PHP’s file_exists() Function: A Comprehensive Look
PHP is a powerful server-side scripting language that allows developers to create dynamic and interactive web applications. One of the essential aspects of web development is working with files and directories. Whether it’s uploading user files, checking for the existence of specific files, or managing file systems, PHP provides a wide range of functions to handle these tasks.
Table of Contents
One such function is file_exists(). In this comprehensive guide, we will delve deep into PHP’s file_exists() function. We’ll explore its syntax, use cases, and best practices, accompanied by code samples and practical examples to help you harness its full potential.
1. Understanding the Basics
Before diving into the specifics of the file_exists() function, let’s first understand its fundamental purpose.
The file_exists() function in PHP is designed to check whether a file or directory exists at a specified path. It returns a boolean value, true if the file or directory exists and false otherwise. This simple yet powerful function plays a crucial role in various aspects of web development.
1.1. Syntax
Let’s start by looking at the syntax of the file_exists() function:
php file_exists($filename)
Here, $filename is the path to the file or directory you want to check. It can be an absolute or relative path.
2. Use Cases
2.1. Checking File Existence
The most common use case of file_exists() is checking whether a file exists before performing operations on it. For instance, before opening a file for reading, you might want to ensure that the file exists to prevent errors. Here’s a simple example:
php $filename = 'data.txt'; if (file_exists($filename)) { // File exists, perform operations $content = file_get_contents($filename); // ... } else { // File does not exist, handle the error echo 'File does not exist.'; }
In this example, the script checks if the file ‘data.txt’ exists using file_exists(). If it does, it proceeds with reading the file’s contents; otherwise, it displays an error message.
2.2. Validating User Uploads
When users upload files to your website, you should validate whether the uploaded file exists on the server before processing it further. The file_exists() function can help ensure that the uploaded file is accessible and ready for processing.
php if (isset($_FILES['user_upload'])) { $uploadFile = $_FILES['user_upload']['tmp_name']; if (file_exists($uploadFile)) { // Process the uploaded file // ... } else { // Handle the error echo 'Uploaded file does not exist.'; } }
In this example, we check if the uploaded file exists in the temporary directory before proceeding with any processing. This step is crucial for ensuring the security and integrity of user uploads.
2.3. Dynamic File Inclusion
PHP allows you to dynamically include files in your scripts. Before including a file, you can use file_exists() to make sure the file is available. This is particularly useful when working with templates, libraries, or configuration files.
php $configFile = 'config.php'; if (file_exists($configFile)) { include($configFile); // ... } else { // Handle the error echo 'Configuration file not found.'; }
By using file_exists() before including the ‘config.php’ file, you prevent potential issues caused by missing files.
2.4. Directory Existence
In addition to checking file existence, file_exists() can also be used to verify the existence of directories. This is helpful when you need to ensure that a directory is in place before creating, moving, or deleting files within it.
php $directory = 'uploads'; if (file_exists($directory)) { // Directory exists, perform operations // ... } else { // Directory does not exist, handle the error echo 'Directory does not exist.'; }
This code checks if the ‘uploads’ directory exists and then proceeds with further operations if it does. If the directory doesn’t exist, it provides an appropriate error message.
3. Handling Edge Cases
When working with the file_exists() function, there are some important considerations and potential challenges to keep in mind.
3.1. Absolute vs. Relative Paths
The path you provide to file_exists() can be either absolute or relative. An absolute path is the full path from the root directory, while a relative path is relative to the current working directory of your script.
It’s essential to be aware of the current working directory because it can affect the outcome of file_exists(). To ensure consistency, you can use absolute paths or use the __DIR__ constant to get the directory of the current script and build paths relative to that.
php $relativePath = 'images/image.jpg'; $absolutePath = __DIR__ . '/images/image.jpg'; if (file_exists($relativePath)) { // This may or may not work as expected depending on the current working directory. // ... } if (file_exists($absolutePath)) { // This will reliably check for the file's existence using an absolute path. // ... }
3.2. File Permissions
File permissions play a significant role in whether file_exists() returns true or false. Even if a file physically exists on the server, if the PHP script does not have the necessary permissions to access it, file_exists() will return false.
Before using file_exists(), ensure that your PHP script has the appropriate permissions to read the file or directory you’re checking.
3.3. Symbolic Links
When dealing with symbolic links, file_exists() behaves differently depending on the operating system and the PHP configuration. On some systems, it may return true if the symbolic link points to a valid target, while on others, it may return false. To check whether a symbolic link exists, you can use the is_link() function.
php $link = 'symlink-to-file.txt'; if (is_link($link)) { // It's a symbolic link // ... } else { // It's not a symbolic link or doesn't exist // ... }
4. Best Practices
To make the most out of file_exists() and ensure robust file and directory handling in your PHP applications, consider the following best practices:
4.1. Error Handling
Always include error handling when using file_exists(). If the function returns false, provide informative error messages or take appropriate actions to handle the absence of the file or directory gracefully.
4.2. Use Absolute Paths
Using absolute paths with file_exists() is generally more reliable than using relative paths, as it eliminates ambiguity regarding the current working directory.
4.3. Combine with Other Functions
file_exists() is often used in combination with other file system functions, such as is_file(), is_dir(), and unlink(), to perform more complex operations. Familiarize yourself with these functions to enhance your file and directory management capabilities.
4.4. Handle File Permissions
Ensure that your PHP scripts have the necessary file permissions to access the files or directories you’re checking. Use PHP’s chmod() function to modify permissions if needed.
Conclusion
PHP’s file_exists() function is a fundamental tool for file and directory management in web development. It allows you to check the existence of files and directories, enabling you to handle various scenarios gracefully. By following best practices and being aware of potential challenges, you can leverage file_exists() effectively in your PHP applications.
In this comprehensive guide, we’ve covered the syntax, use cases, edge cases, and best practices related to file_exists(). Armed with this knowledge and the provided code samples, you’re well-equipped to incorporate this function into your PHP projects with confidence.
Remember that robust file and directory handling is a critical aspect of web development, and file_exists() is a valuable addition to your PHP toolkit for achieving that. Whether you’re building a file upload system, validating user inputs, or managing configuration files, file_exists() is there to help you ensure the smooth operation of your web applications.
Table of Contents
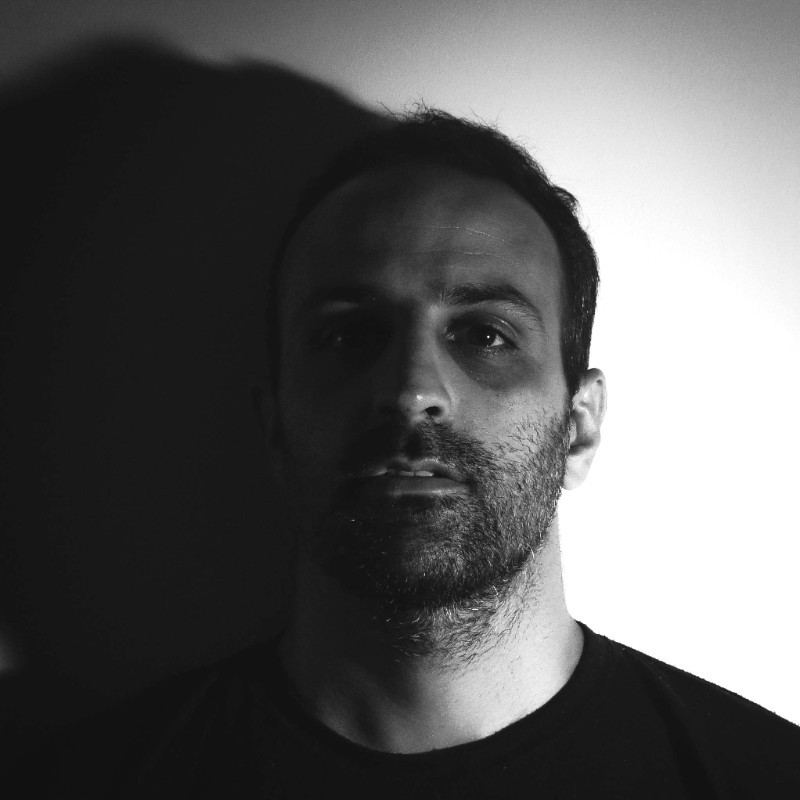
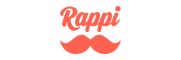