PHP Error Handling: Effective Strategies
In the world of web development, errors are inevitable. From syntax mistakes to unforeseen runtime issues, PHP developers often encounter errors during the development and maintenance of their applications. Effective error handling is crucial to ensure that these errors are caught, logged, and appropriately managed, leading to more robust and reliable PHP applications.
In this blog post, we will explore various strategies for handling errors in PHP. We will discuss best practices, guidelines, and code samples to help you implement an error handling mechanism that meets the specific needs of your PHP projects.
Understanding PHP Errors
Before diving into error handling strategies, it is crucial to understand the different types of errors that can occur in PHP. PHP errors can be broadly categorized into three main types:
- a) Notices: These are non-critical errors that do not affect the execution of the script but provide useful information about potential issues or inefficiencies in the code.
Example:
php // Notice: Undefined variable: name echo $name;
- b) Warnings: Warnings indicate potential issues that may cause problems in the script execution but allow the code to continue running.
Example:
php // Warning: Division by zero $result = 10 / 0;
- c) Fatal Errors: These are critical errors that prevent the script from executing further. Fatal errors typically occur due to severe issues like syntax errors or missing files.
Example:
php // Fatal error: Call to undefined function foo() foo();
Error Reporting Levels
PHP provides error_reporting, a configuration directive that controls the level of error reporting. By setting the error_reporting level, you can define which types of errors should be displayed or logged.
To set the error reporting level, you can use the error_reporting() function or modify the error_reporting directive in the php.ini file.
Example:
php // Display all errors except notices error_reporting(E_ALL & ~E_NOTICE);
The try-catch Block
One of the essential error handling techniques in PHP is the use of try-catch blocks. The try block contains the code that might throw an exception, while the catch block handles the exception if one occurs.
Example:
php try { // Code that might throw an exception // ... } catch (Exception $e) { // Handle the exception // ... }
Logging Errors
Logging errors is crucial for debugging and monitoring the behavior of your PHP applications. By logging errors, you can keep track of issues that occur during the execution of your code and gain insights into the root causes of failures.
Example:
php // Log errors to a file ini_set('error_log', '/path/to/error.log');
Graceful Error Messages
When an error occurs in a PHP application, it is essential to present users with informative and user-friendly error messages. Graceful error messages help users understand the issue and provide them with guidance on how to proceed.
Example:
php // Display a custom error message echo "An error occurred. Please try again later.";
Custom Error Handlers
PHP allows you to define custom error handlers to override the default error handling behavior. By implementing custom error handlers, you gain control over how errors are displayed, logged, or processed.
Example:
php // Custom error handler function function customErrorHandler($errno, $errstr, $errfile, $errline) { // Handle the error } // Set the custom error handler set_error_handler("customErrorHandler");
Exception Handling and Exception Types
Exceptions provide a structured way to handle errors and exceptional situations in PHP. By using the built-in Exception class or creating custom exception classes, you can catch and handle specific types of exceptions, allowing for more granular error handling.
Example:
php try { // Code that might throw an exception // ... } catch (InvalidArgumentException $e) { // Handle invalid argument exceptions // ... } catch (Exception $e) { // Handle other exceptions // ... }
Dealing with Fatal Errors
Fatal errors are challenging to handle because they halt the script execution abruptly. However, PHP provides a way to catch fatal errors using the register_shutdown_function() function. By registering a shutdown function, you can handle fatal errors gracefully.
Example:
php // Shutdown function to handle fatal errors function handleFatalError() { $error = error_get_last(); if ($error !== null && $error['type'] === E_ERROR) { // Handle the fatal error } } // Register the shutdown function register_shutdown_function('handleFatalError');
Debugging Techniques
Effective error handling goes hand in hand with robust debugging techniques. PHP provides various debugging tools and techniques to help you identify and fix errors in your code. Techniques such as using var_dump(), debug_backtrace(), and error_log() can be immensely helpful during the debugging process.
Example:
php // Dump variable information for debugging var_dump($variable); // Print a backtrace for debugging debug_backtrace(); // Log a message for debugging error_log("Debug message");
Testing Error Handling
To ensure that your error handling mechanism works as expected, it is crucial to thoroughly test it. Write unit tests and test scenarios that cover various error conditions to verify that your code handles errors appropriately.
Example:
php // Test for expected exceptions $this->expectException(Exception::class); // Test for error handling behavior // ...
Best Practices for Error Handling
When it comes to error handling in PHP, following best practices can help you build more robust and maintainable applications. Some key best practices include:
- Enable error reporting during development and testing.
- Log errors to a file or a centralized logging system.
- Use consistent and informative error messages.
- Implement granular exception handling.
- Separate error handling from the core application logic.
- Test error handling thoroughly.
Conclusion
Effective error handling is a critical aspect of PHP application development. By understanding the different types of errors, setting appropriate error reporting levels, and implementing best practices, you can build robust and reliable PHP applications.
In this blog post, we explored various strategies for handling errors in PHP, including the use of try-catch blocks, logging errors, creating custom error handlers, and dealing with fatal errors. We also discussed debugging techniques and the importance of testing error handling.
By implementing these strategies and adhering to best practices, you can enhance the stability, maintainability, and user experience of your PHP applications. Remember, error handling is not an afterthought but an integral part of the development process that contributes to the overall quality of your codebase.
Table of Contents
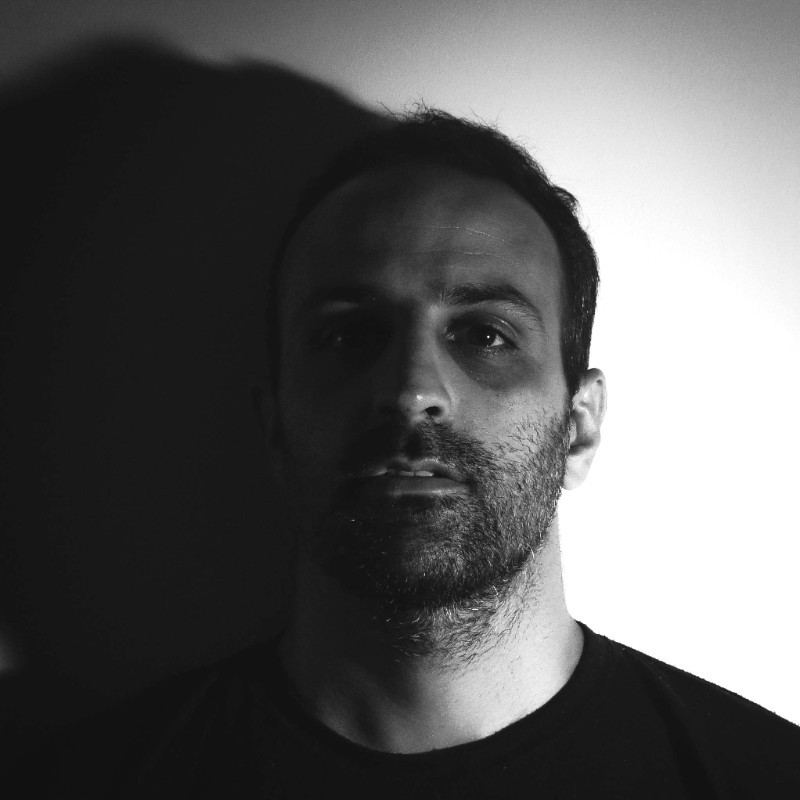
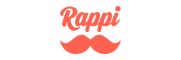