How to implement database caching?
Implementing database caching in PHP can significantly enhance the performance of your web applications by reducing the load on your database server. Caching involves storing frequently accessed database query results, database objects, or even entire web pages in a cache storage system, such as Memcached or Redis, for quick retrieval. Here’s a guide on how to implement database caching in PHP:
- Choose a Caching Solution:
Begin by selecting a caching solution that suits your project’s requirements. Memcached and Redis are popular choices due to their speed and scalability. These in-memory data stores allow you to store and retrieve data quickly.
- Install and Configure the Caching Server:
Install and configure your chosen caching server. For example, if you’re using Memcached, you’d need to install the Memcached server and PHP extension. Similarly, for Redis, you’d install the Redis server and PHP extension. Configure the caching server’s settings, such as cache expiration times and memory allocation, according to your project’s needs.
- Modify Your PHP Application:
In your PHP application code, identify the database queries or data retrieval operations that could benefit from caching. Wrap these operations with caching logic. Here’s a simplified example using Memcached:
```php $cacheKey = 'unique_key_for_your_data'; $cachedData = $cache->get($cacheKey); if (!$cachedData) { // If data is not in the cache, fetch it from the database $data = fetchDataFromDatabase(); // Store the data in the cache for future use $cache->set($cacheKey, $data, $expirationTime); } else { // Use the cached data $data = $cachedData; } ```
This code checks if the data exists in the cache (Memcached) before querying the database. If the data is present in the cache, it’s retrieved from there, reducing the load on the database server.
- Set Cache Expiration Times:
Determine appropriate cache expiration times for your data. Cache expiration ensures that the data remains fresh and up-to-date. Shorter expiration times might be suitable for frequently changing data, while longer times can be used for relatively static content.
- Clear Cache When Data Changes:
Implement a mechanism to clear or update cache entries when the underlying data changes. This ensures that cached data remains synchronized with the database.
- Monitor and Fine-Tune:
Regularly monitor your application’s performance and cache usage. Adjust cache expiration times and other settings based on usage patterns and changing data requirements.
By implementing database caching in your PHP applications, you can reduce the load on your database server, improve response times, and deliver a more responsive user experience. However, it’s essential to strike a balance between caching and ensuring that data remains consistent and up-to-date.
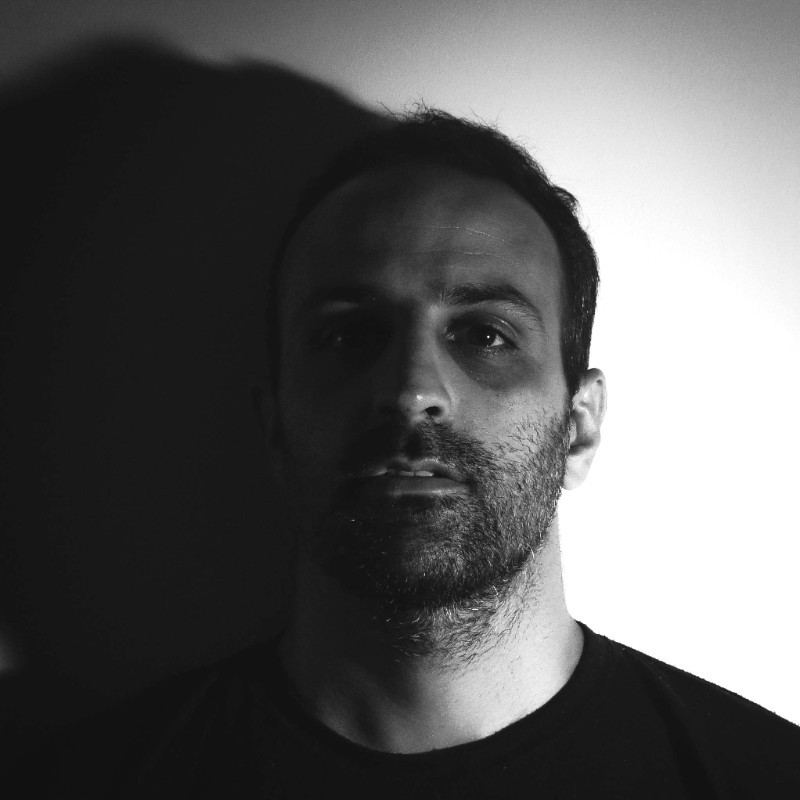
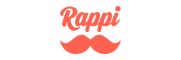