PHP’s in_array() Function: An In-Depth Guide
Arrays are an essential data structure in programming, allowing developers to store and organize multiple values under a single variable. In PHP, arrays are extensively used, and often, we need to determine whether a specific value exists within an array. This is where the in_array() function comes into play. In this guide, we’ll delve deep into the workings of the in_array() function, uncover its various parameters, use cases, and optimization techniques.
1. Understanding the Basics:
At its core, the in_array() function is used to check if a given value exists within an array. It returns true if the value is found and false if it’s not. This simple yet powerful function can save time and effort when dealing with arrays.
2. Syntax:
The basic syntax of the in_array() function is as follows:
php in_array($needle, $haystack, $strict);
Here:
- $needle is the value you want to search for.
- $haystack is the array in which you’re searching for the value.
- $strict (optional) is a boolean parameter that indicates whether the comparison should be strict (both type and value) or not. Default is false.
3. Examples:
Let’s dive into some examples to understand how the in_array() function works:
3.1. Basic Usage:
php $fruits = ["apple", "banana", "orange", "grape"]; $targetFruit = "banana"; if (in_array($targetFruit, $fruits)) { echo "$targetFruit is found in the array."; } else { echo "$targetFruit is not found in the array."; }
3.2. Using Strict Comparison:
php $numbers = [1, 2, 3, 4, 10]; $targetNumber = "2"; if (in_array($targetNumber, $numbers, true)) { echo "$targetNumber is found in the array."; } else { echo "$targetNumber is not found in the array."; }
4. Parameters in Detail:
4.1. Needle:
The $needle parameter is the value you’re searching for within the array. It can be of any data type: string, integer, float, boolean, or even an array. Keep in mind that if the $needle is an array, the function will always return false, regardless of the contents of the array.
4.2. Haystack:
The $haystack parameter represents the array in which you’re searching for the $needle. This is the array you want to check for the presence of the value. It’s crucial to provide the correct array in order to get accurate results.
4.3. Strict Comparison (Optional):
The $strict parameter is optional. When set to true, it enforces strict type checking. This means that not only the values need to match, but their data types must also match. By default, this parameter is set to false.
5. Use Cases and Practical Examples:
5.1. Checking for Membership:
The most common use case of in_array() is to check whether a specific value exists within an array. For instance, you can use it to validate user input against a list of allowed values:
php $validColors = ["red", "green", "blue", "yellow"]; $userColor = $_POST['color']; if (in_array($userColor, $validColors)) { echo "Valid color chosen: $userColor"; } else { echo "Invalid color!"; }
5.2. Removing Duplicates:
You can leverage the in_array() function to remove duplicate values from an array. By iterating through the array and appending non-duplicate values to a new array, you can achieve this efficiently:
php $numbersWithDuplicates = [1, 2, 3, 2, 4, 1, 5]; $uniqueNumbers = []; foreach ($numbersWithDuplicates as $number) { if (!in_array($number, $uniqueNumbers)) { $uniqueNumbers[] = $number; } } print_r($uniqueNumbers);
5.3. Associative Arrays and Values:
The in_array() function can also work with associative arrays, but it only searches through the values, not the keys. If you want to check for a value within the keys of an associative array, you’ll need to use array_key_exists().
php $userData = [ "username" => "john_doe", "email" => "john@example.com", "age" => 28 ]; $searchValue = "john@example.com"; if (in_array($searchValue, $userData)) { echo "$searchValue found in user data."; } else { echo "$searchValue not found in user data."; }
6. Optimizing Search with Array Keys:
While in_array() is a handy function, it might not be the most efficient choice for large arrays, especially if you’re going to perform multiple searches. In such cases, using associative arrays or transforming your array into a map (associative array) can significantly improve performance. This involves using the values as keys and assigning some constant or minimal value as the corresponding values.
php $bigArray = [/* a large array */]; $smallValueArray = array_flip($bigArray); $searchValue = "target_value"; if (isset($smallValueArray[$searchValue])) { echo "$searchValue found in the array."; } else { echo "$searchValue not found in the array."; }
Conclusion
In the world of PHP programming, efficient array operations are essential for optimal performance. The in_array() function provides a simple yet effective way to search for values within arrays. By understanding its parameters, syntax, and real-world use cases, you can harness the power of this function to enhance your coding efficiency and improve the performance of your applications. Whether you’re validating user input, removing duplicates, or optimizing array searches, the in_array() function is a valuable tool in your PHP toolkit.
Table of Contents
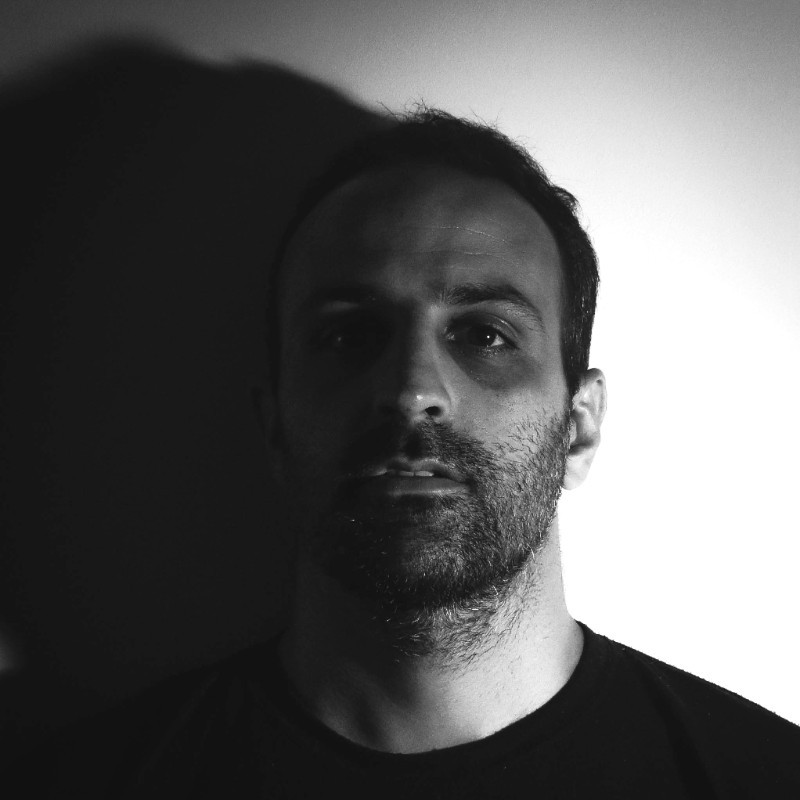
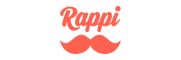