PHP and XML: Building a Feed Reader
In today’s fast-paced digital world, staying updated with the latest content from your favorite websites, blogs, and news sources is essential. Traditional methods of visiting each website individually to check for updates can be time-consuming and inefficient. That’s where a feed reader comes in handy. A feed reader allows you to consolidate content from various sources into one centralized location, saving you time and effort. In this tutorial, we’ll delve into the process of building a dynamic feed reader using the versatile combination of PHP and XML.
1. Introduction to Feed Readers and their Importance
Feed readers, also known as RSS readers or news aggregators, are tools that help you stay updated with content from multiple websites in one place. They collect and display the latest articles, blog posts, news, and other content from websites you follow, eliminating the need to manually visit each site. Building a personalized feed reader empowers you to control the content you consume, ensuring you never miss out on updates.
2. Understanding XML as a Data Format
XML (eXtensible Markup Language) is a versatile data format that’s widely used for storing and transporting structured data. It’s particularly useful for feed readers because it allows information to be organized hierarchically with meaningful tags. RSS (Really Simple Syndication) and Atom feeds, common formats for delivering content, are based on XML. In our project, XML will serve as the foundation for storing and distributing the feed data.
3. Getting Started with PHP for Feed Reading
Before we dive into the coding process, let’s set up our development environment and ensure we have PHP installed.
- Setting Up the Development Environment: To get started, you’ll need a text editor for writing your code. Popular choices include Visual Studio Code, Sublime Text, and Atom. Additionally, you’ll need a local server environment to execute PHP scripts. You can use solutions like XAMPP or WAMP for this purpose.
- Installing PHP: If PHP is not already installed on your system, you can download it from the official PHP website. Follow the installation instructions for your specific operating system.
4. Parsing XML Feeds with PHP
In this section, we’ll explore how to parse XML feeds using PHP. We’ll utilize the SimpleXML extension, which provides a convenient way to work with XML data.
- SimpleXML: A Powerful Tool for XML Parsing: SimpleXML is a PHP extension that makes it easy to work with XML documents by converting them into objects that can be manipulated like any other PHP objects.
- Loading and Accessing XML Data: To begin, you’ll load an XML feed using the simplexml_load_file() function. Once loaded, you can traverse the XML structure and access its elements using object-oriented syntax.
php $xml = simplexml_load_file('feed.xml'); $title = $xml->channel->title; $items = $xml->channel->item;
5. Designing the Feed Reader Interface
A user-friendly interface is crucial for any application, and our feed reader is no exception. Let’s start by setting up the HTML and CSS for our reader’s interface.
- HTML and CSS Setup: Create an HTML file and link it to a CSS stylesheet. This will provide the structure and styling for your feed reader.
- Creating the User Interface: Design the layout of your feed reader. You might want to include a header, a navigation menu for different feed categories, and a section to display the feed items.
6. Fetching and Displaying Feeds
Now comes the exciting part: fetching and displaying feeds in your reader’s interface.
- Retrieving XML Feeds: Create a PHP script that retrieves XML feeds from various sources. You can use the file_get_contents() function to fetch the feed’s content.
- Extracting Relevant Data: Once you have the XML content, parse it using SimpleXML as discussed earlier. Extract relevant data such as article titles, descriptions, and publication dates.
- Displaying Feed Items: Populate your reader’s interface with the extracted feed items. You can use loops to iterate through the items and display them in a user-friendly format.
7. Adding Interactivity and User Experience
To make your feed reader more engaging, add interactivity and user experience features.
- Making the Feed Reader Dynamic: Implement AJAX to enable dynamic loading of new feed items without having to refresh the entire page. This ensures a seamless experience for users.
- Implementing Refresh Functionality: Provide users with the option to manually refresh the feed list to get the latest content. This can be achieved through a “Refresh” button or an automatic refresh timer.
8. Final Touches and Future Enhancements
As you near completion, consider adding some final touches and planning for future improvements.
- Error Handling and User Feedback: Implement error handling to gracefully handle situations where the XML feed cannot be fetched or parsed. Provide users with meaningful error messages to enhance their experience.
- Adding Feed Categories: Extend your feed reader by incorporating the concept of categories. Allow users to organize their feeds into different categories based on their interests.
Conclusion
Congratulations! You’ve successfully built a functional feed reader using PHP and XML. This project showcases the power of PHP for data manipulation and XML for structuring content. By following this tutorial, you’ve gained insights into creating a dynamic, user-friendly feed reader that keeps you connected to the latest content from your favorite websites. As you continue to explore and enhance your feed reader, you’ll deepen your understanding of PHP and XML while providing yourself with a valuable tool for staying informed in the digital age.
Table of Contents
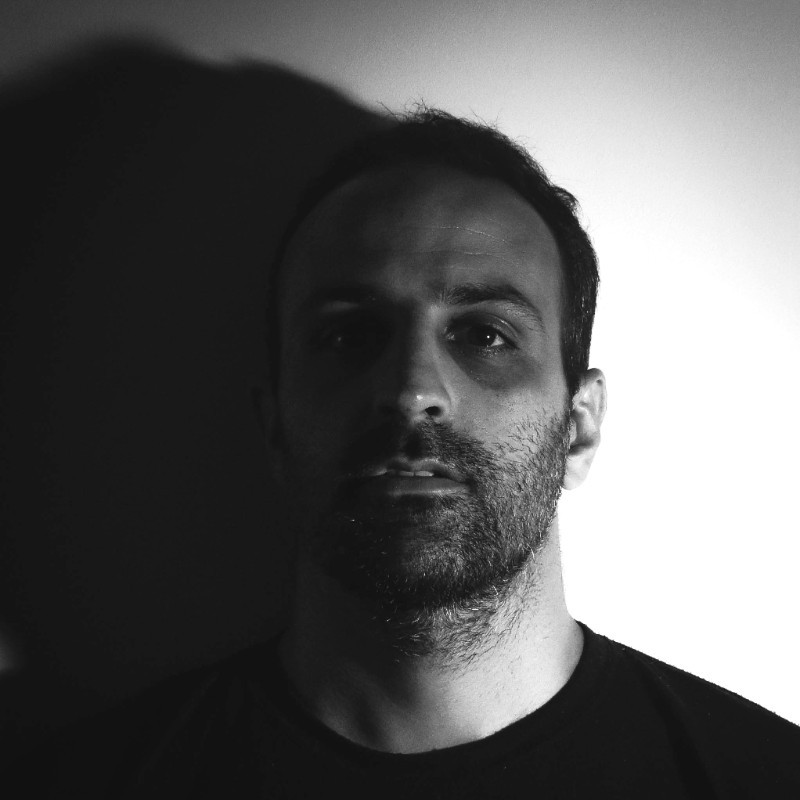
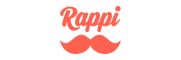