Understanding PHP’s die() and exit() Functions
In the realm of PHP programming, error handling and program termination are essential aspects to ensure smooth and efficient execution of code. Two functions that play a crucial role in achieving this are die() and exit(). These functions allow developers to gracefully exit a script or handle errors effectively. In this blog post, we will dive deep into the functionality of die() and exit(), examining their differences, use cases, and best practices.
1. The Purpose of die() and exit()
Both die() and exit() functions serve the same primary purpose: to terminate the execution of a PHP script. These functions are particularly useful when you want to halt the program flow due to certain conditions or errors. When invoked, they immediately stop the execution of the script and display a message if provided. While the core purpose is the same, there are subtle differences that set them apart.
2. die() Function
The die() function is a language construct in PHP that serves as a language construct to terminate the script. It takes an optional message as an argument, which is displayed as an error message when the script stops. Here’s the basic syntax:
php die(message); Example: php Copy code $age = 15; if ($age < 18) { die("You must be at least 18 years old to access this content."); } // Rest of the code if the condition is met
In this example, if the condition is met, the script will terminate, and the error message will be displayed. This can be particularly useful for preventing unauthorized access to certain parts of your application.
3. exit() Function
Similar to die(), the exit() function also terminates the script’s execution. It shares the same syntax and functionality but is primarily used to gracefully handle program termination. The optional message argument serves as a way to communicate the reason for termination. Here’s the syntax:
php exit(message);
Example:
php $file = "data.txt"; if (!file_exists($file)) { exit("The required file does not exist."); } // Rest of the code if the file exists
In this scenario, the script checks if a required file exists. If it doesn’t, the script will exit, displaying an informative message. This approach can help avoid further processing if necessary resources are missing.
4. Differences Between die() and exit()
While die() and exit() might seem interchangeable due to their similar purposes, there’s an interesting nuance that sets them apart. The primary distinction lies in their historical context within PHP development.
die() is a construct that originated in the earliest versions of PHP. On the other hand, exit() is a function that was introduced in later versions to provide a more consistent and conventional approach to program termination. In modern PHP development, both die() and exit() can be used interchangeably without any functional differences. However, using exit() is generally considered more in line with modern coding practices.
5. Error Handling and Best Practices
While die() and exit() can be valuable tools for halting script execution, they should be used with caution, particularly in larger applications. Abruptly terminating a script can lead to inconsistent states, incomplete data, and unexpected behaviors. Here are some best practices to keep in mind:
5.1. Use Conditional Statements
Before resorting to using die() or exit(), consider using conditional statements to handle errors and exceptional cases. This approach allows you to gracefully handle errors and provide informative error messages without immediately stopping the script.
5.2. Logging Errors
Instead of relying solely on error messages displayed to the user, implement a robust error logging system. This will help you track errors and issues more effectively, enabling you to identify and fix issues without the need for abrupt script termination.
5.3. Graceful Degradation
In situations where part of your application encounters an error, consider implementing graceful degradation. This means that even if an error occurs in one component, the rest of the application continues to function properly.
5.4. Exception Handling
For more complex applications, consider using PHP’s exception handling mechanisms. Exceptions allow you to handle errors in a structured manner, providing greater control over error messages and recovery strategies.
Conclusion
In the realm of PHP programming, the die() and exit() functions play a crucial role in controlling the flow of execution and handling errors. While their core purpose is the same—to terminate the script—subtle differences and historical context set them apart. When used judiciously and in accordance with best practices, these functions can be powerful tools for improving the reliability and robustness of your PHP applications.
Remember that modern coding practices emphasize graceful error handling and maintaining program integrity even in the face of unexpected issues. By understanding the nuances of die() and exit(), you can make informed decisions about when and how to use them effectively in your PHP projects.
Table of Contents
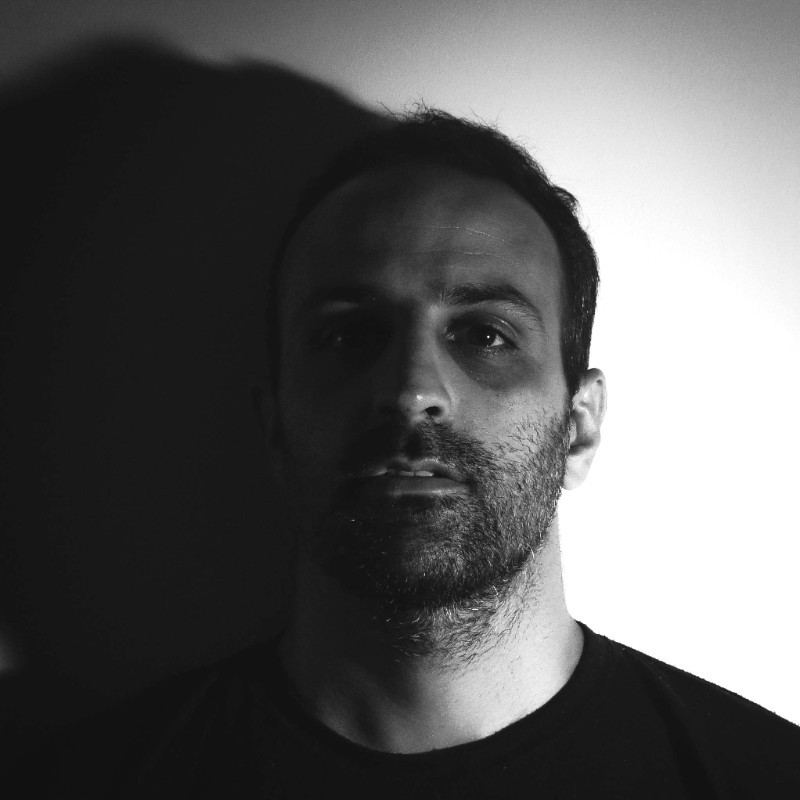
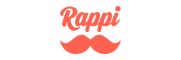