Mastering PHP Form Handling and Validation
In today’s digital world, web forms have become an integral part of website design and interaction. The ability to effectively handle and validate forms is crucial for any web developer, particularly those skilled in PHP, a popular server-side scripting language.
This necessity amplifies the demand to hire PHP developers who are adept at form handling and validation. This blog post aims to guide both developers and those looking to hire PHP developers, through the process of mastering PHP form handling and validation.
Understanding PHP Form Handling
Form handling is an essential process that allows interaction between users and web servers. In PHP, you can easily create HTML forms and use various PHP superglobal variables, like $_GET, $_POST, and $_REQUEST, to collect form data.
$_GET vs $_POST
These are both PHP superglobals that collect data sent from a form. The main difference between them lies in how they send this data:
– $_GET: Data is appended to the URL (visible), and it has limits on the amount of data to send.
– $_POST: Data is sent in the body of the HTTP request (invisible to users), and it has a higher capacity to handle data.
Basic PHP Form
Let’s start by creating a simple HTML form which will be processed by a PHP script.
```html <form method="post" action="welcome.php"> Name: <input type="text" name="name"><br> Email: <input type="text" name="email"><br> <input type="submit"> </form>
In the form above, the method attribute is set to “post“, and the action attribute points to the PHP file that will process the form data.
Now, let’s create a PHP script named “welcome.php” to handle the form data.
```php <?php $name = $_POST["name"]; $email = $_POST["email"]; echo "Welcome, " . $name . "<br>"; echo "Your email address is: " . $email; ?>
PHP Form Validation
While handling forms is essential, validating the data that comes from those forms is just as important. Form validation ensures that the data received is safe, secure, and aligns with your application’s expectations. This is a vital skill for PHP developers and a key factor when you are looking to hire PHP developers, as it helps in maintaining the integrity and security of your web application.
Below are some of the common validations:
– Required: Ensures that the field is not left empty.
– URL: Ensures that the field contains a valid URL.
– Email: Ensures that the field contains a valid email address.
– Numeric: Ensures that the field contains only numbers.
– Length: Ensures that the field’s length does not exceed a specified number of characters.
Here’s an example of how these validations can be applied using PHP:
```php <?php $name = $email = $website = ""; $nameErr = $emailErr = $websiteErr = ""; if ($_SERVER["REQUEST_METHOD"] == "POST") { if (empty($_POST["name"])) { $nameErr = "Name is required"; } else { $name = test_input($_POST["name"]); if (!preg_match("/^[a-zA-Z-' ]*$/",$name)) { $nameErr = "Only letters and white space allowed"; } } if (empty($_POST["email"])) { $emailErr = "Email is required"; } else { $email = test_input($_POST["email"]); if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $emailErr = "Invalid email format"; } } if (empty($_POST["website"])) { $website = ""; } else { $website = test_input($_POST["website"]); if (!filter_var($website, FILTER_VALIDATE_URL )) { $websiteErr = "Invalid URL"; } } } function test_input($data) { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data; } ?>
In the code snippet above, we’re checking whether the “name”, “email”, and “website” fields are empty and we’re using PHP’s in-built functions like `preg_match()`, `filter_var()` to check for a valid name, email, and URL respectively.
Advanced PHP Form Validation
Now that you have the basics, let’s discuss some advanced PHP form validation techniques:
CSRF Protection
Cross-Site Request Forgery (CSRF) is a type of attack that forces an end user to execute unwanted actions on a web application in which they’re authenticated. To prevent this, you can use CSRF tokens in your form. A CSRF token is a unique, random value associated with a user’s session, which is added as a hidden field in the form and validated on form submission.
```php session_start(); $token = bin2hex(random_bytes(32)); $_SESSION['token'] = $token; ``` In your form, you should include this token as a hidden field: ```html <input type="hidden" name="token" value="<?php echo $_SESSION['token']; ?>"> ``` On form submission, validate the token: ```php if($_POST['token'] !== $_SESSION['token']) { die('Invalid CSRF token'); }
Protecting Against SQL Injection
SQL injection is a code injection technique that attackers use to insert malicious SQL code into a query. To prevent this, you can use prepared statements or parameterized queries to separate SQL logic from the data being supplied.
Using PHP’s PDO extension:
```php $stmt = $pdo->prepare('SELECT * FROM users WHERE email = :email'); $stmt->execute(['email' => $email]); $user = $stmt->fetch();
Using MySQLi:
```php $stmt = $mysqli->prepare('SELECT * FROM users WHERE email = ?'); $stmt->execute([$email]); $user = $stmt->fetch();
Conclusion
Mastering PHP form handling and validation is a sought-after skill when you’re looking to hire PHP developers. It involves understanding the basics of PHP form handling, implementing simple to complex validations, and integrating advanced security practices. Equipped with this knowledge, PHP developers can create more secure and effective web applications.
The security of your application is only as strong as its weakest link, making it important to always validate and sanitize user input, and stay updated with the latest security practices to protect your application against threats. This is a key consideration when you decide to hire PHP developers.
By mastering PHP form handling and validation, developers can ensure smooth user interactions and significantly increase the security and reliability of their applications. If you’re looking to hire PHP developers, this proficiency can be a significant factor in your decision-making process. So, whether you’re a budding PHP developer or an organization looking to hire PHP developers, keep practicing and implementing these techniques. Happy coding!
Table of Contents
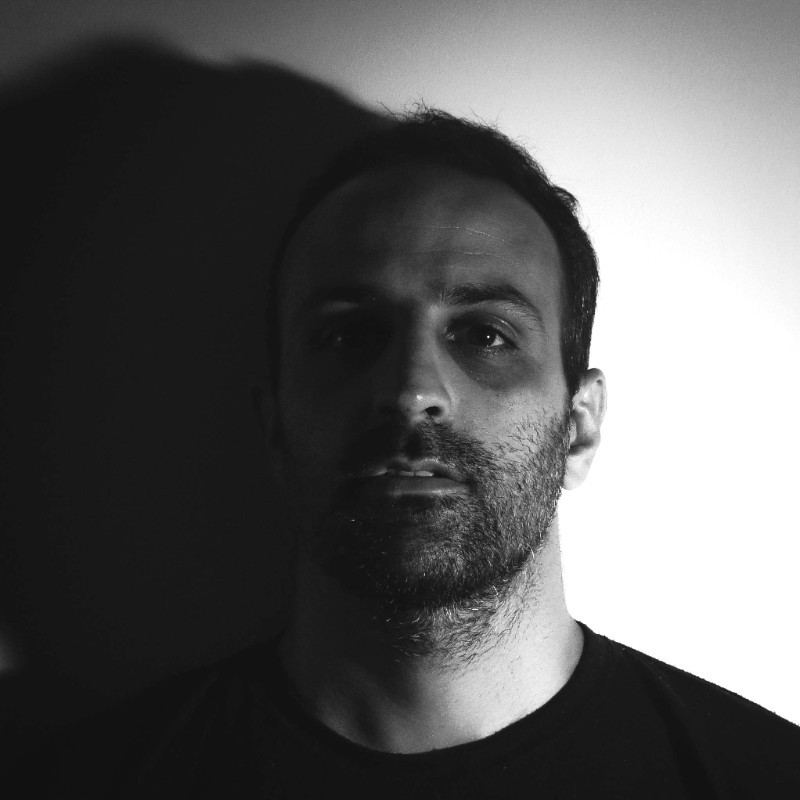
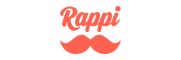