Building a PHP E-commerce Platform: A Step-by-Step Guide
In the digital age, e-commerce has become an integral part of the business landscape. Whether you’re a startup entrepreneur or an established business owner, having an online presence is crucial for reaching a wider audience and maximizing your sales potential. In this step-by-step guide, we will walk you through the process of building a PHP e-commerce platform from scratch. We’ll cover the fundamental concepts, architectural design, database management, and the integration of popular frameworks. By the end of this guide, you’ll have the knowledge and tools to create a powerful and customizable e-commerce platform that meets your specific business requirements.
Setting Up the Development Environment
To start building your PHP e-commerce platform, you need to set up a development environment. This typically involves installing PHP, a web server (such as Apache or Nginx), and a database management system (such as MySQL). You can use XAMPP or WAMP, which provide all-in-one packages that include these components. Once the environment is set up, you can create a new project directory and initialize a Git repository for version control.
Code Sample:
bash # Install XAMPP (example for Windows) Download XAMPP from https://www.apachefriends.org Run the installer and follow the instructions. # Initialize a new Git repository $ cd /path/to/project $ git init
Defining the Project Scope and Requirements
Before diving into the development process, it’s essential to define the project scope and requirements. Determine the target audience, the type of products you’ll be selling, and any specific features or functionalities you want to include. Create a list of user stories or use cases to better understand the interactions between the platform and its users. This step will help you plan and prioritize the development tasks effectively.
Code Sample:
plaintext User Story: As a customer, I want to be able to browse products by category. User Story: As a store owner, I want to manage product inventory and receive low stock alerts. User Story: As a customer, I want to add products to a shopping cart and proceed to checkout.
Designing the Database Structure
A well-designed database is crucial for storing and retrieving data efficiently. Determine the entities and relationships that will be part of your e-commerce platform, such as users, products, categories, orders, etc. Use a tool like MySQL Workbench or phpMyAdmin to create the database schema and define the necessary tables, columns, and relationships. Normalize the database structure to minimize data redundancy and improve performance.
Code Sample:
sql -- Create the users table CREATE TABLE users ( id INT PRIMARY KEY AUTO_INCREMENT, username VARCHAR(50) NOT NULL, email VARCHAR(100) NOT NULL, password VARCHAR(255) NOT NULL, role ENUM('customer', 'admin') NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ); -- Create the products table CREATE TABLE products ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(100) NOT NULL, description TEXT NOT NULL, price DECIMAL(10, 2) NOT NULL, stock INT NOT NULL, category_id INT, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP, FOREIGN KEY (category_id) REFERENCES categories(id) ON DELETE SET NULL ); -- Create the categories table CREATE TABLE categories ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(100) NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
Creating User Authentication and Authorization
Security is paramount for any e-commerce platform. Implementing user authentication and authorization ensures that only authorized users can access certain functionalities. Use PHP’s built-in session management or consider using a popular framework like Laravel or Symfony, which provide robust authentication and authorization mechanisms out of the box. Implement features such as user registration, login, password reset, and role-based access control.
Code Sample (Laravel):
php // User registration public function register(Request $request) { // Validate user input $validatedData = $request->validate([ 'name' => 'required|string|max:255', 'email' => 'required|string|email|unique:users|max:255', 'password' => 'required|string|min:8|confirmed', ]); // Create a new user $user = User::create([ 'name' => $validatedData['name'], 'email' => $validatedData['email'], 'password' => bcrypt($validatedData['password']), ]); // Log in the user Auth::login($user); // Redirect to the dashboard return redirect('/dashboard'); }
Building the Product Catalog
The product catalog is the heart of any e-commerce platform. Create a user-friendly interface for customers to browse and search for products. Develop features such as product listing, filtering by category or attributes, and pagination for large catalogs. Utilize frameworks like Laravel or CodeIgniter to handle database queries efficiently and manage the display of product details.
Code Sample (CodeIgniter):
php // Product listing public function index() { $data['products'] = $this->product_model->get_all_products(); $this->load->view('product_list', $data); } // Product details public function details($id) { $data['product'] = $this->product_model->get_product_by_id($id); $this->load->view('product_details', $data); }
Implementing Shopping Cart Functionality
To allow customers to add products to their carts, create a shopping cart system. Use session management or a dedicated library like Cartalyst’s Cart to store cart data. Implement features such as adding/removing items, updating quantities, and calculating the total price. Display the cart summary on multiple pages, and provide a clear checkout process for users to complete their purchases.
Code Sample:
php // Add item to cart public function addToCart(Request $request, $productId) { $product = Product::find($productId); if (!$product) { return redirect()->back()->with('error', 'Product not found.'); } Cart::add([ 'id' => $product->id, 'name' => $product->name, 'price' => $product->price, 'quantity' => $request->input('quantity', 1), ]); return redirect()->back()->with('success', 'Product added to cart.'); }
Integrating Payment Gateways
To process payments securely, integrate popular payment gateways like PayPal, Stripe, or Braintree. These gateways provide APIs or SDKs that handle payment transactions. Implement features such as selecting the preferred payment method, redirecting users to the payment gateway’s website, and handling payment status callbacks to update order statuses.
Code Sample (PayPal):
php // Create a PayPal payment public function createPayment(Request $request) { $apiContext = new \PayPal\Rest\ApiContext( new \PayPal\Auth\OAuthTokenCredential( 'YOUR_CLIENT_ID', // Replace with your PayPal Client ID 'YOUR_CLIENT_SECRET' // Replace with your PayPal Client Secret ) ); $payer = new \PayPal\Api\Payer(); $payer->setPaymentMethod('paypal'); $amount = new \PayPal\Api\Amount(); $amount->setTotal('10.00'); $amount->setCurrency('USD'); $transaction = new \PayPal\Api\Transaction(); $transaction->setAmount($amount); $redirectUrls = new \PayPal\Api\RedirectUrls(); $redirectUrls->setReturnUrl('http://example.com/success') ->setCancelUrl('http://example.com/cancel'); $payment = new \PayPal\Api\Payment(); $payment->setIntent('sale') ->setPayer($payer) ->setTransactions([$transaction]) ->setRedirectUrls($redirectUrls); try { $payment->create($apiContext); return redirect($payment->getApprovalLink()); } catch (\PayPal\Exception\PayPalConnectionException $e) { // Handle PayPal API connection errors } }
Managing Orders and Inventory
Implement a comprehensive order management system to handle the complete lifecycle of orders. Create tables to store order details, customer information, and order statuses. Develop features such as order creation, tracking, cancellation, and email notifications. For inventory management, update the product stock levels upon order placement and implement low stock notifications for the store owner.
Code Sample:
php // Create an order public function createOrder(Request $request) { $user = Auth::user(); $cartItems = Cart::getContent(); $order = new Order(); $order->user_id = $user->id; $order->status = 'pending'; $order->save(); foreach ($cartItems as $item) { $orderItem = new OrderItem(); $orderItem->order_id = $order->id; $orderItem->product_id = $item->id; $orderItem->quantity = $item->quantity; $orderItem->save(); // Update product stock $product = Product::find($item->id); $product->stock -= $item->quantity; $product->save(); } // Clear the cart Cart::clear(); // Send order confirmation email Mail::to($user->email)->send(new OrderConfirmationMail($order)); return redirect('/orders')->with('success', 'Order placed successfully.'); }
Enhancing Security and Performance
Security and performance are crucial for any e-commerce platform. Implement measures such as input validation, secure password storage, and protection against common vulnerabilities (e.g., SQL injection, cross-site scripting). Optimize database queries, utilize caching mechanisms, and implement techniques like lazy loading to enhance performance. Regularly update and patch dependencies to mitigate security risks.
Customizing and Extending the Platform
Every business has unique requirements, so it’s essential to provide customization options. Build an intuitive administration panel where the store owner can customize the platform’s appearance, configure settings, and manage products, categories, and orders. Develop extension mechanisms (e.g., plugins, modules) to allow third-party developers to add additional features to the platform.
Deploying the E-commerce Platform
When your e-commerce platform is ready, it’s time to deploy it to a production environment. Choose a reliable web hosting provider or set up your own server infrastructure. Ensure proper configuration of the web server, PHP settings, and database connection. Use HTTPS encryption for secure communication and regularly back up your data to prevent any potential data loss.
Conclusion
Building a PHP e-commerce platform from scratch may seem like a complex task, but by following this step-by-step guide, you’ve gained valuable insights into the process. Remember to prioritize security, performance, and user experience throughout the development journey. By leveraging PHP, MySQL, and popular frameworks, you can create a robust and customizable platform that meets your specific business needs. Good luck with your e-commerce venture!
Table of Contents
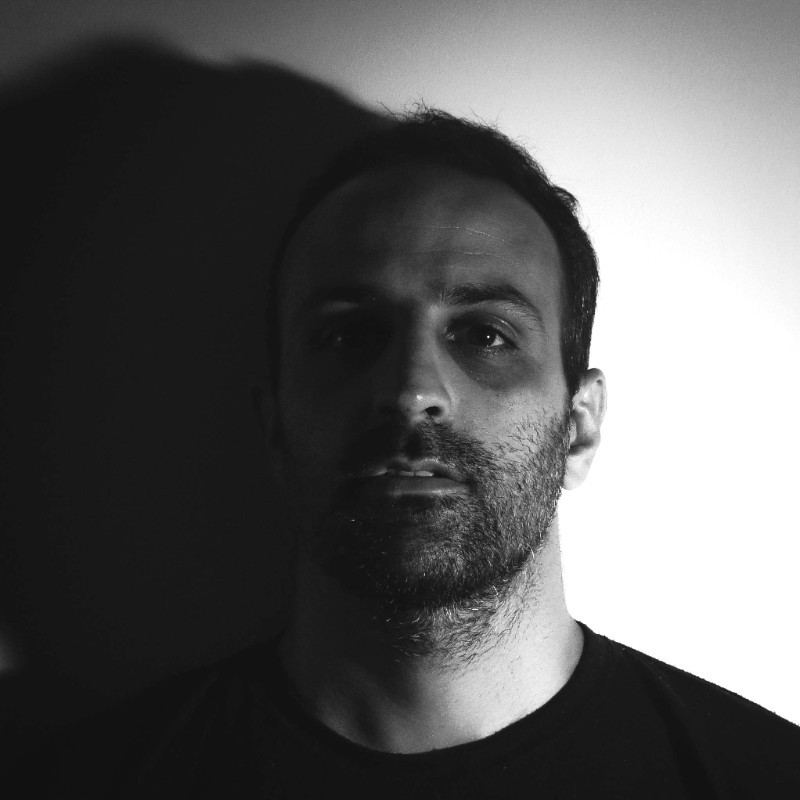
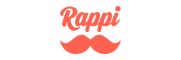