Unraveling the Magic of PHP’s implode() Function
In the world of PHP, string manipulation is a fundamental task, and the implode() function is a versatile tool that simplifies it. This function allows developers to combine the elements of an array into a single string, making it invaluable for tasks ranging from creating comma-separated lists to generating dynamic content. In this blog, we will explore the workings of the implode() function, provide practical examples, and discuss its best practices.
What is the implode() Function?
The implode() function in PHP is used to concatenate array elements into a string. It takes two arguments: a separator and an array. The separator is a string that is placed between each element of the array in the resulting string.
Syntax
```php string implode ( string $separator , array $array ) ```
- $separator: The delimiter to place between each array element.
- $array: The array containing elements to join.
Basic Example
Here’s a simple example of how the implode() function works:
```php $fruits = ['apple', 'banana', 'cherry']; $fruitString = implode(', ', $fruits); echo $fruitString; // Output: apple, banana, cherry ```
In this example, the implode() function joins the elements of the $fruits array into a single string, with each fruit name separated by a comma and a space.
Practical Uses of implode()
The implode() function is highly useful in various scenarios. Let’s delve into some common use cases.
Creating a CSV Line
One of the most common uses of implode() is to generate a line for a CSV file. For instance, if you have an array of values representing a row of data, you can use implode() to create a CSV-formatted string.
```php $data = ['John Doe', 'johndoe@example.com', '123 Main St']; $csvLine = implode(',', $data); echo $csvLine; // Output: John Doe,johndoe@example.com,123 Main St ```
Generating a Query String
When constructing URLs with query parameters, implode() can help format the query string efficiently.
```php $params = ['name' => 'John', 'age' => '30', 'country' => 'USA']; $queryString = implode('&', array_map( fn($k, $v) => "$k=$v", array_keys($params), $params )); echo $queryString; // Output: name=John&age=30&country=USA ```
This example demonstrates how to concatenate key-value pairs into a query string format.
Combining Paths
implode() can also be used to build file paths or URL paths from an array of directory names or URL segments.
```php $directories = ['usr', 'local', 'bin']; $path = implode(DIRECTORY_SEPARATOR, $directories); echo $path; // Output: usr/local/bin (on Unix-like systems) ```
Best Practices and Considerations
While implode() is a powerful function, it’s important to use it wisely:
- Separator Choice: Choose a separator that does not appear in the array elements to avoid confusion.
- Data Sanitization: When working with user input, always sanitize and validate data to prevent security issues, especially in query strings.
- Readable Code: Use descriptive variable names and comments to make the code more understandable.
Handling Empty Arrays
When the array passed to implode() is empty, the function returns an empty string. This behavior should be handled appropriately in your code.
```php $emptyArray = []; $result = implode(', ', $emptyArray); echo $result; // Output: (an empty string) ```
Conclusion
The implode() function is an essential tool in a PHP developer’s toolkit, providing a simple yet powerful way to join array elements into a string. Whether you’re generating CSV files, query strings, or paths, understanding how to use implode() effectively can streamline your coding process and improve your code’s readability.
Further Reading
Table of Contents
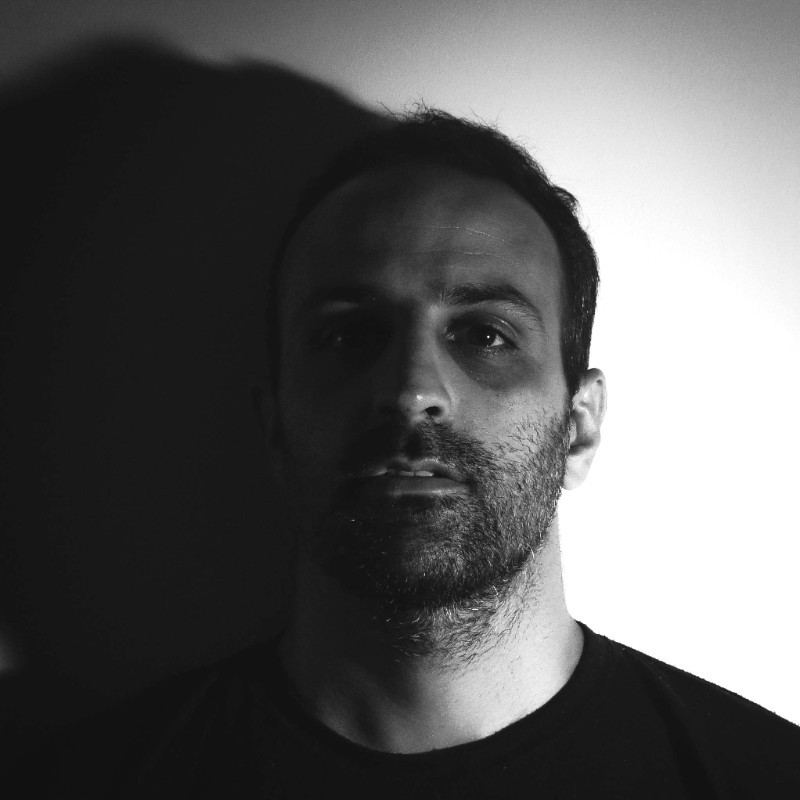
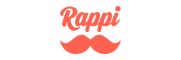