PHP Functions: An Essential Toolkit for Developers
PHP, a server-side scripting language designed specifically for web development, has become an indispensable tool for developers, including those businesses looking to hire. PHP developers, in particular, have a distinct advantage due to the language’s comprehensive library of built-in functions.
These functions provide pre-defined code to perform certain tasks, reducing the amount of code that needs to be written, improving readability, and making the code more efficient and maintainable. In this blog post, we’ll explore PHP functions in depth, demonstrating how they can serve as an essential toolkit for PHP developers, and why businesses should consider hiring PHP developers to leverage this powerful feature.
What are PHP Functions?
A function in PHP is a block of statements that can be used repeatedly in a program. A function will not execute automatically when a page loads. It will be called by a piece of code when needed.
function functionName() { // Code to be executed }
PHP comes packed with a massive list of built-in functions that do everything from array manipulation to XML parsing. While there are too many to list here, we will focus on some of the most useful and commonly used ones.
Essential PHP Functions
1. strlen(): This function returns the length of a string.
```php echo strlen("Hello world!"); // Outputs: 12
2. str_word_count(): This function returns the number of words in a string.
```php echo str_word_count("Hello world!"); // Outputs: 2
3. str_replace(): This function replaces some characters with some other characters in a string.
```php echo str_replace("world", "PHP", "Hello world!"); // Outputs: Hello PHP!
4. count(): This function returns the number of elements in an array.
```php $cars = array("Volvo", "BMW", "Toyota"); echo count($cars); // Outputs: 3
5. sort(): This function sorts arrays in ascending order.
```php $numbers = array(4, 6, 2, 22, 11); sort($numbers);
6. array_push(): This function inserts new items at the end of an array.
```php $fruits = array("apple", "banana"); array_push($fruits, "mango", "pineapple");
7. in_array(): This function checks if a value exists in an array.
```php $fruits = array("apple", "banana", "mango"); if(in_array("apple", $fruits)) { echo "Apple found"; }
8. date(): This function formats a local date and time.
```php echo date('Y-m-d H:i:s'); // Outputs: current date and time in '2023-06-22 10:30:00' format
9. isset(): This function checks if a variable is set and is not NULL.
```php $var = ""; if(isset($var)) { echo "Var is set"; }
10. empty(): This function checks whether a variable is empty.
```php $var = ""; if(empty($var)) { echo "Var is empty"; }
User-Defined Functions
Besides built-in functions, PHP allows you to define your own functions. A function definition in PHP consists of a function keyword, followed by a function name, a pair of parentheses for containing parameters, and a pair of curly braces that define the function body.
```php function sayHello() { echo "Hello, world!"; } sayHello(); // Outputs: Hello, world!
User-defined functions empower developers, particularly PHP developers, to create complex operations without having to repeatedly write the same code. This efficiency is one reason why businesses often hire PHP developers. They also provide the ability to handle specific tasks that built-in functions can’t address. The unique expertise that PHP developers bring to the table, especially in terms of crafting effective user-defined functions, makes them a valuable asset for companies looking to streamline their web development processes.
Function Parameters
Parameters are like placeholders for actual values that are passed to the function when it is called. They allow the function to accept input(s), process it, and return a value.
```php function addNumbers($num1, $num2) { $sum = $num1 + $num2; echo $sum; } addNumbers(5, 10); // Outputs: 15
Variable Scope
When you use variables inside a function, they are by default local to that function. PHP functions do not automatically have access to the global scope.
If you want to access a global variable inside a function, you can use the `global` keyword:
```php $x = 5; $y = 10; function test() { global $x, $y; $y = $x + $y; } test(); echo $y; // Outputs: 15
Conclusion
Functions in PHP are a powerful tool in the hands of a developer, making those proficient in its use highly sought after. That’s why businesses often choose to hire PHP developers. PHP functions allow developers to write cleaner, more modular, and more maintainable code. A deep understanding of how to use built-in functions, create user-defined functions, and manage variable scope is a fundamental skill for PHP developers. By effectively utilizing these functions, PHP developers can make code more efficient and readable, leading to better software and a smoother development experience. This is one of the many reasons why companies choose to hire PHP developers, as they can leverage these benefits to improve their software solutions.
Table of Contents
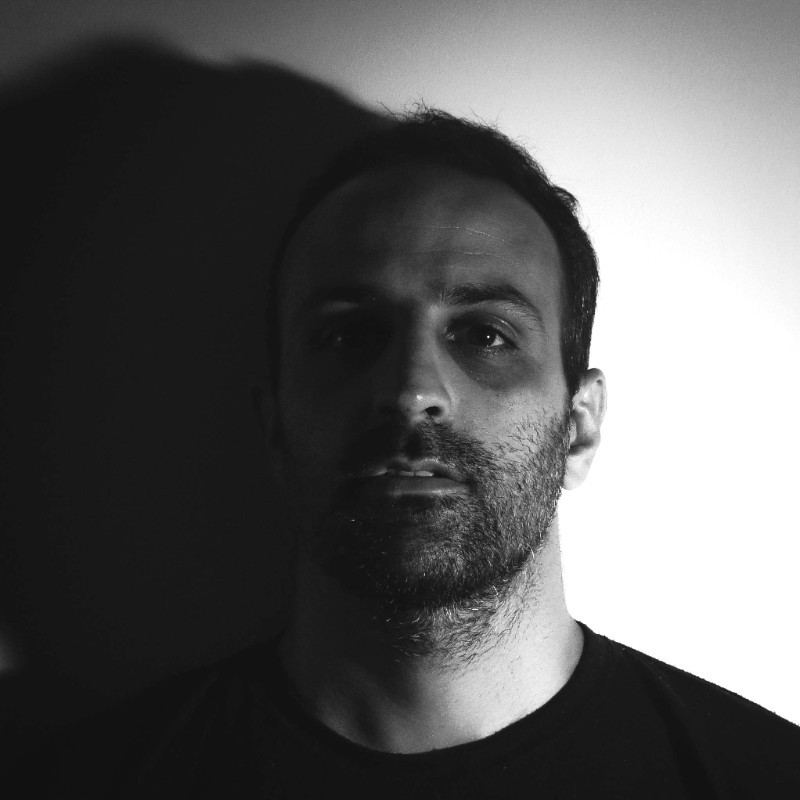
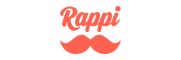