PHP Q & A
How to implement caching?
Implementing caching in PHP is an effective technique to improve the performance and reduce the load on your web server by storing frequently used data in a temporary storage system. Caching helps serve content faster to users and reduces the need for repeated data processing. Here’s a guide on how to implement caching in PHP:
- Choose a Caching Mechanism: PHP offers various caching mechanisms, such as Memcached, Redis, and built-in mechanisms like PHP’s APCu or file-based caching. Select the one that suits your project’s needs and infrastructure.
- Install and Configure: If you choose a server-based caching system like Memcached or Redis, you’ll need to install and configure it on your server. For file-based caching, create a directory with appropriate permissions to store cache files.
- Identify Cacheable Content: Determine which parts of your application can benefit from caching. These can include database queries, rendered HTML, API responses, or any expensive computations.
- Implement Caching Logic: Wrap the code that generates or retrieves the data you want to cache with logic to check if the data is already cached. If it is, retrieve the cached data; otherwise, generate it and store it in the cache.
```php $key = 'unique_cache_key'; $data = $cache->get($key); // Attempt to retrieve data from cache if ($data === false) { // Data not found in cache, generate it and store it $data = fetchDataFromDatabaseOrExpensiveOperation(); $cache->set($key, $data, $ttl); // Set a time-to-live (TTL) for the cache entry } // Use the $data in your application ```
- Set Expiry Time (TTL): When storing data in the cache, set a reasonable time-to-live (TTL) to ensure that cached data is refreshed periodically. This prevents serving outdated content.
- Cache Invalidation: Implement cache invalidation mechanisms to remove or update cached data when it becomes stale or outdated. This can be done manually or automatically based on certain events.
- Monitor and Optimize: Continuously monitor your cache’s performance and adjust caching strategies as needed. Over-caching or under-caching can impact your application’s performance.
- Handle Cache Failures: Implement error handling for cache failures to ensure that your application gracefully handles situations where the cache is unavailable or encounters issues.
Caching is a valuable tool for optimizing PHP applications, and it can significantly improve response times and reduce server load. However, it requires careful planning and management to ensure that cached data remains consistent and up-to-date.
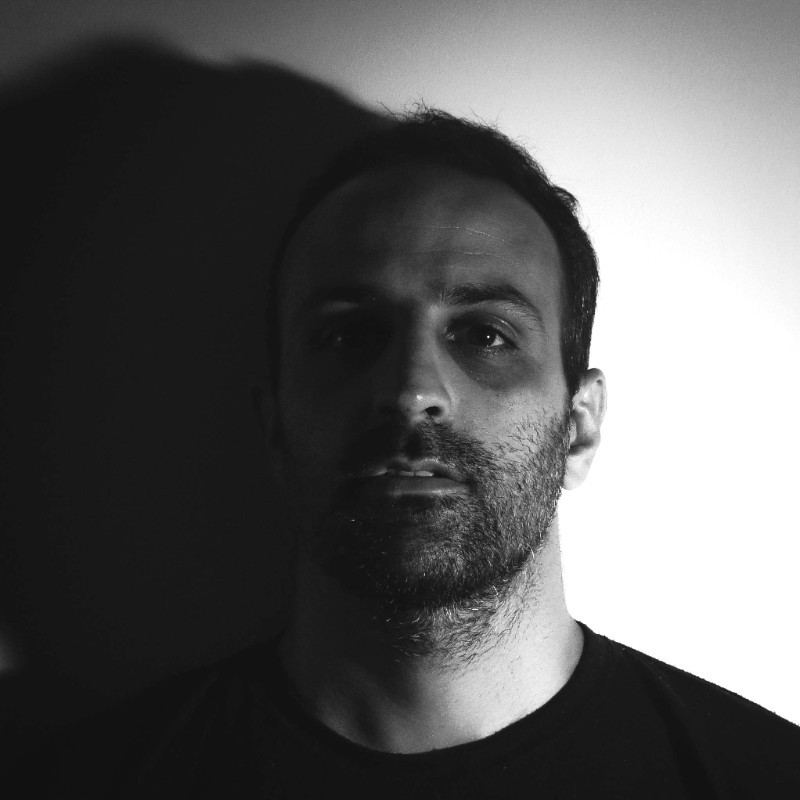
Previously at
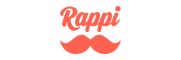
Full Stack Engineer with extensive experience in PHP development. Over 11 years of experience working with PHP, creating innovative solutions for various web applications and platforms.