Deciphering PHP’s gettype() Function: A Detailed Guide
PHP is a versatile and widely-used scripting language known for its ability to handle various data types and operations. As a PHP developer, you’ll often find yourself needing to work with different types of variables, from simple integers and strings to more complex arrays and objects. To effectively manipulate these variables, it’s crucial to understand their types. This is where PHP’s gettype() function comes into play.
Table of Contents
In this comprehensive guide, we will delve into the gettype() function, exploring its purpose, syntax, and practical applications. By the end of this article, you’ll have a solid grasp of how to use gettype() to determine variable types in PHP and why it’s a valuable tool in your development arsenal.
1. Understanding Variable Types in PHP
Before diving into the intricacies of the gettype() function, let’s briefly review the common variable types in PHP:
1.1. Integer
An integer is a whole number without a decimal point. For example, $age = 30;
1.2. Float (Floating-Point Numbers)
Floats are numbers with decimal points or numbers in scientific (exponential) notation. For example, $price = 19.99;
1.3. String
Strings are sequences of characters enclosed in single or double quotes. For example, $name = “John”;
1.4. Boolean
Boolean values can be either true or false. For example, $is_valid = true;
1.5. Array
Arrays are ordered, map-like data structures that can store multiple values. For example, $colors = [‘red’, ‘green’, ‘blue’];
1.6. Object
Objects are instances of user-defined classes and can contain both properties and methods. For example, $person = new Person();
1.7. NULL
The NULL type represents a variable with no value. For example, $status = null;
Knowing the types of variables you’re working with is essential when designing robust PHP applications. This is where the gettype() function proves invaluable.
2. Introducing the gettype() Function
The gettype() function in PHP is designed to return the type of a given variable. Its syntax is straightforward:
php string gettype(mixed $variable)
Here, $variable is the variable whose type you want to determine. gettype() returns a string representing the type of $variable. Let’s explore its usage with some practical examples.
Example 1: Checking an Integer
php $age = 30; $type = gettype($age); echo "The variable $age is of type $type"; // Output: The variable $age is of type integer
In this example, we declare a variable $age and use gettype() to determine its type, which is integer. We then display this information using echo.
Example 2: Checking an Object
php class Car { public $brand; public $model; } $myCar = new Car(); $type = gettype($myCar); echo "The variable $myCar is of type $type"; // Output: The variable $myCar is of type object
Here, we define a class Car and create an instance of it named $myCar. Using gettype(), we confirm that $myCar is of type object.
Example 3: Handling NULL Values
php $status = null; $type = gettype($status); echo "The variable $status is of type $type"; // Output: The variable $status is of type NULL
In this example, we set $status to null and then use gettype() to determine that it is of type NULL.
Example 4: Dynamic Typing
PHP is a dynamically typed language, which means that variable types can change during runtime. gettype() can help you manage this flexibility:
php $dynamicVar = "Hello, World!"; $type = gettype($dynamicVar); echo "The variable $dynamicVar is of type $type"; // Output: The variable $dynamicVar is of type string $dynamicVar = 42; $type = gettype($dynamicVar); echo "The variable $dynamicVar is now of type $type"; // Output: The variable $dynamicVar is now of type integer
In this example, we start with $dynamicVar as a string, and then we change its type to an integer. gettype() accurately reflects these changes.
3. Practical Use Cases
Now that you understand how to use the gettype() function let’s explore some practical use cases where it can be beneficial:
3.1. Input Validation
When dealing with user inputs, it’s crucial to validate data types to ensure that your application receives the expected data. Here’s an example of how gettype() can be used for input validation:
php function validateAge($age) { if (gettype($age) === 'integer') { if ($age >= 18 && $age <= 99) { return "Valid age: $age"; } else { return "Invalid age: $age"; } } else { return "Invalid data type: " . gettype($age); } } $input1 = 25; $input2 = "30"; echo validateAge($input1); // Output: Valid age: 25 echo validateAge($input2); // Output: Invalid data type: string
In this example, we define a validateAge function that checks if the provided $age is of type integer and within a valid range.
3.2. Debugging
During development, you may encounter unexpected behavior in your code. gettype() can be a useful tool for debugging to understand the types of variables at various points in your code:
php function complexCalculation($input) { // Some complex calculations... $result = $input * 2; // Debugging: Log the type of $input $inputType = gettype($input); error_log("Type of $input is $inputType"); return $result; } $input = "5"; $result = complexCalculation($input); // Output: Type of $input is string
In this example, we use gettype() to log the type of the $input variable within a function for debugging purposes.
3.3. Database Interaction
When working with databases, data types must match the database schema. gettype() can help ensure that data sent to the database is of the correct type:
php // Assume a database table 'users' with an 'age' column of type INT $userId = 123; $age = "30"; if (gettype($age) === 'integer') { // Perform database query $query = "UPDATE users SET age = $age WHERE id = $userId"; // Execute the query... } else { // Handle data type mismatch error echo "Data type mismatch for age."; }
Here, before executing the database query, we use gettype() to check if the $age variable is of type integer to prevent data type mismatches.
4. Handling Special Cases
While gettype() is generally useful, there are some special cases to be aware of:
4.1. Objects and Class Types
When you use gettype() with objects, it returns “object” for all object instances, regardless of their class. To determine the class of an object, you should use instanceof. Here’s an example:
php class Dog {} class Cat {} $myDog = new Dog(); $myCat = new Cat(); $typeDog = gettype($myDog); $typeCat = gettype($myCat); echo "Type of $myDog: $typeDog"; // Output: Type of $myDog: object echo "Type of $myCat: $typeCat"; // Output: Type of $myCat: object $isDog = ($myDog instanceof Dog); $isCat = ($myCat instanceof Cat); echo "Is $myDog a Dog? " . ($isDog ? "Yes" : "No"); // Output: Is $myDog a Dog? Yes echo "Is $myCat a Cat? " . ($isCat ? "Yes" : "No"); // Output: Is $myCat a Cat? Yes
In this example, gettype() returns “object” for both $myDog and $myCat, but instanceof allows us to determine their actual classes.
4.2. Resource Type
PHP has a resource type for handling external resources, such as file handles or database connections. gettype() returns “resource” for these types, but it doesn’t provide specific information about the resource. To work with resources, you may need to use resource-specific functions.
php $fileHandle = fopen("example.txt", "r"); $type = gettype($fileHandle); echo "Type of $fileHandle: $type"; // Output: Type of $fileHandle: resource // You would use resource-specific functions to work with $fileHandle
4.3. Arrays and Associative Arrays
gettype() returns “array” for both indexed arrays and associative arrays. If you need to distinguish between the two, you can use is_array() and array_key_exists():
php $indexedArray = [1, 2, 3]; $assocArray = ["name" => "John", "age" => 30]; $isIndexed = is_array($indexedArray); $isAssoc = is_array($assocArray); echo "Is $indexedArray an indexed array? " . ($isIndexed ? "Yes" : "No"); // Output: Is $indexedArray an indexed array? Yes echo "Is $assocArray an associative array? " . ($isAssoc ? "Yes" : "No"); // Output: Is $assocArray an associative array? Yes
In this example, we use is_array() to differentiate between indexed and associative arrays.
Conclusion
PHP’s gettype() function is a versatile tool for determining variable types in your PHP code. Whether you’re validating user inputs, debugging your application, or working with databases, understanding variable types is crucial for writing reliable and maintainable code.
In this detailed guide, we’ve explored the syntax and practical applications of gettype(). By incorporating it into your PHP development workflow, you can gain better control over your variables, improve code quality, and reduce the likelihood of type-related bugs.
Remember that while gettype() is a valuable resource, it has limitations, especially when dealing with objects, resources, and distinguishing between types within the “array” category. In such cases, additional PHP functions and operators come into play.
As you continue to develop your PHP skills, mastering the use of functions like gettype() will contribute to your effectiveness as a developer. So, go ahead and start using gettype() in your projects to make your PHP code more robust and reliable.
Table of Contents
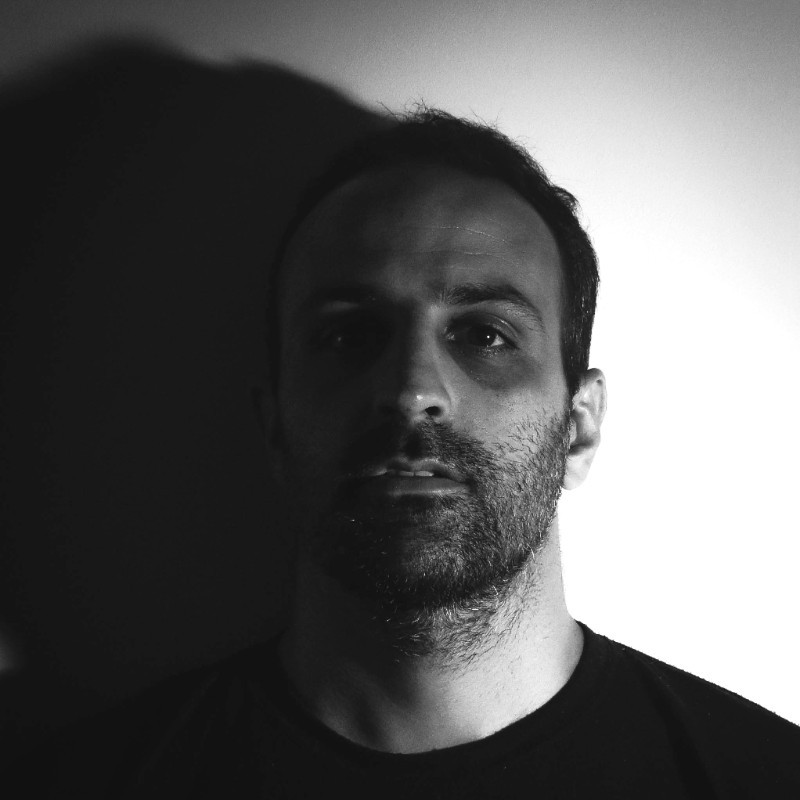
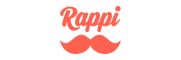