How to use if statements?
Conditional statements, such as “if” statements, are fundamental in PHP for making decisions and controlling the flow of your code. An “if” statement in PHP allows you to execute a block of code conditionally based on whether a specified condition is true or false. Here’s a comprehensive guide on how to use “if” statements in PHP:
Basic “if” Statement:
The basic structure of an “if” statement in PHP is as follows:
```php if (condition) { // Code to execute if the condition is true } ```
– The condition is an expression that evaluates to either true or false.
– If the condition is true, the code within the curly braces will be executed. If the condition is false, the code will be skipped.
Example:
```php $age = 25; if ($age >= 18) { echo "You are an adult."; } ```
In this example, the code checks if the variable `$age` is greater than or equal to 18. If the condition is true (which it is with a value of 25), it prints “You are an adult.”
“if-else” Statement:
You can extend the basic “if” statement with an “else” block to specify what should happen when the condition is false:
```php if (condition) { // Code to execute if the condition is true } else { // Code to execute if the condition is false } ```
Example:
```php $temperature = 28; if ($temperature > 30) { echo "It's hot outside."; } else { echo "It's not too hot."; } ```
In this example, the code checks the value of `$temperature` and prints different messages based on whether it’s greater than 30 or not.
“if-elseif-else” Statement:
You can also use multiple conditions with “if-elseif-else” statements to handle more complex scenarios:
```php if (condition1) { // Code to execute if condition1 is true } elseif (condition2) { // Code to execute if condition2 is true } else { // Code to execute if none of the conditions are true } ```
Example:
```php $score = 75; if ($score >= 90) { echo "You got an A."; } elseif ($score >= 80) { echo "You got a B."; } else { echo "You got a C or lower."; } ```
In this example, the code evaluates the value of `$score` and prints a corresponding message based on the grading criteria.
These are the fundamental concepts of using “if” statements in PHP. Conditional statements are crucial for controlling the logic and behavior of your PHP scripts, making them a fundamental building block in programming and web development.
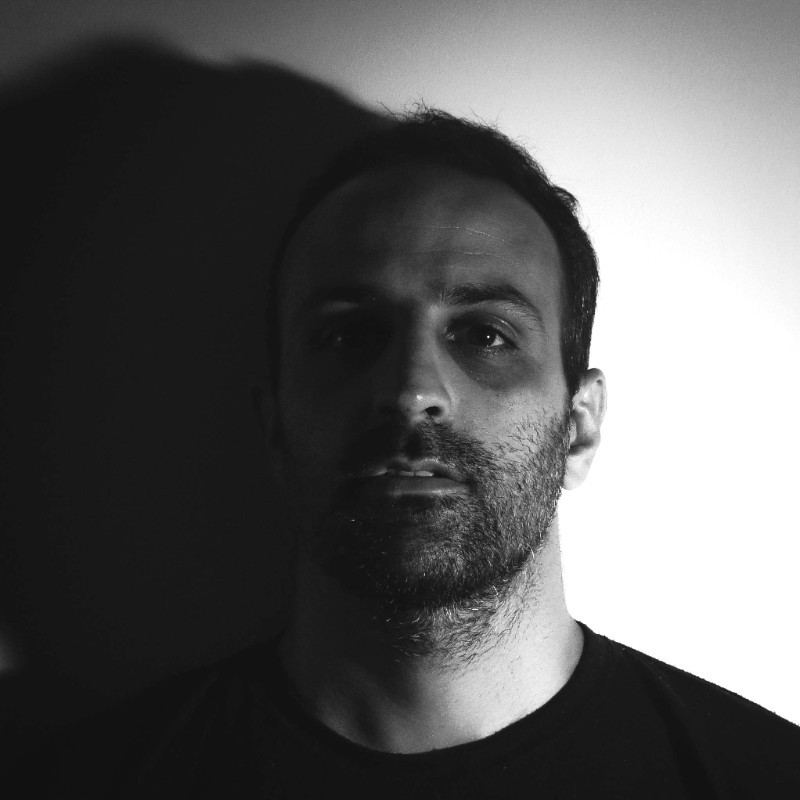
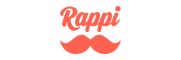