Leveraging PHP’s date_diff() Function: A Practical Guide
In the realm of web development, handling date and time is an essential task. Whether you’re building a blog with scheduled posts, creating an event management system, or simply displaying the date an article was published, mastering date and time manipulation in PHP is crucial. One valuable tool in your PHP toolkit is the date_diff() function. In this comprehensive guide, we will delve into the depths of this function, exploring its syntax, practical use cases, and best practices.
Table of Contents
1. Understanding date_diff()
Before we dive into practical examples, let’s gain a solid understanding of the date_diff() function in PHP.
1.1. Syntax
The date_diff() function calculates the difference between two DateTime objects, providing you with a DateInterval object that contains the difference. Its syntax looks like this:
php date_diff(DateTimeInterface $date1, DateTimeInterface $date2, bool $absolute = false): DateInterval|false
- $date1: The first DateTime object.
- $date2: The second DateTime object.
- $absolute (optional): If set to true, the interval will always be positive. Default is false.
2. Creating DateTime Objects
Before using date_diff(), you need to create DateTime objects representing the dates you want to compare. You can create DateTime objects using the DateTime class or its child classes such as DateTimeImmutable.
Here’s how to create DateTime objects for two dates:
php $date1 = new DateTime('2023-09-13'); $date2 = new DateTime('2023-10-15');
Now that we have our DateTime objects, let’s explore various practical scenarios where date_diff() can be incredibly useful.
3. Calculating the Difference in Days
One common use case is finding the difference between two dates in terms of days. This is particularly useful for applications like calculating the number of days until an event or determining the age of a user based on their birthdate.
php $date1 = new DateTime('1990-05-20'); $date2 = new DateTime('2023-09-13'); $interval = date_diff($date1, $date2); $daysDifference = $interval->days; echo "The difference in days is: $daysDifference days";
In this example, $daysDifference will contain the number of days between May 20, 1990, and September 13, 2023.
4. Working with Hours, Minutes, and Seconds
While finding the difference in days is common, you may also need to calculate the difference in hours, minutes, and seconds. This is crucial for applications involving time-sensitive operations.
php $startDateTime = new DateTime('2023-09-13 10:00:00'); $endDateTime = new DateTime('2023-09-13 14:30:45'); $interval = date_diff($startDateTime, $endDateTime); $hours = $interval->h; $minutes = $interval->i; $seconds = $interval->s; echo "The difference is $hours hours, $minutes minutes, and $seconds seconds";
Here, we calculate the time difference between two DateTime objects, including hours, minutes, and seconds.
5. Dealing with Absolute Intervals
By default, date_diff() provides intervals with negative values if the second date is earlier than the first. However, sometimes you may need the absolute difference regardless of the order of the dates. You can achieve this by setting the $absolute parameter to true.
php $date1 = new DateTime('2023-09-13'); $date2 = new DateTime('2023-08-01'); $interval = date_diff($date1, $date2, true); $daysDifference = $interval->days; echo "The absolute difference in days is: $daysDifference days";
In this example, regardless of the order of $date1 and $date2, the absolute difference will always be positive.
6. Working with Different Date Formats
In real-world applications, date and time values often come in various formats. date_diff() can handle DateTime objects, but what if you have dates in different formats or strings? You can parse them into DateTime objects first using the DateTime::createFromFormat() method.
php $dateString1 = '2023-09-13'; $dateString2 = '15/10/2023'; $date1 = DateTime::createFromFormat('Y-m-d', $dateString1); $date2 = DateTime::createFromFormat('d/m/Y', $dateString2); $interval = date_diff($date1, $date2); $daysDifference = $interval->days; echo "The difference in days is: $daysDifference days";
In this example, we parse two different date formats into DateTime objects before calculating the difference.
7. Handling Time Zones
When dealing with date and time calculations, it’s crucial to consider time zones, especially in global applications. PHP allows you to set the time zone for DateTime objects using the DateTime::setTimezone() method.
php $dateString = '2023-09-13 15:00:00'; $timezone = new DateTimeZone('America/New_York'); $date = DateTime::createFromFormat('Y-m-d H:i:s', $dateString, $timezone); // Perform calculations with $date
By setting the time zone, you ensure that the DateTime object represents the correct time in the specified zone.
8. Practical Example: Countdown Timer
Let’s put our knowledge to practical use by creating a countdown timer for an upcoming event. Suppose we want to display the time remaining until New Year’s Eve. We can achieve this by calculating the difference between the current date and time and the target date (December 31, 2023, 23:59:59).
php $currentDateTime = new DateTime(); $targetDateTime = new DateTime('2023-12-31 23:59:59'); $interval = date_diff($currentDateTime, $targetDateTime); $days = $interval->days; $hours = $interval->h; $minutes = $interval->i; $seconds = $interval->s; echo "Time remaining until New Year's Eve: $days days, $hours hours, $minutes minutes, and $seconds seconds";
This code calculates and displays the time remaining until New Year’s Eve, counting down in days, hours, minutes, and seconds.
Conclusion
In this practical guide, we’ve explored the versatility of PHP’s date_diff() function for various date and time calculations. Understanding how to create DateTime objects, work with different date formats, handle time zones, and calculate differences in days, hours, minutes, and seconds empowers you to tackle a wide range of date and time-related challenges in your web development projects.
By leveraging the date_diff() function effectively, you can create dynamic features like countdown timers, age calculators, and event schedulers, enhancing the user experience and functionality of your PHP applications. As you continue to hone your PHP skills, this invaluable tool will undoubtedly become a staple in your development toolkit.
Table of Contents
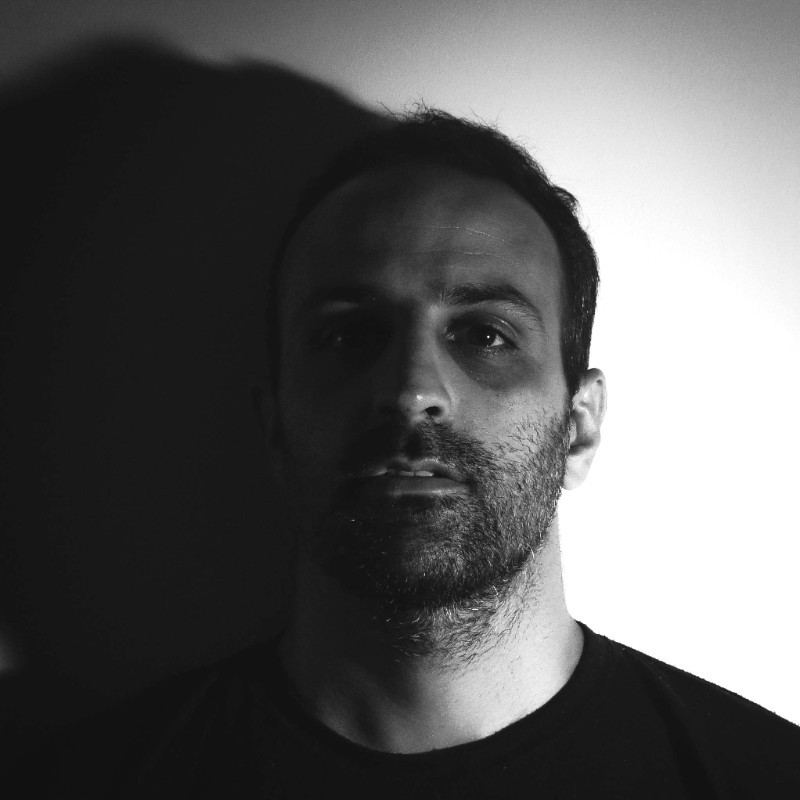
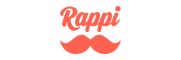