Leveraging PHP’s is_string() Function: A Practical Guide
PHP is a versatile and widely-used scripting language, known for its ability to handle various data types. One common task in PHP development is checking whether a variable is of a specific type. This is where PHP’s is_string() function comes into play. In this comprehensive guide, we will explore the is_string() function, its applications, benefits, and provide practical code examples to help you leverage its power effectively.
Table of Contents
1. Understanding the is_string() Function
Before diving into practical applications, let’s first understand what the is_string() function does. In PHP, this function is used to check whether a given variable is a string or not. It returns true if the variable is a string and false otherwise. Here’s the basic syntax:
php bool is_string ( mixed $var )
- mixed $var: The variable you want to check.
2. Advantages of Using is_string()
The is_string() function offers several advantages when it comes to data validation and handling in PHP:
2.1. Simplified Type Checking
When dealing with user inputs, data from external sources, or even data fetched from a database, it’s crucial to ensure that the expected data type is maintained. The is_string() function simplifies this type-checking process by providing a clear and concise way to determine if a variable is a string.
2.2. Preventing Unexpected Errors
Using is_string() can help you prevent unexpected errors in your code. For example, if you expect a variable to contain a string but it’s actually an array or an integer, you can catch this issue early and handle it gracefully rather than encountering runtime errors.
2.3. Improved Code Readability
Explicitly checking the data type of a variable with is_string() makes your code more readable and self-explanatory. When someone else reads your code or when you revisit it later, it’s immediately clear what type of data is expected at that point in the program.
2.4. Avoiding Type Juggling Pitfalls
PHP is known for its loose type system, which means it can perform implicit type conversions. While this flexibility can be useful, it can also lead to unexpected behavior. is_string() allows you to explicitly check the data type, reducing the chances of type juggling pitfalls.
3. Practical Applications of is_string()
Now that we understand the benefits of using the is_string() function, let’s explore some practical scenarios where it can be invaluable.
3.1. Validating User Input
One of the most common use cases for is_string() is validating user input. When your PHP application expects a user to enter a string, you can use this function to ensure that the input is indeed a string before processing it.
php $userInput = $_POST['username']; if (is_string($userInput)) { // Process the user input as a string } else { // Handle the case where the input is not a string echo "Invalid input. Please enter a valid username."; }
This code snippet checks if the $_POST[‘username’] variable is a string before processing it further. If it’s not a string, an error message is displayed to the user.
3.2. Working with JSON Data
In modern web development, working with JSON data is commonplace. When you decode JSON data using functions like json_decode(), you may need to ensure that specific values within the decoded data are strings.
php $jsonData = '{"name": "John", "age": 30}'; $data = json_decode($jsonData, true); if (is_string($data['name'])) { // The 'name' field is a string, continue processing } else { // Handle the case where 'name' is not a string echo "Invalid data format: 'name' should be a string."; }
Here, we check if the ‘name’ field in the JSON data is a string before proceeding with further processing.
3.3. Handling Database Results
When fetching data from a database, the retrieved values can be of various data types. To ensure that you’re working with strings as expected, you can use is_string().
php // Assume $row is a result row fetched from a database if (is_string($row['email'])) { // $row['email'] is a string, proceed with email-related operations } else { // Handle the case where 'email' is not a string echo "Invalid data format: 'email' should be a string."; }
This code snippet verifies that the ’email’ field retrieved from the database is a string before using it in email-related operations.
4. Combining is_string() with Other Functions
In many cases, you might need to combine is_string() with other functions to perform more complex type checking or data validation.
4.1. Checking for Empty Strings
To check if a variable is not only a string but also not empty, you can use is_string() in combination with empty() or strlen().
php $userInput = $_POST['address']; if (is_string($userInput) && !empty($userInput)) { // Process the user's address as a non-empty string } else { // Handle the case where the input is not a non-empty string echo "Invalid input. Please enter a valid address."; }
This code checks if the $_POST[‘address’] variable is both a string and not empty before processing it.
4.2. Validating Email Addresses
When validating email addresses, you can combine is_string() with regular expressions or dedicated email validation libraries for more precise validation.
php $email = $_POST['email']; if (is_string($email) && filter_var($email, FILTER_VALIDATE_EMAIL)) { // Process the email address as a valid string } else { // Handle the case where the input is not a valid email address echo "Invalid email address. Please enter a valid email."; }
In this example, is_string() checks if the input is a string, and filter_var() checks if it’s a valid email address.
5. Handling Non-String Values
In situations where a variable might not be a string, it’s essential to have a fallback plan or handle non-string values gracefully. Here are some approaches to consider:
5.1. Type Conversion
If the variable can be safely converted to a string, you can use type casting or functions like strval() to convert it.
php $value = 42; // an integer if (is_string($value)) { // Process the string value } else { // Convert non-string value to a string $stringValue = strval($value); // Process the $stringValue as a string }
In this example, if $value is not initially a string, it’s converted to a string using strval() before further processing.
5.2. Providing Default Values
Another approach is to provide default values when the variable is not a string.
php $input = $_POST['input']; if (is_string($input)) { // Process the string input } else { // Use a default value or display an error $defaultValue = "Default Value"; echo "Invalid input. Using default: $defaultValue"; }
Here, if $_POST[‘input’] is not a string, the code uses a default value or displays an error message.
5.3. Logging Errors
In some cases, you might want to log errors or exceptions when non-string values are encountered.
php $userInput = $_POST['input']; if (is_string($userInput)) { // Process the string input } else { // Log an error error_log("Invalid input: " . print_r($userInput, true)); // Handle the error gracefully echo "An error occurred. Please try again later."; }
This code logs the invalid input for debugging purposes and provides a user-friendly error message.
Conclusion
The is_string() function in PHP is a valuable tool for ensuring that your variables contain string data when expected. By using is_string(), you can simplify type checking, prevent unexpected errors, and improve code readability. It’s particularly useful when dealing with user input, JSON data, or database results.
Remember that while is_string() is a powerful function, it’s essential to handle non-string values gracefully and provide appropriate fallback mechanisms in your code. With the knowledge and practical examples provided in this guide, you can confidently leverage the is_string() function in your PHP projects to enhance data validation and improve overall code reliability.
Table of Contents
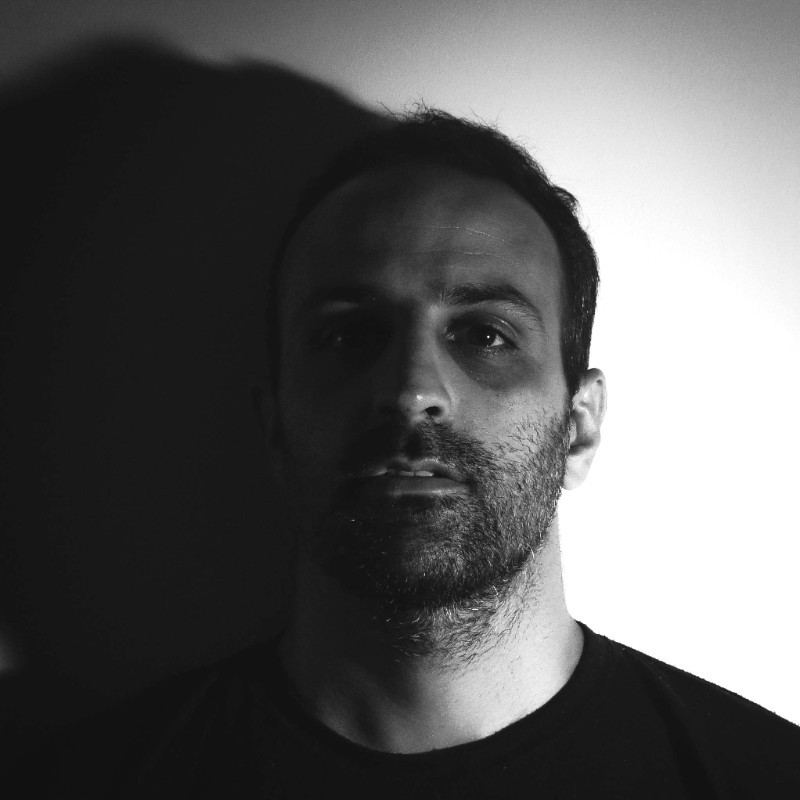
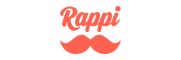