How to work with exceptions?
Working with exceptions in PHP is essential for handling and gracefully recovering from errors and unexpected situations in your code. Exceptions allow you to catch and handle errors without causing the script to terminate abruptly. Here’s how to work with exceptions in PHP:
- Throwing Exceptions:
– To signal an error or exceptional condition in your code, you can throw an exception using the `throw` statement. Exceptions should be instances of classes that extend the built-in `Exception` class or its subclasses.
```php if ($errorCondition) { throw new CustomException("An error occurred."); } ```
- Catching Exceptions:
– To catch exceptions and handle errors, use the `try` and `catch` blocks. Wrap the code that may throw exceptions inside a `try` block, and specify the type of exception to catch in the `catch` block.
```php try { // Code that may throw an exception } catch (CustomException $e) { // Handle the exception echo "Caught exception: " . $e->getMessage(); } ```
- Handling Multiple Exceptions:
– You can handle multiple exceptions by adding multiple `catch` blocks, each for a specific exception type, in descending order of specificity.
```php try { // Code that may throw exceptions } catch (CustomException $e) { // Handle CustomException } catch (AnotherException $e) { // Handle AnotherException } catch (Exception $e) { // Handle other exceptions } ```
- The `finally` Block:
– You can use a `finally` block to specify code that should run regardless of whether an exception was thrown or caught. This block is useful for cleanup tasks.
```php try { // Code that may throw exceptions } catch (CustomException $e) { // Handle CustomException } finally { // Cleanup code } ```
- Creating Custom Exceptions:
– You can create custom exception classes by extending the `Exception` class or its subclasses. Custom exceptions allow you to provide more context and specific error messages.
```php class CustomException extends Exception { // Custom properties and methods } ```
Exception handling in PHP provides a structured way to manage errors and unexpected conditions, making your code more robust and maintainable. It allows you to gracefully handle issues and provide meaningful error messages to users while ensuring that your scripts continue to execute without abrupt termination.
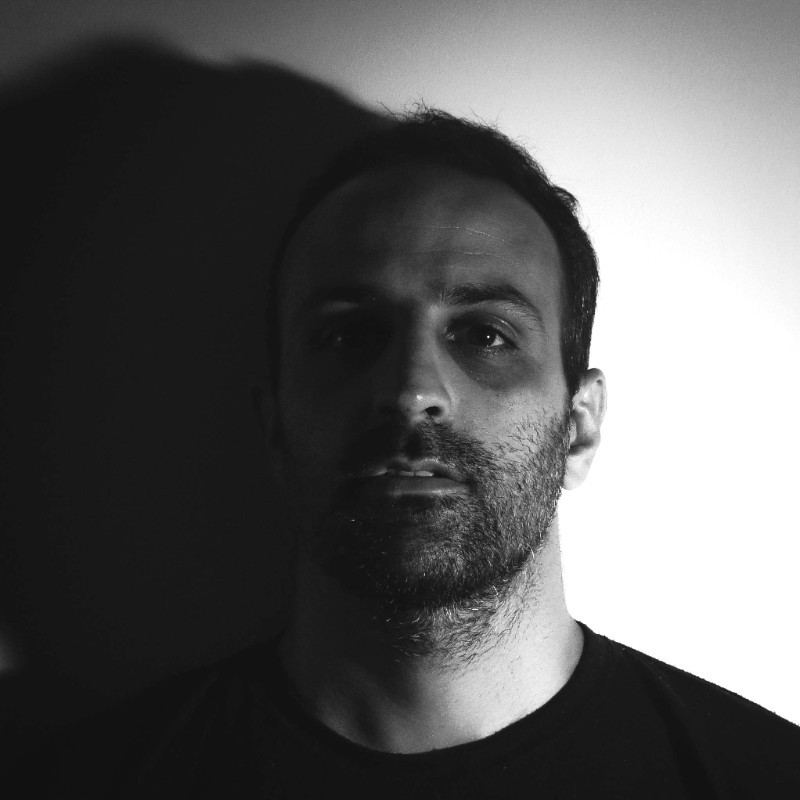
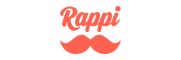