How to create and send HTML emails?
Creating and sending HTML emails in PHP is a common requirement, especially when you want to send visually appealing and formatted emails. Here’s a step-by-step guide on how to achieve this:
- Set Up Your Environment:
Before you begin, ensure that your PHP environment is configured to send emails. You can use libraries like PHPMailer or the built-in `mail()` function. PHPMailer is a popular choice as it offers better control and flexibility.
- Install PHPMailer (Optional):
If you choose to use PHPMailer, you need to download and include it in your project. You can use Composer, a PHP package manager, to easily install PHPMailer and manage its dependencies.
- Create an HTML Email Template:
Design an HTML template for your email. This template should include the email’s content, styling, and any images you want to include. Make sure your HTML is well-structured and responsive for different email clients.
- PHP Script for Sending Email:
Write a PHP script to send the HTML email. Here’s a basic example using PHPMailer:
```php use PHPMailer\PHPMailer\PHPMailer; use PHPMailer\PHPMailer\Exception; require 'vendor/autoload.php'; // Include PHPMailer library $mail = new PHPMailer(true); try { $mail->isSMTP(); $mail->SMTPAuth = true; $mail->Host = 'smtp.example.com'; // Your SMTP server $mail->Username = 'your_email@example.com'; // Your email address $mail->Password = 'your_password'; // Your email password $mail->SMTPSecure = 'tls'; $mail->Port = 587; $mail->setFrom('your_email@example.com', 'Your Name'); $mail->addAddress('recipient@example.com', 'Recipient Name'); $mail->isHTML(true); $mail->Subject = 'Subject of your email'; $mail->Body = '<html><body>Your HTML content here</body></html>'; $mail->send(); echo 'Email sent successfully'; } catch (Exception $e) { echo 'Message could not be sent. Mailer Error: ', $mail->ErrorInfo; } ```
- Customize Email Content:
Replace placeholders in your HTML template with dynamic data, if needed. You can use PHP variables to inject personalized content into your emails.
- Send the Email:
Execute the PHP script to send the email. The recipient will receive a beautifully formatted HTML email.
- Testing and Debugging:
Test your HTML email across various email clients and devices to ensure it renders correctly. Debug any issues that may arise during testing.
- Attachments and Additional Features (Optional):
PHPMailer and other libraries offer features like attaching files, sending emails to multiple recipients, and handling different email protocols (e.g., SMTP, sendmail).
By following these steps and using a library like PHPMailer, you can create and send HTML emails in PHP with ease, providing a visually appealing and effective means of communication with your audience.
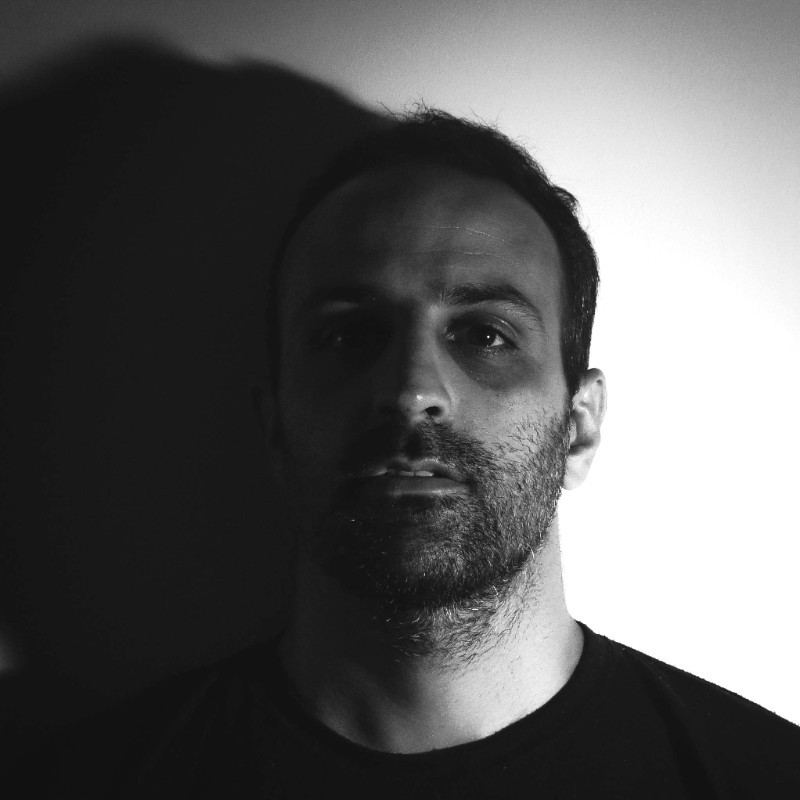
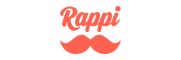