Understanding the Magic of PHP’s include() and require()
In the world of web development, PHP (Hypertext Preprocessor) stands as a robust and versatile scripting language, powering a significant portion of the web. One of its most powerful features is the ability to include external files using the include() and require() functions. These functions might appear simple on the surface, but they hold incredible potential for enhancing code organization, modularity, and reusability. In this guide, we’ll delve into the magic of include() and require(), uncover their differences, and explore best practices for utilizing them effectively.
1. Introduction to include() and require()
Imagine building a complex web application. As your codebase grows, maintaining and managing it can become overwhelming. This is where the PHP functions include() and require() come to your rescue. These functions allow you to split your code into smaller, more manageable chunks stored in separate files.
Both include() and require() serve the same purpose: they insert the content of an external file into the current script. This is immensely beneficial for breaking down a large codebase into smaller, more organized components.
2. The Basics of Including Files
2.1. Using include()
The include() function is a versatile tool for combining files. If the included file is not found, PHP generates a warning and continues executing the script.
php // index.php echo "This is the main file."; include("header.php"); echo "Page content."; include("footer.php");
2.2. Using require()
On the other hand, the require() function is more strict. If the specified file is not found, it will halt script execution and generate a fatal error.
php // index.php echo "This is the main file."; require("header.php"); echo "Page content."; require("footer.php");
In situations where the included file is crucial for the functionality of your application, require() is a safer choice. It ensures that the necessary components are present before proceeding.
3. Handling File Inclusion Failures
Understanding how to handle file inclusion failures is crucial for creating robust applications. While include() merely generates a warning and continues script execution, require() raises a fatal error, stopping the script in its tracks.
To mitigate the risks associated with require(), you can use require_once() and include_once(). These functions ensure that a file is included only once, even if the inclusion statement appears multiple times in your code.
php // header.php echo "Header content."; // index.php require_once("header.php"); require_once("header.php"); // This won't cause an error. echo "Page content.";
4. Practical Use Cases
4.1. Template Separation
When building web applications, separating your code into distinct templates is essential. Using include() or require() allows you to compartmentalize your header, footer, sidebar, and other common components into separate files. This makes your codebase more organized and easier to maintain.
4.2. Modular Code Design
Large-scale applications often consist of multiple modules or features. With file inclusion, you can create a modular architecture by placing each module’s code in its own file. This approach simplifies collaboration among developers and makes it easier to extend or modify specific parts of the application.
4.3. Reusing Code Snippets
Code reusability is a hallmark of efficient programming. With include() and require(), you can create libraries of reusable code snippets. Imagine you have a file containing functions that calculate complex mathematical formulas. By including this file in multiple projects, you ensure consistent and accurate computations without duplicating code.
5. Best Practices for Using include() and require()
5.1. Conditional Inclusion
Sometimes, you may want to include a file only if a certain condition is met. Utilize PHP’s conditional statements to achieve this.
php if ($isAdmin) { require("admin_panel.php"); }
5.2. Using Paths Wisely
PHP supports different types of paths when including files, such as absolute paths and relative paths. Choose the appropriate path type based on your project’s structure to ensure smooth file inclusion.
php // Using an absolute path include("/var/www/html/includes/header.php"); // Using a relative path include("../includes/header.php");
5.3. Minimizing Redundancy
While splitting code into smaller files is beneficial, be mindful not to overcomplicate your application with excessive file inclusions. Strive for a balance between modularity and simplicity.
6. Performance Considerations
File inclusion might raise concerns about performance. Including numerous files could lead to additional I/O operations, impacting the overall speed of your application. However, modern PHP engines are optimized to mitigate this impact. If performance becomes a concern, consider using techniques like opcode caching to improve execution speed.
Conclusion
In the dynamic realm of web development, maintaining clean, organized, and efficient code is paramount. PHP’s include() and require() functions provide a powerful mechanism to achieve these goals. By embracing these functions and following best practices, you can create modular, reusable, and maintainable codebases, simplifying the development process and enhancing the overall quality of your applications. So go ahead, harness the magic of include() and require(), and unlock a world of possibilities in your PHP projects.
Table of Contents
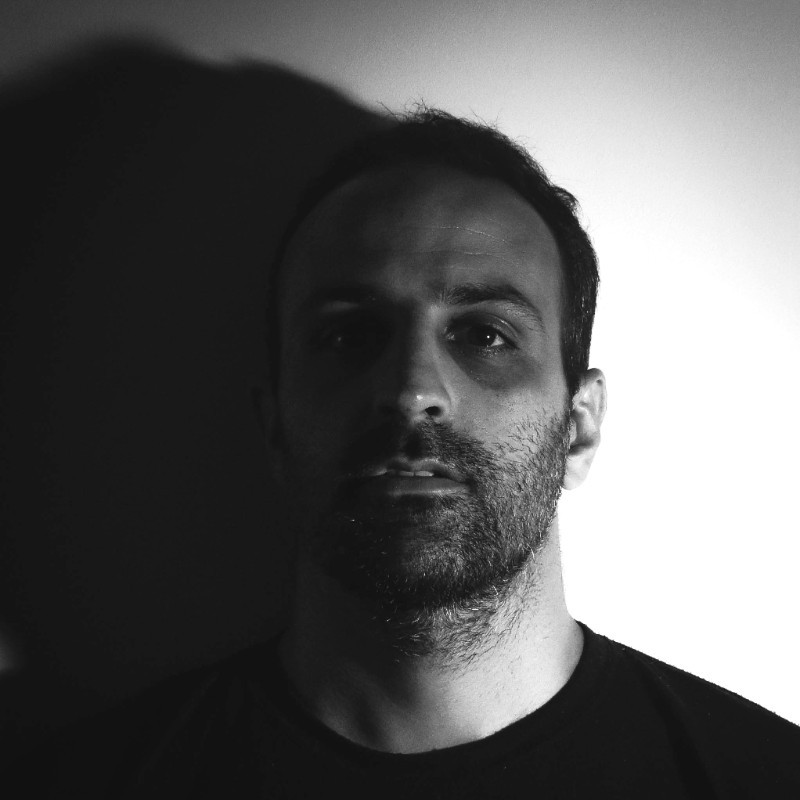
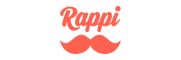