Understanding PHP’s date() Function: A Complete Guide
In the world of web development, handling dates and times is an essential task. Whether you’re building a blog, an e-commerce platform, or a social media application, the ability to manipulate and display dates accurately is crucial. PHP, a widely-used server-side scripting language, offers a powerful tool for this purpose: the date() function. In this guide, we’ll dive deep into understanding the date() function, exploring its features, formatting options, and providing practical examples.
1. Introduction to the date() Function
1.1. What is the date() function?
The date() function in PHP is used to format and display dates and times according to specified formats. It allows developers to extract various components of a date, such as the year, month, day, hour, minute, and second, and format them as needed. This function is highly versatile and plays a crucial role in generating dynamic content that includes time-sensitive information.
1.2. Why is it important in web development?
In web development, displaying dates accurately and in user-friendly formats is essential for providing a positive user experience. Whether you’re showing the publication date of an article, the last login time of a user, or the delivery date of a product, the ability to customize and format these dates enhances the usability and readability of your website or application. The date() function empowers developers to achieve this level of flexibility and precision.
2. Getting Started with date()
2.1. Basic syntax of the date() function
The basic syntax of the date() function is as follows:
php string date(string $format, int $timestamp = time())
The $format parameter specifies the format in which the date and time should be displayed.
The $timestamp parameter is optional and represents the Unix timestamp. If not provided, the current timestamp is used.
2.2. Displaying the current date and time
To display the current date and time, you can use the date() function with a simple format. For example:
php $currentDateTime = date("Y-m-d H:i:s"); echo "Current date and time: $currentDateTime";
In this example, the format “Y-m-d H:i:s” represents the year, month, day, hour, minute, and second in a structured manner.
3. Formatting Options
3.1. Common format characters
The date() function uses various format characters to represent different components of a date and time. Some common format characters include:
- Y: Year with 4 digits (e.g., 2023)
- m: Month with leading zeros (e.g., 08 for August)
- d: Day of the month with leading zeros (e.g., 05)
- H: Hour in 24-hour format with leading zeros (e.g., 15 for 3 PM)
- i: Minutes with leading zeros (e.g., 09)
- s: Seconds with leading zeros (e.g., 07)
3.2. Customizing date and time formats
You can combine these format characters to create custom date and time formats. For instance:
php $customFormat = date("F j, Y, g:i a"); echo "Formatted date and time: $customFormat";
In this example, the format “F j, Y, g:i a” would output a date like “August 7, 2023, 3:45 pm.”
3.3. Escape characters for special characters
If you want to include literal characters in your format string, you can escape them using a backslash (\). For example:
php $escapedFormat = date("The current year is Y \\and today is l."); echo $escapedFormat;
Here, the format string includes the escaped Y to represent the literal letter “Y” and the escaped l to represent the literal day of the week.
4. Timezones and date_default_timezone_set()
4.1. Working with different timezones
Dealing with timezones is a critical aspect of displaying accurate and relevant date and time information, especially in applications used by people around the world. You can set the timezone for your script using the date_default_timezone_set() function:
php date_default_timezone_set("America/New_York");
4.2. Setting the default timezone for your script
By setting the default timezone, you ensure that all date and time calculations and displays are adjusted according to the chosen timezone. This is particularly important when dealing with daylight saving time changes and ensuring consistency across user interactions.
5. Practical Examples
5.1. Displaying a copyright notice with the current year
A common use case is to display a copyright notice with the current year. You can achieve this using the date() function:
php $currentYear = date("Y"); echo "© $currentYear YourWebsiteName. All rights reserved.";
5.2. Creating a countdown timer
You can also use the date() function to create a countdown timer for events. Let’s say you have an event occurring on a specific date:
php $eventDate = strtotime("2023-12-31 23:59:59"); $currentDate = time(); $secondsRemaining = $eventDate - $currentDate; $daysRemaining = floor($secondsRemaining / (60 * 60 * 24)); $hoursRemaining = floor(($secondsRemaining % (60 * 60 * 24)) / (60 * 60)); $minutesRemaining = floor(($secondsRemaining % (60 * 60)) / 60); $secondsRemaining = $secondsRemaining % 60; echo "Countdown: $daysRemaining days, $hoursRemaining hours, $minutesRemaining minutes, $secondsRemaining seconds";
5.3. Formatting user-generated timestamps
When dealing with user-generated timestamps, you can use the date() function to format and display these timestamps in a user-friendly manner. For instance:
php $userTimestamp = "2023-07-15 18:30:00"; $formattedTimestamp = date("F j, Y, g:i a", strtotime($userTimestamp)); echo "User's timestamp: $formattedTimestamp";
6. Handling Time Intervals
6.1. Calculating differences between dates
The date() function can be used to calculate the difference between two dates. For example, to find the number of days between two dates:
php $startDate = strtotime("2023-08-01"); $endDate = strtotime("2023-08-15"); $daysDifference = ($endDate - $startDate) / (60 * 60 * 24); echo "Number of days between dates: $daysDifference";
6.2. Adding and subtracting time intervals
You can also add or subtract time intervals from a given date using the strtotime() function. For instance:
php $originalDate = "2023-08-01"; $daysToAdd = 7; $newDate = date("Y-m-d", strtotime($originalDate . " + $daysToAdd days")); echo "New date after adding $daysToAdd days: $newDate";
7. Localized Dates
7.1. Displaying dates in different languages
If your application serves a global audience, you might need to display dates in different languages. The setlocale() function allows you to set the locale for date formatting:
php setlocale(LC_TIME, "fr_FR"); $localizedDate = strftime("%A %d %B %Y"); echo "Localized date: $localizedDate";
7.2. Using the setlocale() function
By using the setlocale() function, you can adapt your date and time displays to the preferred language and format of the user.
8. Dealing with UNIX Timestamps
8.1. Converting between UNIX timestamps and human-readable dates
UNIX timestamps are often used to represent dates and times internally in databases and systems. You can convert them to human-readable dates using the date() function:
php $unixTimestamp = 1678924800; $readableDate = date("Y-m-d H:i:s", $unixTimestamp); echo "Converted date: $readableDate";
8.2. Working with timestamps in database operations
When interacting with databases, UNIX timestamps can simplify date-related operations, making sorting and querying more efficient.
9. Best Practices
9.1. Storing dates in standardized formats
When storing dates in databases or transmitting them between systems, using standardized formats like ISO 8601 (YYYY-MM-DD HH:MM:SS) ensures compatibility and consistency.
9.2. Handling daylight saving time changes
Be mindful of daylight saving time changes when working with different timezones. Use the appropriate timezone handling functions to avoid discrepancies.
9.3. Avoiding deprecated date functions
Some date functions in PHP are deprecated. It’s recommended to use modern alternatives like the DateTime class for better maintainability and compatibility.
Conclusion
In conclusion, the date() function in PHP is a versatile tool for working with dates and times in web development. Its flexibility, along with the ability to customize formats, work with timezones, and handle intervals, makes it an indispensable part of any developer’s toolkit. By mastering the date() function, you can ensure accurate and user-friendly displays of date and time information in your web applications.
Table of Contents
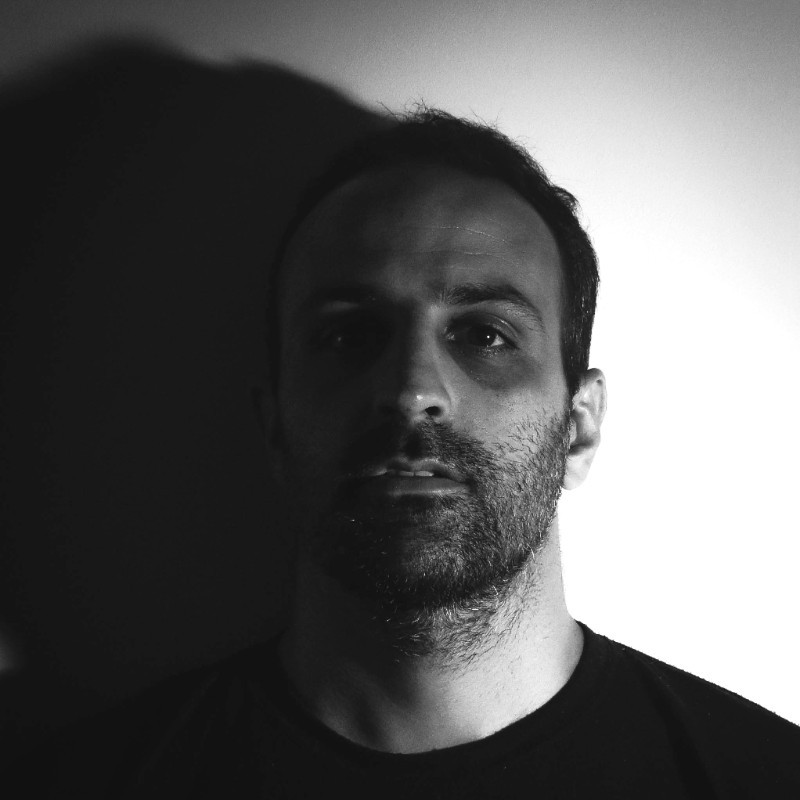
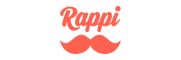