PHP’s glob() Function: Finding Files Made Easy
In the world of web development, managing files is a common and crucial task. Whether you’re building a dynamic website, processing user-uploaded content, or performing system maintenance, the ability to locate and manipulate files efficiently is essential. PHP, a versatile and widely-used scripting language, offers a handy tool for this purpose: the glob() function. In this article, we’ll delve into the details of the glob() function, exploring its features, advantages, and providing real-world code examples.
Table of Contents
1. Understanding the glob() Function
The glob() function in PHP is a powerful utility that simplifies the process of searching for files and directories in a specified directory path using wildcard patterns. This function returns an array of filenames that match the given pattern, allowing developers to quickly retrieve a list of relevant files for further processing. The beauty of glob() lies in its flexibility – it supports a variety of wildcard characters, making it easy to filter files based on different criteria.
2. Benefits of Using glob()
2.1. Ease of Use
One of the primary advantages of using the glob() function is its simplicity. The function’s syntax is straightforward, requiring only the path and the pattern as arguments. Developers can quickly integrate it into their code without extensive configuration or setup.
2.2. Wildcard Support
The glob() function supports a range of wildcard characters that enable precise file matching. The most commonly used wildcards are:
- *: Matches any number of characters.
- ?: Matches a single character.
- []: Matches any character within the brackets.
- {}: Matches any of the comma-separated patterns inside the curly braces.
This wildcard support allows developers to create dynamic patterns that cater to specific file naming conventions.
2.3. Recursive Searching
With the glob() function, you can perform recursive searches through subdirectories. By including the GLOB_BRACE flag, you can extend the pattern matching to include subdirectories, making it convenient to find files regardless of their location within the directory structure.
3. Using glob() in Real-World Scenarios
Let’s explore some practical examples to better understand how the glob() function can be applied in real-world scenarios.
3.1. Listing Image Files
Suppose you’re building a photo gallery application, and you want to retrieve a list of all image files (JPEG, PNG, and GIF) within a specific directory. Here’s how you can accomplish this using the glob() function:
php $imageFiles = glob('/path/to/images/*.{jpg,png,gif}', GLOB_BRACE); print_r($imageFiles);
In this example, the pattern /path/to/images/*.{jpg,png,gif} specifies that you’re looking for files with the extensions jpg, png, or gif in the images directory. The GLOB_BRACE flag enables brace expansion for multiple patterns.
3.2. Finding Configuration Files
Imagine you’re working on a content management system that uses different configuration files spread across various subdirectories. To locate all configuration files (with .conf extension) regardless of their depth within the project structure, you can use the recursive feature of the glob() function:
php $configFiles = glob('/path/to/project/**/*.{conf}', GLOB_BRACE | GLOB_MARK | GLOB_ONLYFILES); print_r($configFiles);
Here, /path/to/project/**/*.{conf} searches for .conf files within all subdirectories of the project. The GLOB_MARK flag ensures that directories are marked with a trailing slash, and GLOB_ONLYFILES excludes directories from the results.
3.3. Cleaning Up Temporary Files
In a web application, temporary files can accumulate over time and clutter the server’s storage. To locate and remove all temporary files (files with .tmp extension) within a specific folder, you can use the glob() function in conjunction with other PHP functions:
php $tempFiles = glob('/path/to/temp_folder/*.tmp'); foreach ($tempFiles as $file) { unlink($file); // Delete the temporary file }
In this example, glob() fetches all .tmp files in the temp_folder, and a loop iterates through the array to delete each file using the unlink() function.
4. Best Practices and Considerations
While the glob() function offers a convenient way to search for files, there are a few best practices and considerations to keep in mind:
- Error Handling: Always include error handling when using glob(). If the specified directory does not exist or the pattern doesn’t match any files, glob() will return false. Handle these cases gracefully to prevent unexpected behavior in your code.
- Performance: While glob() is efficient for most use cases, it might not be the best choice for extremely large directories or deeply nested structures. In such cases, consider alternative approaches like iterating through directories manually.
- Security: Be cautious when dealing with user-generated input in patterns. Improperly sanitized input could lead to unexpected results or even directory traversal vulnerabilities. Sanitize and validate user input before using it in glob() patterns.
Conclusion
The glob() function in PHP provides a simple yet effective way to locate and manipulate files within a directory. Its support for wildcard patterns, recursive searching, and ease of use make it a valuable tool in various web development scenarios. By integrating glob() into your projects, you can streamline file management and enhance the efficiency of your applications. Remember to follow best practices and consider the potential pitfalls to make the most out of this versatile function. Happy coding!
Table of Contents
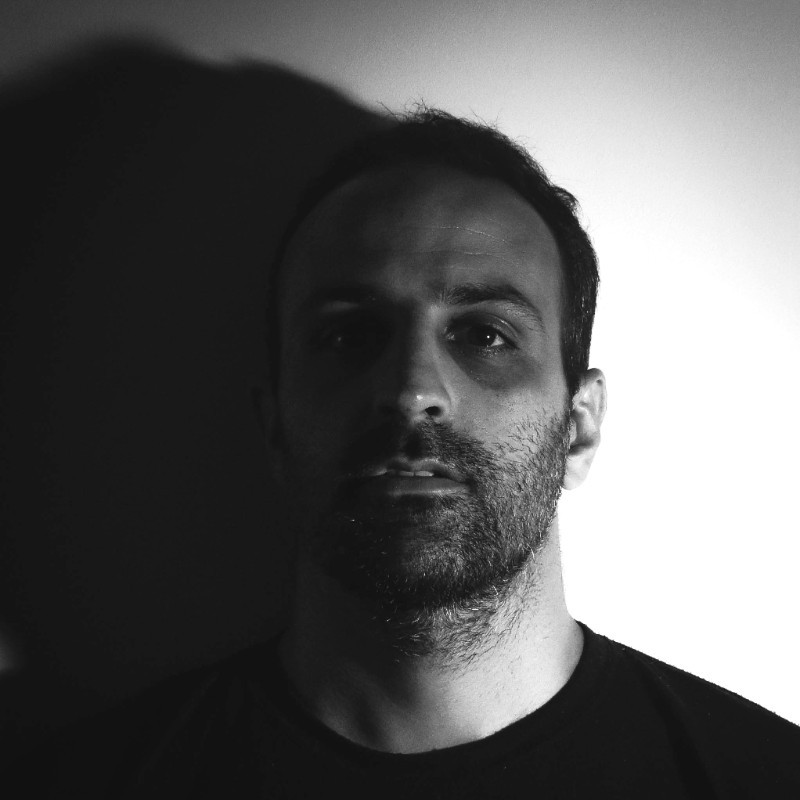
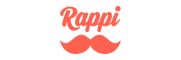