Mastering PHP’s explode() and implode() Functions
In the world of web development, dealing with strings is an everyday task. Whether you’re working on data parsing, form submissions, or database manipulation, understanding how to manipulate strings efficiently is crucial. PHP, being one of the most popular server-side scripting languages, provides a set of powerful string manipulation functions, among which explode() and implode() stand out. These functions allow you to split and join strings with ease, opening up a world of possibilities for data processing and presentation. In this guide, we’ll delve deep into these functions, explore their usage, and provide you with practical examples to master their capabilities.
1. Introduction to explode() and implode()
Before we dive into the specifics of these functions, let’s establish a clear understanding of what they do. explode() and implode() are PHP functions used for splitting and joining strings, respectively.
- explode(): This function splits a string into an array of substrings based on a specified delimiter. It’s incredibly useful when you have a single string containing multiple values that you want to extract and work with separately.
- implode(): Also known as join(), this function takes an array of strings and concatenates them into a single string using a glue (separator) between each element. It’s perfect for combining multiple values into a formatted string.
2. Understanding the explode() Function
2.1. Basic Syntax
The basic syntax of the explode() function is as follows:
php array explode(string $delimiter, string $string, int $limit = PHP_INT_MAX)
- $delimiter: The character or sequence that will be used to split the string.
- $string: The input string to be split.
- $limit: (Optional) The maximum number of elements in the resulting array. If specified, the array will contain a maximum of $limit elements, with the last element containing the remainder of the string.
2.2. Parameters and Usage
Let’s break down the parameters and understand their usage:
- $delimiter: This is the most crucial parameter as it determines where the string will be split. For example, if you’re dealing with a comma-separated list of values, the comma would be your delimiter.
- $string: This parameter holds the input string that you want to split into an array.
- $limit: While optional, this parameter allows you to control the maximum number of elements in the resulting array. It can be useful when you’re interested in only a specific number of elements from the string.
Examples: Splitting Comma-Separated Values
Let’s say you have a string containing the names of fruits separated by commas: “apple,banana,orange,grape”. You can use the explode() function to split this string into an array of fruit names like this:
php $fruitsString = "apple,banana,orange,grape"; $fruitsArray = explode(",", $fruitsString); // $fruitsArray will now contain: ["apple", "banana", "orange", "grape"]
2.3. Handling Different Delimiters
Delimiters can vary depending on the context. If you’re dealing with a list of tags separated by spaces, you can adjust the delimiter accordingly:
php $tagsString = "php programming web development"; $tagsArray = explode(" ", $tagsString); // $tagsArray will contain: ["php", "programming", "web", "development"]
3. Deep Dive into the implode() Function
3.1. Basic Syntax
The basic syntax of the implode() function is as follows:
php string implode(string $glue, array $pieces)
- $glue: The string used to concatenate the array elements.
- $pieces: The array of strings that you want to join together.
3.2. Parameters and Application
Let’s understand the parameters and how they are used:
- $glue: This parameter holds the string that will be used to join the array elements. It’s the glue that sticks the pieces together.
- $pieces: This array parameter contains the elements you want to join together into a single string.
Examples: Joining Array Elements into a String
Suppose you have an array of technology terms that you want to combine into a sentence:
php $techTermsArray = ["HTML", "CSS", "JavaScript", "PHP"]; $techSentence = implode(", ", $techTermsArray); // $techSentence will be: "HTML, CSS, JavaScript, PHP"
3.3. Customizing Glue for Joining
The beauty of the implode() function lies in its flexibility. You can customize the glue to suit your formatting needs:
php $numbersArray = [1, 2, 3, 4, 5]; $numbersString = implode(" - ", $numbersArray); // $numbersString will be: "1 - 2 - 3 - 4 - 5"
4. Practical Examples of Using explode() and implode()
4.1. Creating Tags from a String
Let’s say you’re building a content management system and users enter tags for their articles in a single input field, separated by commas. You can use explode() to turn this input into an array of tags:
php $userTagsInput = "php, programming, web development"; $tagsArray = explode(", ", $userTagsInput); // $tagsArray will contain: ["php", "programming", "web development"]
4.2. Reversing the Process: Converting Tags Back to a String
When you retrieve the tags from the database, you might want to display them in the original format. Here’s where implode() comes to the rescue:
php $tagsArray = ["php", "programming", "web development"]; $tagsString = implode(", ", $tagsArray); // $tagsString will be: "php, programming, web development"
4.3. Managing User Input from Forms
In a user registration form, you might allow users to select multiple interests. When processing the form data, you can split the selected interests into an array using explode():
php $userInterestsInput = "coding, reading, hiking"; $interestsArray = explode(", ", $userInterestsInput); // $interestsArray will contain: ["coding", "reading", "hiking"]
Later, when you want to save these interests in the database as a single string, you can use implode():
php $interestsArray = ["coding", "reading", "hiking"]; $interestsString = implode(", ", $interestsArray); // $interestsString will be: "coding, reading, hiking"
5. Best Practices for Using explode() and implode()
5.1. Data Sanitization and Validation
When dealing with user input, always sanitize and validate the data before using explode() or implode(). This prevents potential security vulnerabilities.
5.2. Error Handling
Both functions can encounter errors, such as passing incorrect parameters or dealing with unexpected data formats. Always implement error handling to gracefully manage such scenarios.
6. Performance Considerations
While explode() and implode() are efficient for most use cases, keep in mind that excessively large strings or arrays can impact performance. Benchmark and test your code when dealing with significant data.
7. Alternatives to Consider
For more complex string manipulation, consider using regular expressions (preg_split()) alongside explode() and implode(). Additionally, PHP’s strtok() function can also be a suitable alternative in certain scenarios.
Conclusion
In this guide, we’ve journeyed through the powerful world of PHP’s explode() and implode() functions. These two functions, while seemingly simple, have the potential to greatly enhance your string manipulation capabilities. From splitting strings into arrays to joining arrays into strings, you now have the tools to tackle various data processing challenges. Remember to implement best practices, such as data validation and error handling, to ensure your code is secure and reliable. With this newfound knowledge, you’re well-equipped to wield the might of explode() and implode() in your web development projects. Happy coding!
Table of Contents
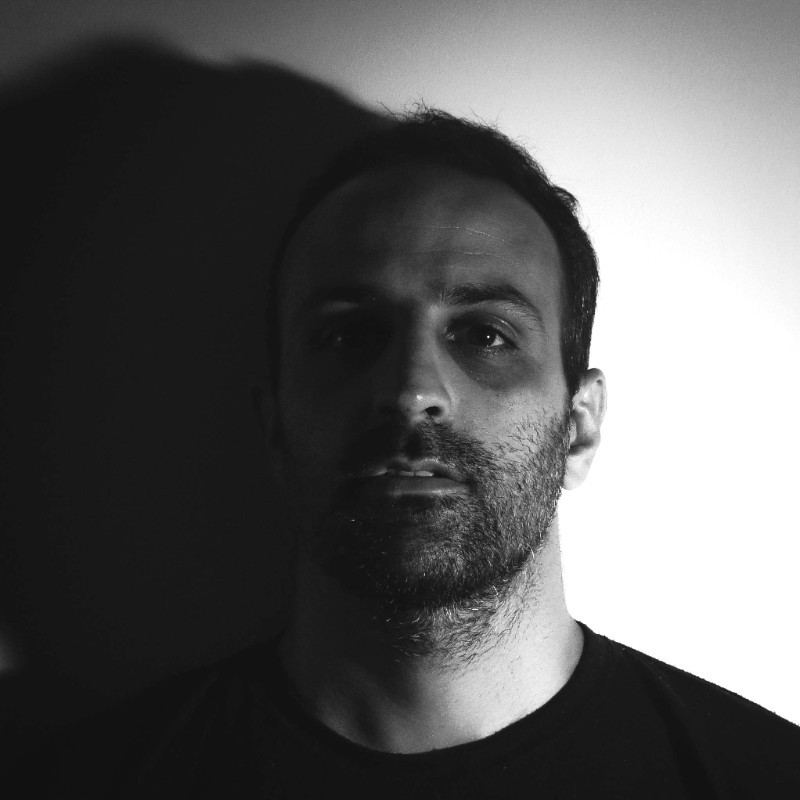
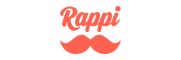