How to handle file downloads?
Handling file downloads in PHP is a common task for web applications, allowing users to retrieve files like images, documents, or media. Here’s a step-by-step guide on how to handle file downloads effectively:
- Server-Side Setup:
Begin by configuring your server to allow file downloads. Ensure that the files you want to serve are stored in a directory accessible to PHP, typically within your web root.
- Create a Download Link:
On your web page, create a link or button that initiates the download. Use the HTML `<a>` element with the `href` attribute pointing to a PHP script that handles the download. For example:
```html <a href="download.php?file=myfile.pdf">Download PDF</a> ```
- PHP Download Script:
Create a PHP script (e.g., `download.php`) that handles the file download. In this script, you’ll need to:
- Sanitize and validate the input, ensuring that the requested file is safe to access.
- Set appropriate HTTP headers to indicate that you’re sending a file for download. Common headers include `Content-Type`, `Content-Disposition`, and `Content-Length`.
- Open and read the file from the server and output it to the client using `readfile()` or similar functions.
Here’s a simplified example of a download script:
```php <?php $file = $_GET['file']; $filepath = 'path_to_your_files/' . $file; if (file_exists($filepath)) { header('Content-Type: application/octet-stream'); header('Content-Disposition: attachment; filename="' . $file . '"'); header('Content-Length: ' . filesize($filepath)); readfile($filepath); exit; } else { echo 'File not found.'; } ```
- Security Considerations:
Ensure that the download script is secure. Validate user input and sanitize file names to prevent directory traversal attacks or malicious file downloads.
- User Experience:
Enhance the user experience by providing informative messages, such as error messages for unavailable files or successful downloads.
By following these steps, you can effectively handle file downloads in PHP, making it easy for users to access files from your web application. Always prioritize security and validation to protect your server and users from potential threats.
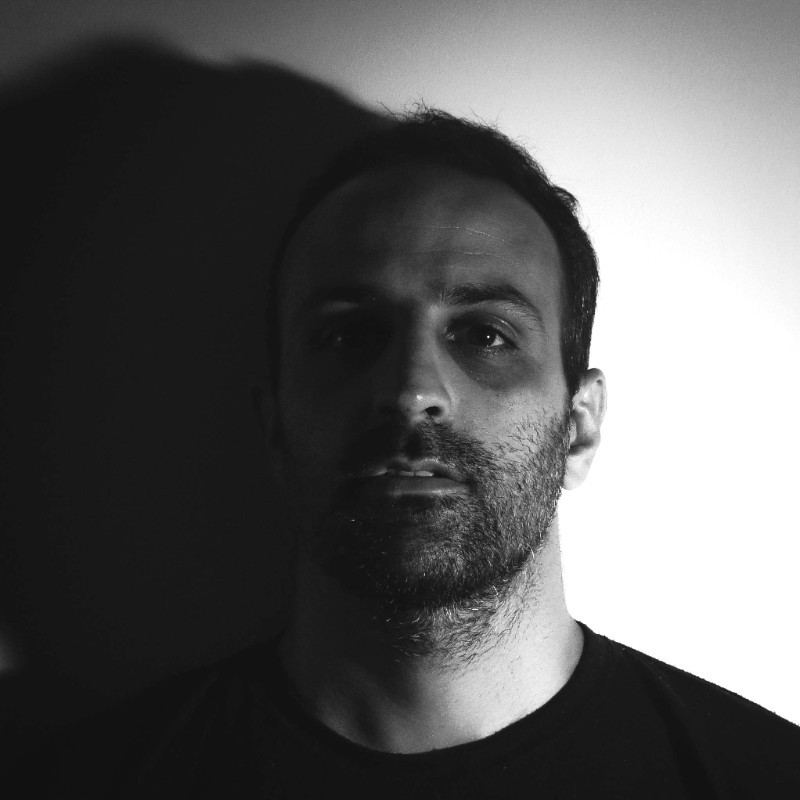
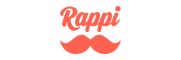