PHP’s PDO: Interacting with Databases the Secure Way
In the world of web development, database interactions are a fundamental aspect of building dynamic and data-driven applications. PHP, as one of the most popular server-side scripting languages, offers various approaches to connect and communicate with databases. However, when it comes to handling sensitive data, security becomes a primary concern. That’s where PHP’s PDO (PHP Data Objects) extension comes into play. PDO provides a robust and secure way to interact with databases, protecting applications from potential threats such as SQL injection attacks and other vulnerabilities.
In this blog post, we’ll delve into the world of PHP’s PDO, exploring its advantages over traditional database connection methods, understanding its secure features, and examining best practices for database interactions to ensure a safer and more reliable web application.
1. Why Choose PDO?
Traditionally, PHP developers have used functions like mysqli or mysql to interact with databases. While these functions are functional, they have some limitations in terms of portability, maintainability, and security. PDO, on the other hand, offers several compelling reasons to use it as the preferred method for database interactions:
- Database Agnostic: PDO is database agnostic, meaning it supports a wide range of database management systems, including MySQL, PostgreSQL, SQLite, Oracle, and more. This portability allows developers to switch between database systems without rewriting the entire codebase, making it more scalable and future-proof.
- Prepared Statements: One of the critical features of PDO is the ability to use prepared statements. Prepared statements separate SQL code from user-provided data, significantly reducing the risk of SQL injection attacks. This feature automatically escapes input data, preventing malicious queries from being executed.
- Error Handling: PDO provides consistent and easy-to-handle error reporting, which is crucial for debugging and maintaining the application. It allows developers to set error handling modes, making it simpler to catch and handle exceptions gracefully.
- Transaction Support: PDO supports database transactions, ensuring data integrity by allowing developers to perform multiple database operations as a single unit. This helps maintain the consistency of data, and in case of an error, it allows for rolling back changes, preserving the database’s integrity.
- Object-Oriented Interface: PDO offers an object-oriented interface, making database interactions more intuitive and readable. The use of objects and classes enhances code organization and readability, making it easier for developers to collaborate and maintain the codebase.
2. Connecting to the Database
Let’s start by looking at how to establish a connection to a database using PDO. Before proceeding, ensure that PDO is enabled in your PHP configuration.
php <?php // Database configuration $host = 'localhost'; $db_name = 'my_database'; $username = 'db_user'; $password = 'db_password'; try { // Create a new PDO instance $pdo = new PDO("mysql:host=$host;dbname=$db_name", $username, $password); // Set PDO error mode to exception $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); echo "Connected successfully!"; } catch (PDOException $e) { // Handle connection errors echo "Connection failed: " . $e->getMessage(); } ?>
In the above code snippet, we use the new PDO constructor to create a new PDO instance and pass in the necessary database connection parameters. The setAttribute method is used to set the error handling mode to exceptions, which means any error will throw a PDOException. This allows us to handle errors gracefully and avoid exposing sensitive information to end-users.
3. Executing Queries with PDO
Once the connection is established, we can perform database queries using PDO. Let’s look at some common query scenarios.
3.1. SELECT Query
To execute a SELECT query and fetch data from the database, we can use the query method along with fetch or fetchAll to retrieve the results.
php <?php try { // Assume $pdo is the PDO instance from the previous example // SELECT query $query = "SELECT * FROM users"; $statement = $pdo->query($query); // Fetch all rows as an associative array $rows = $statement->fetchAll(PDO::FETCH_ASSOC); // Process the data foreach ($rows as $row) { echo "Username: " . $row['username'] . ", Email: " . $row['email'] . "<br>"; } } catch (PDOException $e) { // Handle query errors echo "Query failed: " . $e->getMessage(); } ?>
In this example, we execute a SELECT query to retrieve all user records from the users table. The fetchAll method fetches all rows as an associative array, which we can then process and display.
3.2. INSERT Query
To insert data into the database, we need to use prepared statements to prevent SQL injection attacks.
php <?php try { // Assume $pdo is the PDO instance from the previous example // Sample data $username = "john_doe"; $email = "john.doe@example.com"; $password = password_hash("my_password", PASSWORD_DEFAULT); // INSERT query with a prepared statement $query = "INSERT INTO users (username, email, password) VALUES (:username, :email, :password)"; $statement = $pdo->prepare($query); $statement->bindParam(':username', $username); $statement->bindParam(':email', $email); $statement->bindParam(':password', $password); // Execute the prepared statement $statement->execute(); echo "Data inserted successfully!"; } catch (PDOException $e) { // Handle query errors echo "Insert failed: " . $e->getMessage(); } ?>
In this example, we use a prepared statement to safely insert data into the users table. We bind parameters to placeholders (e.g., :username, :email, :password) and execute the statement. PDO takes care of escaping the data and preventing SQL injection.
3.3. UPDATE Query
Updating records is similar to inserting data, where we use prepared statements to ensure secure updates.
php <?php try { // Assume $pdo is the PDO instance from the previous example // Sample data $user_id = 1; $new_email = "new.email@example.com"; // UPDATE query with a prepared statement $query = "UPDATE users SET email = :email WHERE id = :user_id"; $statement = $pdo->prepare($query); $statement->bindParam(':email', $new_email); $statement->bindParam(':user_id', $user_id); // Execute the prepared statement $statement->execute(); echo "Data updated successfully!"; } catch (PDOException $e) { // Handle query errors echo "Update failed: " . $e->getMessage(); } ?>
In this example, we use a prepared statement to update the email address of a user with the ID of 1. As with the previous examples, prepared statements protect against potential SQL injection vulnerabilities.
3.4. DELETE Query
Similarly, deleting records requires using prepared statements to ensure secure deletion.
php <?php try { // Assume $pdo is the PDO instance from the previous example // Sample data $user_id = 1; // DELETE query with a prepared statement $query = "DELETE FROM users WHERE id = :user_id"; $statement = $pdo->prepare($query); $statement->bindParam(':user_id', $user_id); // Execute the prepared statement $statement->execute(); echo "Data deleted successfully!"; } catch (PDOException $e) { // Handle query errors echo "Deletion failed: " . $e->getMessage(); } ?>
In this example, we use a prepared statement to delete a user record with the ID of 1. By utilizing prepared statements, we eliminate the possibility of SQL injection attacks.
Conclusion
Interacting with databases securely is a crucial aspect of building robust and reliable web applications. PHP’s PDO extension provides an excellent solution for achieving this goal. By leveraging its database agnostic nature, prepared statements, error handling capabilities, and transaction support, developers can enhance the security and performance of their database interactions.
In this blog post, we explored the key features of PDO and learned how to connect to a database, execute common queries, and safeguard our applications against SQL injection attacks. By following these best practices and adopting PDO as our preferred method for database interactions, we can build more secure and scalable PHP applications.
Remember that security is an ongoing concern, and it is essential to keep up to date with the latest security practices and vulnerabilities. By staying vigilant and continuously improving our coding practices, we can build a safer online environment for our users and protect sensitive data from potential threats.
Start using PHP’s PDO today and take your database interactions to the next level of security and reliability!
Table of Contents
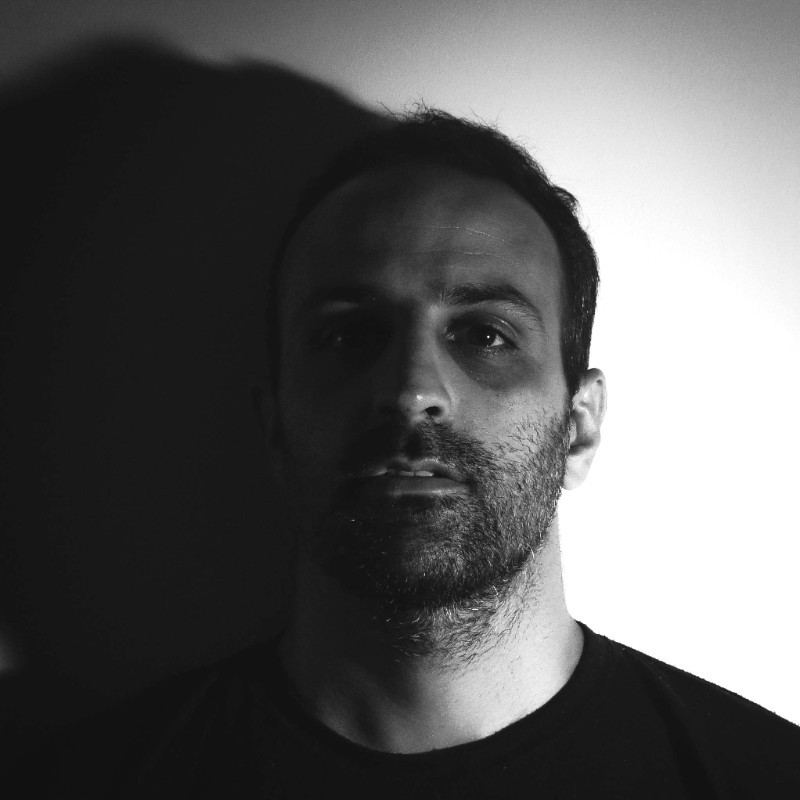
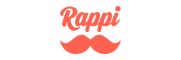