Exploring PHP’s imagecreate() Function: A Guide to GD Library
In the world of web development, creating and manipulating images on the fly is a common requirement. Whether it’s generating thumbnails, adding watermarks, or creating dynamic charts, PHP provides a versatile library for working with images. One of the key functions in this library is imagecreate(), which allows you to create and manipulate images directly from your PHP code. In this guide, we’ll explore the imagecreate() function and dive into the powerful GD Library, providing you with the knowledge and tools to enhance your web applications with dynamic image generation.
Table of Contents
1. Introduction to GD Library and imagecreate()
1.1. What is GD Library?
GD (Graphics Draw) Library is a powerful and versatile graphics library that is often used in PHP to create and manipulate images. It provides functions to create, read, write, and manipulate various image formats, making it an essential tool for dynamic image generation in web applications.
1.2. Why Use imagecreate()?
The imagecreate() function is the gateway to the GD Library in PHP. It allows you to create a new true-color image of a specified size, which can be used as a canvas for various image operations. This function opens up a world of possibilities for generating charts, graphs, dynamic images, and more, all within your PHP code.
2. Getting Started with GD Library
2.1. Installing GD Library
Before you can start using imagecreate(), you need to ensure that the GD Library is installed on your server. Most modern PHP installations come with GD Library support by default. To check if GD is enabled, you can create a simple PHP script:
php <?php // Check for GD Library support if (extension_loaded('gd') && function_exists('gd_info')) { echo "GD Library is installed and enabled."; } else { echo "GD Library is not installed or enabled."; } ?>
2.2. Checking GD Library Support
If you see the message “GD Library is installed and enabled,” you’re good to go. If not, you may need to install or enable the GD Library on your server, which varies depending on your server’s operating system and PHP configuration.
3. Using imagecreate()
Now that you have GD Library up and running let’s dive into the imagecreate() function and explore how it can be used to create and manipulate images.
3.1. Creating a Blank Image
The imagecreate() function allows you to create a new blank image with the specified width and height. Here’s a simple example of creating a 300×200-pixel blank image:
php <?php // Create a blank image with a white background $image = imagecreate(300, 200); // Output the image as a PNG header('Content-Type: image/png'); imagepng($image); // Clean up resources imagedestroy($image); ?>
In this code, we first create a blank image with a white background using imagecreate(300, 200). Then, we set the content type to PNG and output the image using imagepng(). Finally, we clean up the resources using imagedestroy().
3.2. Allocating Colors
To draw on the image, you’ll need to allocate colors. GD Library uses a color index to represent colors, and you can allocate colors using the imagecolorallocate() function. Here’s an example of drawing a red rectangle on a blank image:
php <?php // Create a blank image with a white background $image = imagecreate(300, 200); // Allocate the color red $red = imagecolorallocate($image, 255, 0, 0); // Draw a red rectangle imagefilledrectangle($image, 50, 50, 250, 150, $red); // Output the image as a PNG header('Content-Type: image/png'); imagepng($image); // Clean up resources imagedestroy($image); ?>
In this code, we allocate the color red using imagecolorallocate() with RGB values (255, 0, 0), and then we use imagefilledrectangle() to draw a red rectangle on the image.
3.3. Drawing Shapes and Text
GD Library provides various functions for drawing shapes and text on images. Here’s an example of drawing a blue circle and adding text to the image:
php <?php // Create a blank image with a white background $image = imagecreate(300, 200); // Allocate the color blue $blue = imagecolorallocate($image, 0, 0, 255); // Draw a blue circle imagefilledellipse($image, 150, 100, 100, 100, $blue); // Allocate the color black for text $black = imagecolorallocate($image, 0, 0, 0); // Add text to the image $text = "Hello, GD Library!"; imagettftext($image, 20, 0, 50, 150, $black, 'arial.ttf', $text); // Output the image as a PNG header('Content-Type: image/png'); imagepng($image); // Clean up resources imagedestroy($image); ?>
In this code, we draw a blue circle using imagefilledellipse() and add text to the image using imagettftext(). You can specify the font, size, rotation, and position of the text.
3.4. Adding Images
You can also overlay existing images onto your canvas. Here’s an example of adding a PNG image with transparency to a blank image:
php <?php // Create a blank image with a white background $image = imagecreate(300, 200); // Load the PNG image with transparency $overlay = imagecreatefrompng('overlay.png'); // Copy the overlay onto the blank image imagecopy($image, $overlay, 50, 50, 0, 0, imagesx($overlay), imagesy($overlay)); // Output the image as a PNG header('Content-Type: image/png'); imagepng($image); // Clean up resources imagedestroy($image); imagedestroy($overlay); ?>
In this code, we first create a blank image and then load an overlay image with transparency using imagecreatefrompng(). We then use imagecopy() to paste the overlay onto the blank image at the specified coordinates.
4. Advanced Techniques
4.1. Image Manipulation
GD Library provides a wide range of functions for image manipulation, including resizing, rotating, cropping, and applying filters. Here’s an example of resizing an image:
php <?php // Load an existing image $image = imagecreatefromjpeg('original.jpg'); // Get the current dimensions of the image $width = imagesx($image); $height = imagesy($image); // Create a new blank image with different dimensions $newWidth = 200; $newHeight = ($height / $width) * $newWidth; $newImage = imagecreate($newWidth, $newHeight); // Resize the image imagecopyresized($newImage, $image, 0, 0, 0, 0, $newWidth, $newHeight, $width, $height); // Output the resized image as a JPEG header('Content-Type: image/jpeg'); imagejpeg($newImage); // Clean up resources imagedestroy($image); imagedestroy($newImage); ?>
In this code, we load an existing image, create a new blank image with different dimensions, and then use imagecopyresized() to resize the image.
4.2. Generating Thumbnails
Creating thumbnails is a common use case for dynamic image generation. Here’s an example of generating a thumbnail image:
php <?php // Load an existing image $image = imagecreatefromjpeg('original.jpg'); // Get the current dimensions of the image $width = imagesx($image); $height = imagesy($image); // Calculate the thumbnail dimensions (e.g., 100x100 pixels) $thumbnailWidth = 100; $thumbnailHeight = 100; // Create a new blank image for the thumbnail $thumbnail = imagecreate($thumbnailWidth, $thumbnailHeight); // Resize and crop the image to fit the thumbnail size imagecopyresampled($thumbnail, $image, 0, 0, 0, 0, $thumbnailWidth, $thumbnailHeight, $width, $height); // Output the thumbnail as a JPEG header('Content-Type: image/jpeg'); imagejpeg($thumbnail); // Clean up resources imagedestroy($image); imagedestroy($thumbnail); ?>
In this code, we load an existing image, calculate the dimensions for the thumbnail, create a new blank image, and then use imagecopyresampled() to resize and crop the image to fit the thumbnail size.
4.3. Adding Watermarks
You can easily add watermarks to your images using GD Library. Here’s an example of adding a watermark to an image:
php <?php // Load the main image $image = imagecreatefromjpeg('main.jpg'); // Load the watermark image with transparency $watermark = imagecreatefrompng('watermark.png'); // Get the dimensions of the main image $mainWidth = imagesx($image); $mainHeight = imagesy($image); // Get the dimensions of the watermark image $watermarkWidth = imagesx($watermark); $watermarkHeight = imagesy($watermark); // Calculate the position for the watermark (e.g., bottom-right corner) $positionX = $mainWidth - $watermarkWidth - 10; $positionY = $mainHeight - $watermarkHeight - 10; // Overlay the watermark onto the main image imagecopy($image, $watermark, $positionX, $positionY, 0, 0, $watermarkWidth, $watermarkHeight); // Output the image with the watermark as a JPEG header('Content-Type: image/jpeg'); imagejpeg($image); // Clean up resources imagedestroy($image); imagedestroy($watermark); ?>
In this code, we load both the main image and the watermark image, calculate the position for the watermark (e.g., bottom-right corner), and then overlay the watermark onto the main image using imagecopy().
5. Best Practices
5.1. Memory Management
When working with GD Library, it’s important to manage memory properly to avoid memory leaks. Always use imagedestroy() to free up memory when you’re done with an image resource. Failing to do so can lead to memory exhaustion, especially when generating multiple images in a single script.
5.2. Error Handling
GD Library functions may return false if an error occurs. It’s a good practice to check the return values of GD functions and handle errors gracefully. You can use imagedestroy() to free resources and log errors if necessary.
Conclusion
In this guide, we’ve explored PHP’s imagecreate() function and the powerful capabilities of the GD Library. You’ve learned how to create blank images, allocate colors, draw shapes and text, manipulate images, generate thumbnails, add watermarks, and follow best practices for memory management and error handling.
With these skills, you can enhance your web applications by dynamically generating and manipulating images to meet your specific needs. GD Library provides a wide range of tools for creating visually appealing and interactive web content, making it a valuable asset in your web development toolkit.
Start experimenting with imagecreate() and GD Library to unlock new possibilities for your web projects. Whether you’re building e-commerce websites, data visualization tools, or simply adding a personal touch to your images, GD Library’s capabilities are at your disposal to bring your creative ideas to life. Happy coding!
Table of Contents
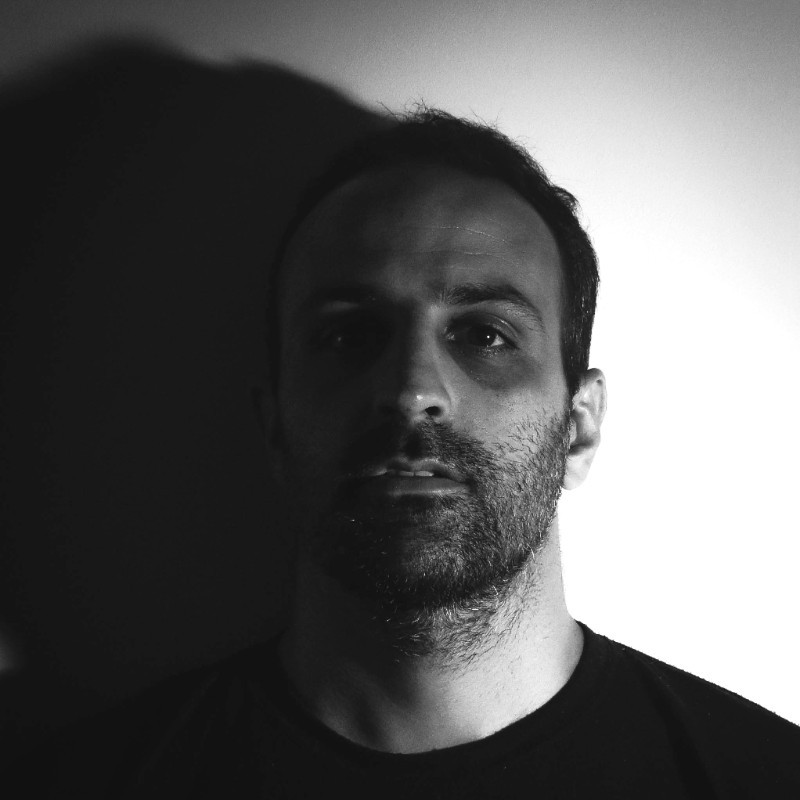
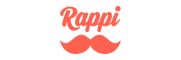