a local server environment like XAMPP, WAMP, or MAMP.
2. Creating Your First PHP File:
A PHP file is similar to an HTML file, but it ends with a .php extension. Open a new file in your text editor of choice, and save it as “index.php“.
<!DOCTYPE html> <html> <body> <?php echo "Hello, World!"; ?> </body> </html>
When you open this file in a web browser, you should see the text “Hello, World!”. The `<?php ?>` tags enclose the PHP code, which is interpreted on the server.
3. PHP and HTML:
A great strength of PHP is its seamless integration with HTML. PHP can be embedded within HTML code, and it can generate HTML. This allows PHP to change the content of a webpage in real time.
<!DOCTYPE html> <html> <body> <h1>Welcome to my website</h1> <p>Today's date is: <?php echo date('Y-m-d'); ?> </p> </body> </html>
In the above example, PHP generates the current date dynamically every time the page is loaded.
4. Variables:
Variables in PHP are represented by a dollar sign followed by the name of the variable. They are dynamically typed, which means you don’t have to declare their data type. Variables can store data of different types like string, integer, float, boolean, array, or object.
<?php $greeting = "Hello, World!"; echo $greeting; ?>
In this code, “Hello, World!” is stored in the $greeting variable and then echoed out.
5. Forms and PHP:
Forms are an essential part of web interactivity. When a user submits a form, the data can be sent to a PHP file which then processes the data.
Here’s a simple form in HTML:
```HTML <form method="POST" action="welcome.php"> Name: <input type="text" name="name"><br> Email: <input type="text" name="email"><br> <input type="submit"> </form>
In the PHP file welcome.php, we can then access the data:
``PHP Welcome <?php echo $_POST["name"]; ?><br> Your email address is: <?php echo $_ POST["email"]; ?> ```
6. Connecting to a Database:
To create a truly dynamic website, you’ll want to interact with a database. MySQL is a common database choice with PHP. The mysqli extension allows PHP to interact with MySQL databases.
Here’s a simple example of connecting to a MySQL database:
```PHP <?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully"; ?>
Once you’re connected to the database, you can use PHP to create, read, update, and delete records, allowing you to create highly dynamic web content.
Conclusion
Creating dynamic web pages with PHP is a powerful way to engage your website’s visitors. With PHP, you can respond to user input, serve up-to-date content, and even interact with a database. Implementing these PHP-driven features allows for a richer, more personalized user experience. However, like any language, PHP takes time and dedication to master. For businesses seeking to implement these dynamic elements swiftly and efficiently, hiring expert PHP developers can be an excellent solution. These professionals can bring your website to life, enhancing its interactivity and keeping your content fresh and engaging. Remember, PHP is not just a tool, it’s a catalyst for crafting highly engaging web experiences. Happy coding!
Table of Contents
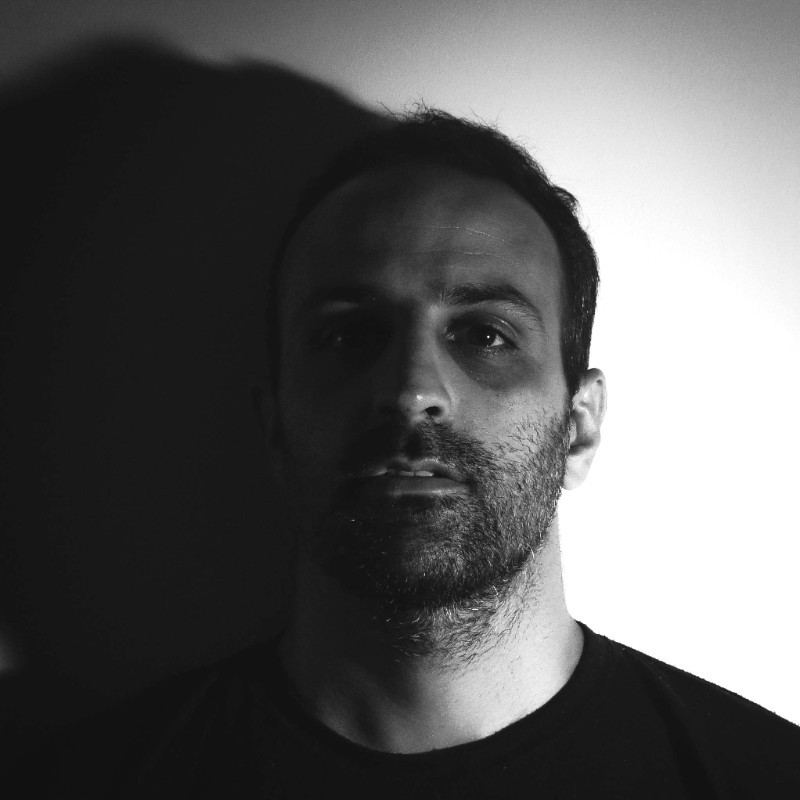
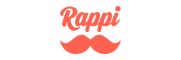