Understanding PHP’s header() Function: Redirects and More
In the world of web development, creating dynamic and interactive websites is a necessity. One crucial aspect of this process is managing how a web server communicates with a client’s browser. This is where the PHP header() function comes into play, offering developers the ability to send HTTP headers to the browser. While it might sound technical, mastering this function can significantly enhance your web development skills. In this guide, we’ll delve deep into the header() function, focusing on its role in performing redirects and exploring its broader capabilities.
1. The Basics of the header() Function
1.1. What Are HTTP Headers?
HTTP (Hypertext Transfer Protocol) headers are crucial pieces of information that a web server sends to a client’s browser before transmitting the actual page content. These headers provide information about the server’s response, such as the content type, response code, and other important metadata. By utilizing the header() function, PHP developers gain the ability to control these headers and tailor the server’s response to suit specific requirements.
1.2. Syntax of the header() Function
The basic syntax of the header() function is as follows:
php header(string $header, bool $replace = true, int $http_response_code = 0);
- $header: The header to be sent, in the format “HeaderName: HeaderValue”.
- $replace: A boolean value that determines whether to replace a previous similar header.
- $http_response_code: An optional HTTP response code to be sent with the header.
2. Redirecting with header()
One of the most common applications of the header() function is performing redirects. Redirects are essential for guiding users to the correct location after a certain action or when a page is moved permanently or temporarily.
2.1. Performing a 301 Redirect
A 301 redirect indicates a permanent move of a page to a new location. This is particularly useful when you’ve updated the URL structure of your website, but you want to ensure that users and search engines are directed to the new URLs. Here’s an example of how you can use the header() function for a 301 redirect:
php header("HTTP/1.1 301 Moved Permanently"); header("Location: https://www.newexample.com/new-page"); exit();
In this example, the header() function sends the appropriate HTTP response code (301) along with the new location. The exit() function is used to halt the script execution immediately after sending the header.
2.2. Implementing a 302 Redirect
A 302 redirect signifies a temporary move of a page to a new location. Unlike a 301 redirect, this indicates that the move might not be permanent. The process is similar to a 301 redirect:
php header("HTTP/1.1 302 Found"); header("Location: https://www.example.com/temporary-page"); exit();
2.3. Considerations for SEO
When implementing redirects, especially permanent ones (301), it’s important to consider the implications for search engine optimization (SEO). Search engines like Google take note of 301 redirects and transfer the SEO value from the old URL to the new one. However, frequent or improper redirects can negatively impact SEO rankings, so use them judiciously.
3. Additional Uses of header()
Beyond redirects, the header() function has several other applications that can enhance your website’s performance and security.
3.1. Controlling Caching
HTTP headers play a crucial role in determining how browsers cache content. By setting cache-related headers using the header() function, you can control how often browsers retrieve content from the server and how they store it locally. For instance, you can set the Cache-Control header to specify caching directives:
php header("Cache-Control: max-age=3600, public");
In this example, the browser is instructed to cache the content for a maximum of 3600 seconds (1 hour).
3.2. Preventing Clickjacking
Clickjacking is a security vulnerability where a malicious website can trick users into clicking on something different from what they perceive. You can mitigate clickjacking risks by using the X-Frame-Options header:
php header("X-Frame-Options: DENY");
This header instructs the browser not to render the page in a frame or iframe, thereby preventing clickjacking attacks.
3.3. MIME Type Specification
MIME (Multipurpose Internet Mail Extensions) types are used to identify the type of data being sent over the internet. By setting the Content-Type header, you can ensure that browsers interpret your content correctly. For example, to indicate that you’re sending JSON data:
php header("Content-Type: application/json");
4. Common Pitfalls and Best Practices
While the header() function offers powerful capabilities, there are certain pitfalls you should be aware of, along with best practices to ensure smooth execution.
4.1. Avoiding Output Before header()
Headers must be sent before any actual content is output to the browser. If you attempt to set headers after outputting content, PHP will throw an error. To avoid this, make sure to call the header() function before using functions like echo or any HTML content.
4.2. Ensuring a Single Execution Point
Headers should ideally be set at a single execution point in your script, preferably near the top. Setting headers at multiple points can lead to unexpected behavior and errors. Creating a clear structure for your script can help prevent header-related issues.
4.3. Proper Error Handling
When setting headers, it’s crucial to implement proper error handling. If a header cannot be set for some reason (e.g., headers were already sent), PHP will generate a warning. To handle these situations gracefully, you can use the header() function in combination with the header_sent() function and conditional statements.
5. Advanced Techniques
5.1. Conditional Redirects
You can implement conditional redirects using the header() function to guide users based on specific conditions. For instance, if you want to redirect users to a login page if they’re not authenticated:
php if (!$authenticated) { header("Location: https://www.example.com/login"); exit(); }
5.2. Delayed Redirects
In some cases, you might want to delay a redirect to provide users with important information before they’re redirected. You can achieve this using the sleep() function:
php echo "You'll be redirected in a moment..."; sleep(3); // Delay for 3 seconds header("Location: https://www.example.com/new-page"); exit();
5.3. Custom Response Codes
While HTTP response codes like 301 and 302 are commonly used, you can also create custom response codes using the header() function. This can be useful for conveying specific information to the browser or client:
php header("HTTP/1.1 450 Blocked by User Preferences");
Conclusion
The PHP header() function is a versatile tool that empowers developers to control various aspects of communication between a web server and a client’s browser. From performing redirects to managing caching and enhancing security, this function plays a pivotal role in modern web development. By mastering its applications and adhering to best practices, developers can create more dynamic and user-friendly websites while ensuring optimal performance and security. As you continue to explore PHP and its capabilities, understanding the header() function will undoubtedly prove to be a valuable asset in your development journey.
Table of Contents
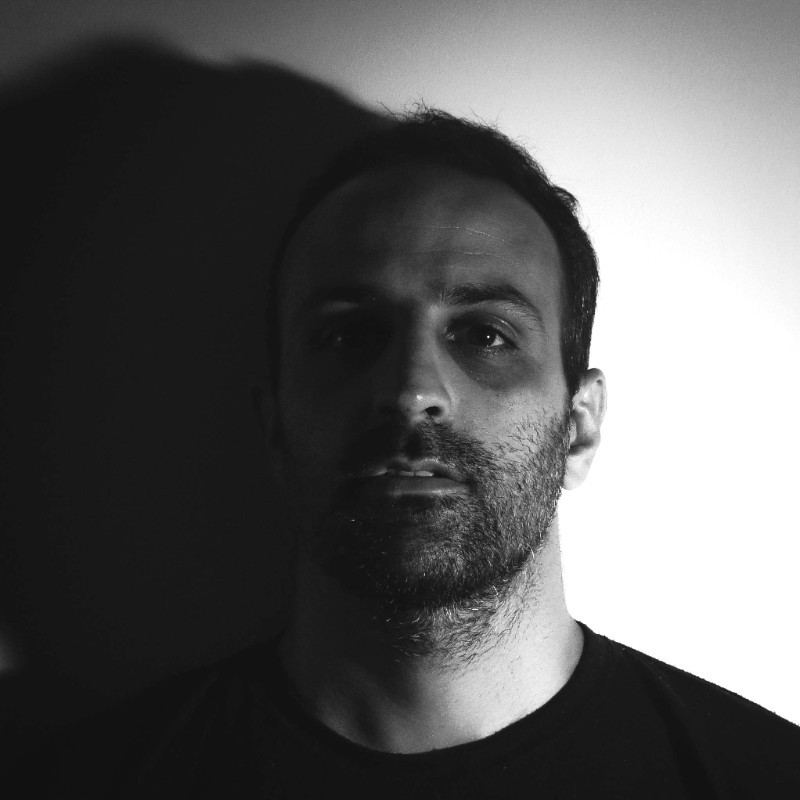
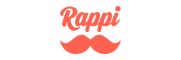