Working with PHP’s is_array() Function: A Practical Guide
When working with PHP, it’s essential to have a solid understanding of the built-in functions to streamline your development process and write more efficient code. One such function is is_array(), which allows you to check if a variable is an array or not. In this practical guide, we will delve into the ins and outs of PHP’s is_array() function, providing you with everything you need to know to leverage it effectively in your projects.
Table of Contents
1. Understanding the Basics
1.1. What is the is_array() Function?
The is_array() function is a fundamental part of PHP’s toolbox for working with arrays. It serves a simple yet crucial purpose: to determine whether a given variable is an array or not. This function returns true if the variable is an array and false otherwise.
Here’s the basic syntax of the is_array() function:
php bool is_array(mixed $variable)
- mixed $variable: The variable you want to check.
1.2. Using is_array() in Your Code
Let’s start with a straightforward example of how to use the is_array() function:
php $myArray = [1, 2, 3]; if (is_array($myArray)) { echo "Yes, it's an array!"; } else { echo "No, it's not an array."; }
In this example, we create an array $myArray and then use is_array() to check if it’s indeed an array. The condition evaluates to true, so the message “Yes, it’s an array!” will be displayed.
1.3. Handling Non-Array Variables
The is_array() function can also handle non-array variables. Let’s take a look:
php $myVariable = 42; if (is_array($myVariable)) { echo "Yes, it's an array!"; } else { echo "No, it's not an array."; }
In this case, the variable $myVariable is not an array, so the condition evaluates to false, and the message “No, it’s not an array.” will be displayed.
2. Practical Applications
2.1. Input Validation
One of the most common use cases for is_array() is input validation, especially when dealing with form data in web applications. By using is_array(), you can check if the input you receive is in the expected format. For example, you can use it to ensure that an uploaded file is an array of files:
php if (is_array($_FILES['user_file']['name'])) { foreach ($_FILES['user_file']['name'] as $fileName) { // Process each file } } else { // Handle the case where only one file is uploaded $fileName = $_FILES['user_file']['name']; // Process the single file }
In this snippet, we use is_array() to determine whether multiple files have been uploaded or just a single file. This allows us to handle both cases appropriately.
2.2. Iterating Over Arrays Safely
When working with arrays in PHP, it’s a good practice to check if a variable is an array before attempting to iterate over it. This prevents potential errors and avoids unexpected behavior in your code. Here’s an example:
php $data = getData(); // A function that may return an array or something else if (is_array($data)) { foreach ($data as $item) { // Process each item in the array } } else { // Handle the case where $data is not an array }
By using is_array() in this scenario, you ensure that the code inside the foreach loop only executes when $data is indeed an array, preventing errors when it’s not.
2.3. Handling Dynamic Data Structures
In some cases, you may work with dynamic data structures where a variable can be an array, an object, or something else entirely. To handle such scenarios gracefully, you can use is_array() in combination with other type-checking functions like is_object(). Here’s an example:
php $data = getData(); // A function that may return an array, an object, or something else if (is_array($data)) { foreach ($data as $item) { // Process each item in the array } } elseif (is_object($data)) { // Handle the case where $data is an object } else { // Handle other data types }
This code snippet demonstrates how to handle different data types returned by the getData() function gracefully.
3. Advanced Techniques
3.1. Checking for Associative Arrays
By default, is_array() considers both indexed and associative arrays as arrays. However, if you want to specifically check for an associative array (an array with string keys), you can use a custom function like this:
php function is_associative_array($arr) { return is_array($arr) && count(array_filter(array_keys($arr), 'is_string')) > 0; } $myArray = ['name' => 'John', 'age' => 30]; if (is_associative_array($myArray)) { echo "Yes, it's an associative array!"; } else { echo "No, it's not an associative array."; }
In this example, the is_associative_array() function checks if an array contains at least one string key, identifying it as an associative array.
3.2. Combining Type Checking
You can also combine is_array() with other type-checking functions to create more specific checks. For instance, you might want to check if a variable is an array of objects:
php function is_array_of_objects($arr) { return is_array($arr) && count(array_filter($arr, 'is_object')) === count($arr); } $myArray = [new MyClass(), new AnotherClass()]; if (is_array_of_objects($myArray)) { echo "Yes, it's an array of objects!"; } else { echo "No, it's not an array of objects."; }
In this case, the is_array_of_objects() function checks if all elements in the array are objects.
4. Common Pitfalls
4.1. Treating Strings as Arrays
One common mistake is treating strings as arrays. PHP allows you to access individual characters in a string as if they were elements of an array. While this can be useful, it’s essential to remember that a string is not an array, and using is_array() on a string will yield false.
php $text = "Hello, world!"; if (is_array($text)) { // This code will not execute because $text is not an array foreach ($text as $char) { // Process each character } } else { echo "No, it's not an array."; }
In this example, $text is a string, not an array, so the code inside the if block will not execute.
4.2. Confusing is_array() with empty()
Another common mistake is confusing the is_array() function with the empty() function. While is_array() checks if a variable is an array, empty() checks if a variable is empty. These are two distinct checks:
php $myArray = []; if (is_array($myArray)) { echo "It's an array."; } if (empty($myArray)) { echo "It's empty."; }
In this example, both conditions may evaluate to true because $myArray is both an empty array and an array.
5. Real-World Examples
Example 1: Filtering User Input
Suppose you are building a web application that allows users to search for products. Users can input search queries in different ways, such as using a single keyword or specifying multiple filters. To handle these variations, you can use is_array() to check the user’s input:
php $userInput = $_GET['search']; if (is_array($userInput)) { // Handle advanced filtering options } else { // Perform a basic keyword search }
By checking if $_GET[‘search’] is an array, you can determine whether the user is applying advanced filters or performing a basic search.
Example 2: Data Transformation
Consider a scenario where you receive data from an external source, such as an API. The data may come in various formats, including arrays and objects. To process this data uniformly, you can use is_array() to check its type:
php $data = fetchDataFromAPI(); if (is_array($data)) { // Process array data } elseif (is_object($data)) { // Convert object data to an array and then process it $data = json_decode(json_encode($data), true); // Process array data } else { // Handle other data types or errors }
In this example, you ensure that regardless of whether the data is initially an array or an object, it is processed consistently as an array.
Conclusion
The is_array() function in PHP is a versatile tool that helps you make informed decisions about the type of data you’re working with. Whether you’re validating user input, processing dynamic data structures, or creating custom type checks, understanding how to use is_array() effectively is essential for writing robust and error-free code.
As you continue to explore PHP and build more complex applications, keep in mind that is_array() is just one of many useful functions at your disposal. By mastering these functions and incorporating them into your development toolkit, you can become a more proficient PHP developer, capable of tackling a wide range of programming challenges with confidence.
Table of Contents
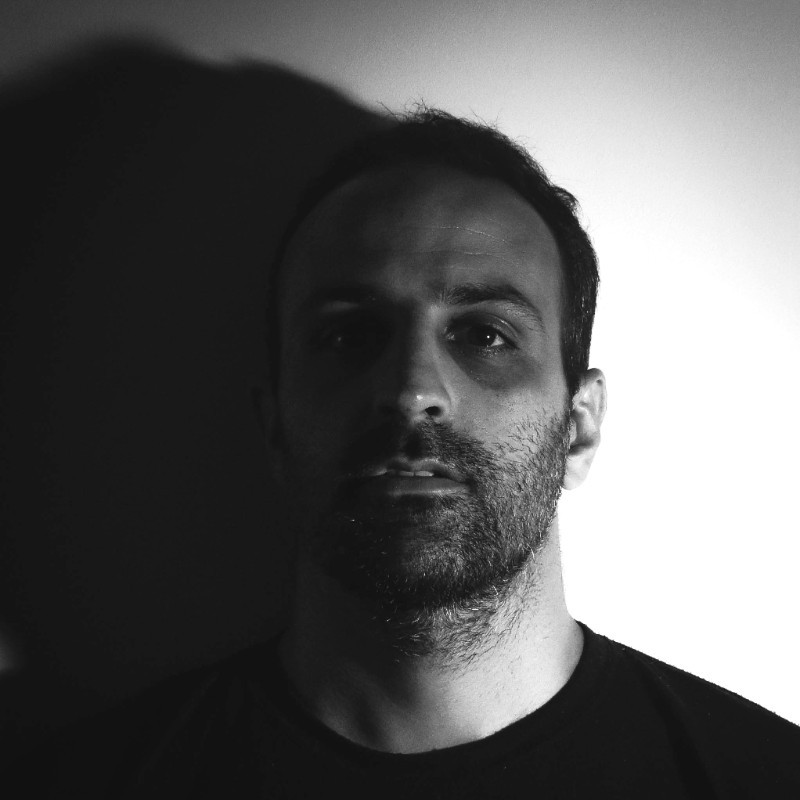
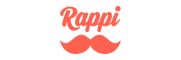