Unpacking PHP’s explode() Function: A Complete Guide
PHP, one of the most popular server-side scripting languages, offers a wide array of functions to manipulate strings. Among these functions, explode() stands out as a versatile tool for breaking down strings into smaller components. Whether you’re parsing user input or handling data from external sources, understanding how to use explode() effectively is essential for efficient string manipulation in PHP.
Table of Contents
In this comprehensive guide, we will dive deep into the explode() function, exploring its syntax, use cases, examples, and best practices. By the end, you’ll have a solid grasp of how to harness the power of explode() to simplify your PHP programming tasks.
1. Understanding explode()
At its core, the explode() function in PHP is used to split a string into an array of substrings based on a specified delimiter. This can be immensely useful when dealing with text data, as it allows you to break down a string into manageable parts for further processing.
2. Syntax of explode()
Before we delve into practical examples, let’s take a look at the syntax of explode():
php explode(string $delimiter, string $string, int $limit = PHP_INT_MAX): array
Here’s what each parameter means:
- $delimiter: The string used to split the input $string.
- $string: The input string that you want to split.
- $limit (optional): An integer that specifies the maximum number of elements in the resulting array. If provided, explode() will return at most $limit elements, and the last element will contain the rest of the string.
3. Basic Usage
To get started, let’s look at a simple example of how to use explode():
php $input = "apple,banana,cherry"; $fruits = explode(",", $input); print_r($fruits);
In this example, we have a comma-separated list of fruits in the $input variable. We use explode(“,”) to split the string into an array of fruits. When we print the resulting array using print_r(), the output will be:
csharp Array ( [0] => apple [1] => banana [2] => cherry )
As you can see, explode() has successfully split the string into an array, with each element corresponding to a fruit.
4. Delimiter: Your Splitting Companion
The delimiter is a crucial element when using explode(). It determines how the input string should be divided. Let’s explore different scenarios involving delimiters.
4.1. Using Single Characters as Delimiters
In most cases, you’ll use single characters as delimiters. Here’s an example:
php $input = "one,two,three,four,five"; $numbers = explode(",", $input); print_r($numbers);
The output will be:
csharp Array ( [0] => one [1] => two [2] => three [3] => four [4] => five )
In this case, the comma (,) serves as the delimiter, splitting the string into an array of numbers.
4.2. Using Multiple Characters as Delimiters
Sometimes, you may need to use multiple characters as delimiters. For instance, if you’re working with a text document where paragraphs are separated by two newlines (\n\n), you can use that as the delimiter:
php $text = "This is the first paragraph.\n\nThis is the second paragraph."; $paragraphs = explode("\n\n", $text); print_r($paragraphs);
The output will be:
csharp Array ( [0] => This is the first paragraph. [1] => This is the second paragraph. )
By specifying \n\n as the delimiter, we successfully split the text into paragraphs.
5. Limiting Exploded Pieces
In some cases, you might not need to split the entire string. You can limit the number of pieces you get from explode() by using the optional $limit parameter.
Here’s an example:
php $input = "apple,banana,cherry,dates"; $fruits = explode(",", $input, 2); print_r($fruits);
With a limit of 2, the output will be:
csharp Array ( [0] => apple [1] => banana,cherry,dates )
As you can see, the resulting array contains only two elements, and the rest of the string remains intact in the second element.
6. Handling Empty Elements
By default, explode() will include empty elements in the resulting array if the delimiter appears consecutively in the input string. For example:
php $input = "apple,,banana,,cherry"; $fruits = explode(",", $input); print_r($fruits);
The output will be:
csharp Array ( [0] => apple [1] => [2] => banana [3] => [4] => cherry )
To handle empty elements, you can use the array_filter() function to remove them from the array:
php $fruits = array_filter(explode(",", $input)); print_r($fruits);
Now, the output will be:
csharp Array ( [0] => apple [2] => banana [4] => cherry )
By applying array_filter(), we’ve removed the empty elements, resulting in a cleaner array.
7. Real-world Use Cases
Now that we’ve covered the basics, let’s explore some real-world use cases where the explode() function can be a lifesaver.
7.1. Splitting User Input
Imagine you have a form where users enter their hobbies separated by commas. To process this input, you can use explode() to obtain an array of hobbies:
php $userInput = "Reading,Swimming,Coding,Painting"; $hobbies = explode(",", $userInput); foreach ($hobbies as $hobby) { echo "I love $hobby!<br>"; }
This code will display:
css I love Reading! I love Swimming! I love Coding! I love Painting!
7.2. Parsing CSV Data
When dealing with CSV (Comma-Separated Values) files, explode() can be incredibly useful for parsing data. Let’s say you have a CSV file containing student information:
php $csvData = "John,Doe,25\nJane,Smith,23\nBob,Johnson,28"; $students = explode("\n", $csvData); foreach ($students as $student) { $data = explode(",", $student); echo "Name: {$data[0]} {$data[1]}, Age: {$data[2]}<br>"; }
This code will output:
yaml Name: John Doe, Age: 25 Name: Jane Smith, Age: 23 Name: Bob Johnson, Age: 28
By using explode(), we’ve effectively parsed the CSV data into a structured format.
7.3. Extracting URLs from Text
Suppose you have a block of text containing multiple URLs, and you want to extract and display them separately. Here’s how you can do it:
php $text = "Check out our website at https://www.example.com. For more information, visit https://blog.example.com."; $urls = explode(" ", $text); foreach ($urls as $url) { if (strpos($url, "http") === 0) { echo "Link: $url<br>"; } }
This code will output:
arduino Link: https://www.example.com. Link: https://blog.example.com.
By splitting the text using spaces as delimiters and checking for the presence of “http,” we’ve successfully extracted and displayed the URLs.
8. Best Practices
As you incorporate explode() into your PHP projects, consider the following best practices:
- Error Handling: Always check the return value of explode(). If the delimiter is not found in the input string, it will return false. Handle this case gracefully to avoid unexpected errors in your code.
- Sanitization: If you’re using user input as the input string, be cautious about potential security risks. Sanitize the input to prevent malicious input that could disrupt your application.
- Efficiency: For large strings or when processing many strings in a loop, be mindful of performance. In such cases, consider alternative string manipulation functions like strpos() and substr() for better efficiency.
- Documentation: Comment your code to make it more readable and understandable for yourself and other developers. Explain the purpose of the explode() function and how it fits into your code.
Conclusion
In this comprehensive guide, we’ve unpacked PHP’s explode() function, covering its syntax, usage, delimiters, limiting options, and real-world applications. Armed with this knowledge, you now have a powerful tool at your disposal for manipulating strings efficiently in PHP.
Remember that explode() is just one of the many string manipulation functions PHP offers. As you gain experience, you’ll discover when it’s the best fit for your projects and when other functions may be more suitable. String manipulation is a fundamental skill in PHP development, and mastering functions like explode() will make you a more proficient and versatile developer.
So go ahead, experiment with explode(), explore its capabilities, and elevate your PHP programming skills to new heights. Happy coding!
Table of Contents
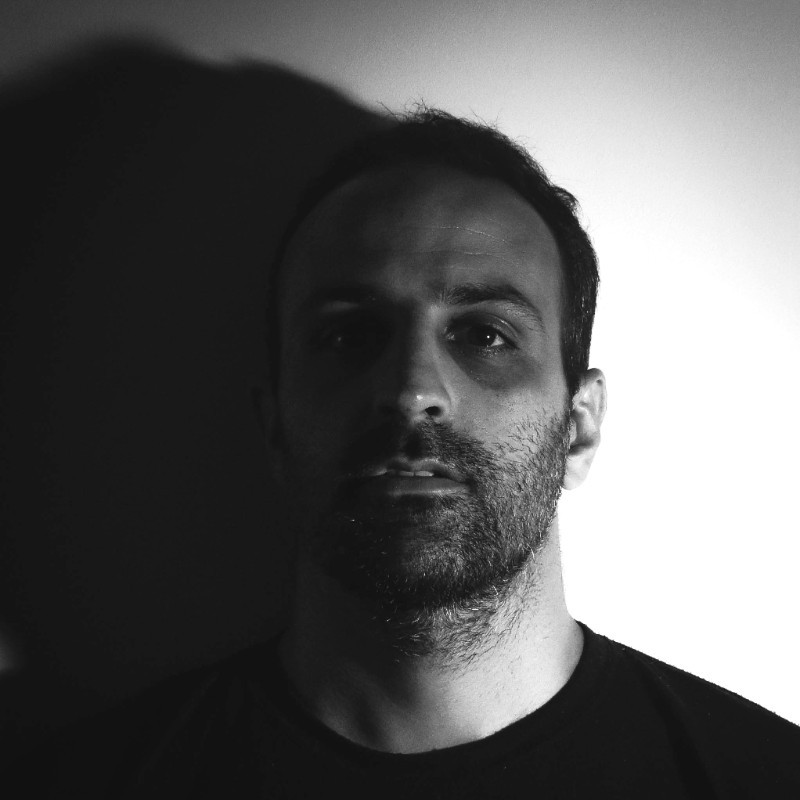
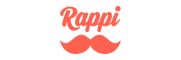