Getting the Most Out of PHP’s is_null() Function
PHP, a popular server-side scripting language, offers a multitude of functions and tools to make your coding experience smoother and more efficient. One such function that can significantly contribute to writing robust and clean code is is_null(). In this comprehensive guide, we’ll delve into the is_null() function, its various use cases, and how to leverage it effectively in your PHP projects.
Table of Contents
1. Understanding the Basics of is_null()
Before we dive into the practical applications of is_null(), let’s start with the fundamentals. The is_null() function is used to determine whether a variable is null or not. It returns true if the variable is null and false if it is not. This function can be invaluable in a wide range of scenarios, from error handling to data validation.
1.1. Syntax
Here is the basic syntax of the is_null() function:
php bool is_null ( mixed $var )
- mixed $var: The variable to be checked for null.
Now, let’s explore some real-world scenarios where is_null() can be a lifesaver.
2. Validating User Input
When building web applications, user input validation is crucial to ensure data integrity and security. In PHP, you often receive user inputs through forms. Using is_null() can help you verify if a user has provided the required data before processing it.
Let’s take a look at an example where we expect the user to enter their name:
php $name = $_POST['name']; if (is_null($name)) { echo "Please enter your name."; } else { // Process the user's input }
In this code snippet, we first retrieve the user’s input from a form. Then, we use is_null() to check if the $name variable is null. If it is, we display a message asking the user to enter their name; otherwise, we proceed with processing the input.
3. Handling Database Results
Database queries are a common part of web development, and dealing with query results is essential. Sometimes, a query may not return any data, which means the result is null. To handle such cases gracefully, you can utilize is_null().
Consider a scenario where you fetch a user’s profile from a database:
php $userProfile = fetchUserProfile($userId); if (is_null($userProfile)) { echo "User not found."; } else { // Display the user's profile }
In this example, we use is_null() to check if the $userProfile variable contains a valid user profile. If it’s null, we display a message indicating that the user was not found in the database.
4. Avoiding Undefined Variable Errors
Undefined variables can lead to runtime errors in your PHP code. To prevent such errors and improve code stability, you can employ is_null().
Let’s say you have a script that depends on a configuration value:
php $configValue = getConfigValue('some_key'); if (!is_null($configValue)) { // Use the configuration value } else { // Handle the absence of the configuration value }
In this snippet, is_null() helps us ensure that we only access and use the $configValue variable if it exists. If it’s null, we can implement a fallback or error-handling mechanism.
5. Advanced Usage: Checking for Null in Objects
So far, we’ve looked at how is_null() can be used with variables. However, PHP’s is_null() function can also be applied to object properties. Let’s explore an example where you want to check if a property of an object is null.
Suppose you have a Product class:
php class Product { public $name; public $price; public function __construct($name, $price) { $this->name = $name; $this->price = $price; } }
Now, you create an instance of the Product class:
php $product = new Product('Widget', 19.99);
To check if the price property of the $product object is null, you can use is_null():
php if (is_null($product->price)) { echo "Price is not available for this product."; } else { echo "Price: $" . $product->price; }
In this example, we use is_null() to examine the $product->price property, allowing us to display a relevant message based on its null or non-null status.
6. Best Practices with is_null()
While is_null() is a valuable tool, it’s essential to use it judiciously to maintain code clarity and readability. Here are some best practices to keep in mind:
6.1. Combine with Conditional Statements
is_null() is often used in conjunction with conditional statements like if and else to make your code more expressive and clear. Always provide meaningful messages or actions in each branch of the condition to enhance code readability.
6.2. Use It Where It Makes Sense
Avoid using is_null() unnecessarily. Only apply it to variables or properties where it’s essential to check for null values. Overusing it can lead to code clutter and decreased readability.
6.3. Be Consistent with Error Handling
When using is_null() for error handling, be consistent in how you handle errors across your codebase. Establish a standard approach to error messages and responses to maintain a coherent user experience.
6.4. Document Your Intent
If you use is_null() in a non-standard way or for a specific reason, document it in comments to clarify your code’s intent for other developers who may work on the project.
Conclusion
The is_null() function is a versatile tool in PHP that helps you determine whether a variable or object property is null. By incorporating it into your code, you can enhance user input validation, gracefully handle database queries, and prevent undefined variable errors. However, it’s crucial to use is_null() judiciously and in alignment with best practices to maintain code clarity and readability.
As you continue to explore PHP and its features, remember that mastering functions like is_null() is just one step toward becoming a proficient PHP developer. Practice, experimentation, and ongoing learning are the keys to success in the dynamic world of web development. So, keep coding, keep learning, and keep building amazing things with PHP!
Table of Contents
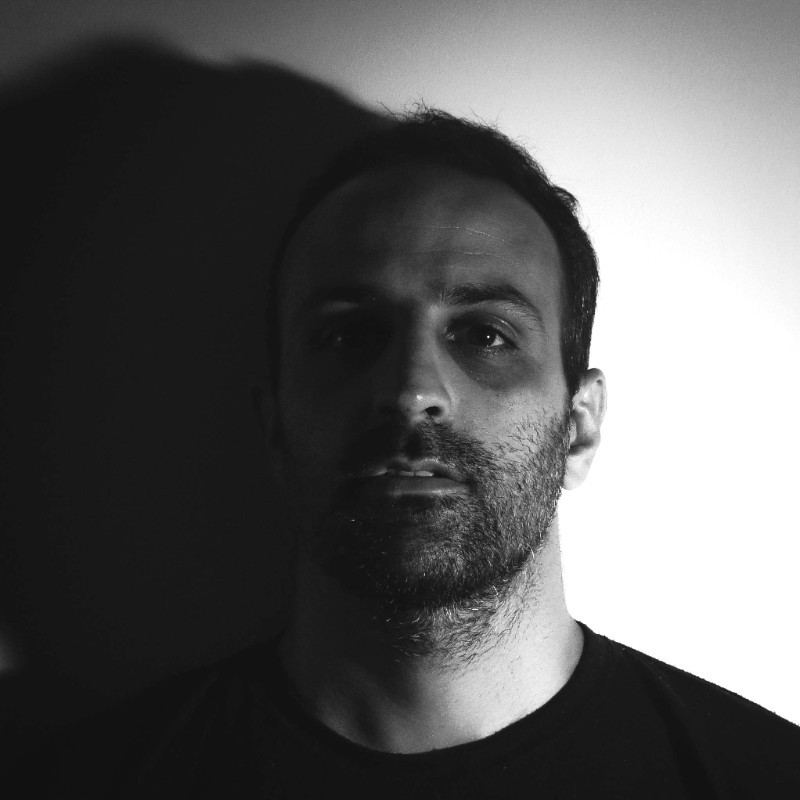
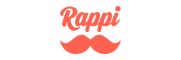