PHP Q & A
How to handle file uploads securely?
Handling file uploads securely in PHP is crucial to prevent potential security vulnerabilities and ensure the integrity of your web application. Here’s a comprehensive guide on how to handle file uploads securely in PHP:
- Validate File Types: Before processing an uploaded file, validate its file type using the `$_FILES` array and the `filetype` function. Check if the file type matches the expected types (e.g., allow only images or specific document formats). This helps prevent malicious users from uploading harmful files.
```php $allowedTypes = ['image/jpeg', 'image/png', 'application/pdf']; if (!in_array($_FILES['file']['type'], $allowedTypes)) { die("Invalid file type."); } ```
- Limit File Size: Set a maximum file size limit using the `upload_max_filesize` and `post_max_size` directives in your PHP configuration (php.ini). Additionally, you can check the file size in PHP code:
```php $maxSize = 5 * 1024 * 1024; // 5 MB if ($_FILES['file']['size'] > $maxSize) { die("File size exceeds the limit."); } ```
- Rename Uploaded Files: Avoid using the original filename provided by users. Generate a unique filename to prevent conflicts and directory traversal attacks. You can use a combination of timestamps and random strings for this purpose.
- Use a Secure Upload Directory: Store uploaded files in a separate directory outside the web root (e.g., `/var/uploads`) to prevent direct access to uploaded files via URLs. Configure the directory permissions to ensure that only the web server user can write to it.
- Validate File Contents: Check the content of the uploaded file to ensure it matches the expected format. For example, if you expect an image file, use image-processing libraries to verify that the file is a valid image.
- Sanitize File Data: Remove any potentially harmful characters or data from file names and content to prevent SQL injection or other attacks. Use functions like `htmlspecialchars` and prepared statements when interacting with databases.
- Implement Access Controls: If the uploaded files contain sensitive data, consider implementing access controls and user authentication to restrict access to authorized users only.
- Regularly Clean Up: Regularly clean up your upload directory by removing unused or outdated files to prevent the accumulation of unnecessary data.
By following these security best practices, you can ensure that your PHP application safely handles file uploads, minimizing security risks and potential vulnerabilities. Always stay updated with the latest PHP security recommendations and regularly test your application for vulnerabilities.
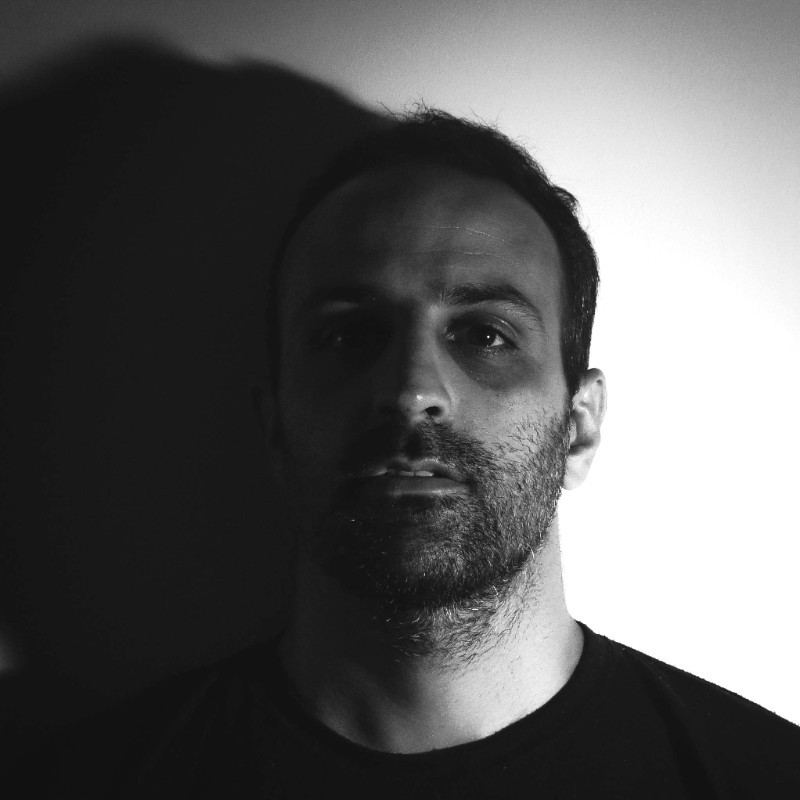
Previously at
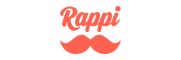
Full Stack Engineer with extensive experience in PHP development. Over 11 years of experience working with PHP, creating innovative solutions for various web applications and platforms.