Demystifying PHP’s isset() and empty()
When working with PHP, two commonly used functions for checking variable states are isset() and empty(). These functions play a vital role in determining the existence and emptiness of variables, which are essential aspects of programming. However, their subtle differences and specific use cases can often lead to confusion. In this blog post, we’ll dive deep into the world of PHP’s isset() and empty() functions, unraveling their nuances and providing clear examples of when to use each.
1. Understanding isset(): Checking Variable Existence
The isset() function in PHP serves as a tool to verify whether a variable exists and whether it has been assigned a non-null value. This function returns true if the variable is defined and not null, and false otherwise. Here’s the basic syntax of the isset() function:
php if (isset($variable)) { // Do something if the variable is set and not null } else { // Do something else if the variable is not set or is null }
Example:
php $name = "John"; if (isset($name)) { echo "Hello, $name!"; } else { echo "Name variable is not set."; }
In this example, since the $name variable is set and contains a non-null value (“John”), the output will be “Hello, John!”.
2. Exploring empty(): Evaluating Variable Emptiness
On the other hand, the empty() function is used to determine whether a variable is considered “empty.” A variable is considered empty if it is not set, it’s set to null, an empty string “”, the integer 0, the float 0.0, an empty array, or a variable with a value of false. The empty() function returns true if the variable is considered empty, and false otherwise. Here’s how you can use the empty() function:
php if (empty($variable)) { // Do something if the variable is empty } else { // Do something else if the variable is not empty }
Example:
php $email = ""; if (empty($email)) { echo "Email address is empty."; } else { echo "Email address is not empty."; }
In this example, since the $email variable is an empty string (“”), the output will be “Email address is empty.”
3. Differentiating Between isset() and empty()
At a glance, both isset() and empty() might seem similar, as they both deal with checking the state of variables. However, understanding their differences is crucial for writing accurate and efficient code.
Variable Existence vs. Variable Emptiness: The primary distinction between the two functions is what they’re checking for. isset() solely focuses on whether a variable exists and is not null, while empty() is concerned with whether a variable is considered “empty” based on specific conditions.
Return Values: isset() returns true if the variable is set and not null, and false otherwise. Conversely, empty() returns true if the variable is considered empty, and false if it’s not empty.
Conditions for Empty: It’s important to note that while an empty string (“”) is considered “empty” according to the empty() function, it would still be considered “set” according to the isset() function.
4. Use Cases and Best Practices
4.1. When to Use isset():
- Checking Variable Existence: Use isset() when you want to determine whether a variable has been defined and is not null before using it. This is particularly useful to avoid using uninitialized or non-existent variables.
4.2. When to Use empty():
- Checking Input Fields: Use empty() when you’re dealing with form input fields and want to check if a field is left blank. However, be cautious when using it, as it considers the integer 0 and other non-null values as empty.
- Empty Array Check: You can use empty() to check if an array is empty, which is helpful when you want to handle arrays differently based on whether they have elements.
5. Common Pitfalls and Misunderstandings
- Undefined Variables: Using isset() on an undefined variable won’t produce an error, as the function’s purpose is to check if a variable is set. However, using empty() on an undefined variable will result in an error, as PHP cannot evaluate the emptiness of something that doesn’t exist.
- Non-null Values: Remember that empty() considers various non-null values, such as 0 and false, as empty. Be cautious when using empty() to avoid unexpected results.
Conclusion
In conclusion, the isset() and empty() functions in PHP serve distinct purposes when it comes to checking variable states. isset() is primarily used to verify the existence and non-null status of a variable, while empty() determines whether a variable is considered “empty” based on specific conditions. Understanding these differences is essential for writing accurate and efficient PHP code.
When using isset(), you ensure that the variable you’re working with is defined and not null, thus preventing potential errors. On the other hand, empty() is a useful tool for checking emptiness in various contexts, from input fields to arrays.
By demystifying the roles and behaviors of isset() and empty(), you can confidently navigate through PHP code, avoiding common pitfalls and making informed decisions about when to use each function. This knowledge empowers you to write more robust and reliable PHP applications.
Remember, mastering these fundamental functions is a crucial step towards becoming a proficient PHP developer, capable of creating clean, efficient, and error-free code.
Table of Contents
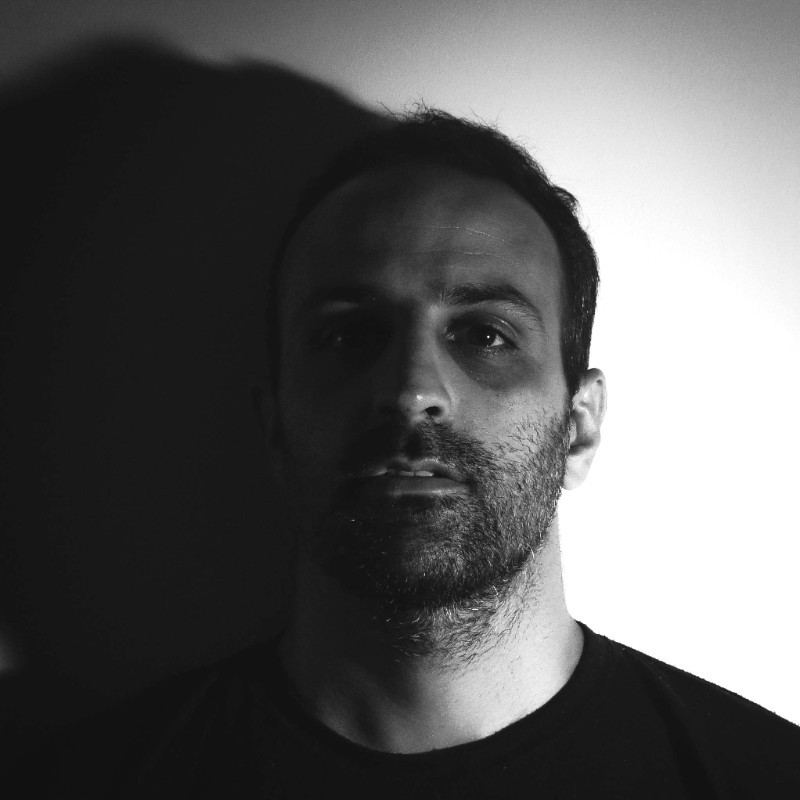
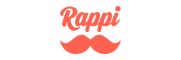