Exploring the Power of PHP’s lcfirst() Function
In the vast world of programming, readability and maintainability are paramount. Code that is easy to understand and modify not only saves time but also reduces the likelihood of errors. PHP, a widely used server-side scripting language, offers a plethora of functions to aid in achieving these goals. One such function that often flies under the radar is lcfirst(). In this comprehensive guide, we’ll delve into the power of PHP’s lcfirst() function and how it can enhance your code quality.
Table of Contents
1. Understanding the Basics
Before we dive into the intricacies of lcfirst(), let’s start with the fundamentals. lcfirst() is a PHP function that stands for “lowercase first.” As the name suggests, its primary purpose is to convert the first character of a given string to lowercase. While this may seem like a simple task, the implications of using lcfirst() go beyond its apparent simplicity.
1.1. Syntax
The syntax for the lcfirst() function is straightforward:
php lcfirst(string $string): string
Here, $string represents the input string, and the function returns a new string with the first character converted to lowercase. The original string remains unchanged.
2. Code Readability and Maintainability
One of the key reasons to embrace lcfirst() is its significant impact on code readability and maintainability. By converting the first character of a string to lowercase, you can adhere to coding standards and naming conventions more effectively. Let’s explore how this function can be a game-changer in real-world scenarios.
2.1. Improved Variable Naming
Consider a scenario where you need to declare a variable to hold the name of a person. Without lcfirst(), you might write the following:
php $Name = "John";
While this code is technically correct, it doesn’t adhere to common naming conventions where variables typically start with a lowercase letter. By using lcfirst(), you can make your code more readable:
php $name = "John";
This small change can have a big impact on code consistency and ease of collaboration, especially in larger projects with multiple developers.
2.2. Enhanced Function and Method Names
Similar to variable naming, function and method names should also follow naming conventions. Using lcfirst() allows you to create more meaningful and consistent function names:
php function calculateInterest($principal, $rate, $time) { // Function logic here }
With lcfirst():
php function calculateInterest($principal, $rate, $time) { // Function logic here }
This makes it easier for you and your team to quickly grasp the purpose of each function, leading to more efficient code maintenance and debugging.
2.3. Generating Dynamic HTML IDs and Classes
When working with web development, you often need to generate dynamic HTML elements with unique IDs or classes. lcfirst() can be a handy tool in this context. For example, if you have a list of products and want to generate unique IDs for each item:
php $products = ["Laptop", "Smartphone", "Tablet"]; foreach ($products as $product) { $productId = lcfirst($product) . "Id"; echo "<div id='$productId'>$product</div>"; }
This code snippet demonstrates how lcfirst() can be used to generate IDs based on product names, ensuring uniqueness and adherence to naming conventions.
3. String Manipulation
Beyond improving code readability and maintainability, lcfirst() can be a valuable tool for string manipulation. Let’s explore some practical examples where this function can simplify tasks.
3.1. Capitalize the First Letter of a Sentence
Suppose you have a string containing a sentence, and you want to capitalize only the first letter. lcfirst() can be used in conjunction with other string functions to achieve this:
php $sentence = "hello, world!"; $firstLetterCapitalized = ucfirst(lcfirst($sentence)); echo $firstLetterCapitalized; // Output: "Hello, world!"
In this example, lcfirst() converts the first letter to lowercase, and then ucfirst() capitalizes it, resulting in a properly formatted sentence.
3.2. Standardize Input Data
When dealing with user input or data from external sources, it’s essential to standardize the format to ensure consistency in your application. lcfirst() can be helpful in normalizing data, especially when it comes to user-generated content:
php $userInput = "jOhN doE"; $standardizedInput = ucfirst(strtolower($userInput)); echo $standardizedInput; // Output: "John doe"
Here, strtolower() is used in conjunction with lcfirst() to ensure that the input starts with a lowercase letter, regardless of the user’s input.
4. Error Prevention
Consistency in coding practices can help prevent errors and improve code quality. lcfirst() contributes to this by reducing the likelihood of naming and formatting inconsistencies. Let’s explore how it can play a role in error prevention.
4.1. Avoiding Variable Name Collisions
In larger codebases or collaborative projects, naming collisions can be a nightmare to debug and resolve. Using lcfirst() to ensure all variables start with a lowercase letter can help mitigate this issue:
php $userId = 123; $userName = "John Doe";
With lcfirst():
php $userId = 123; $userName = "John Doe";
By following a consistent naming convention, you minimize the chances of inadvertently overwriting variables with similar names.
4.2. Preventing Function Name Confusion
Imagine a scenario where two developers are working on different parts of a project, and both decide to create a function to calculate interest. Without lcfirst() and naming conventions, you might end up with conflicting function names:
php function calculateInterest($principal, $rate, $time) { // Function logic here } function CalculateInterest($amount, $interestRate, $years) { // Function logic here }
By utilizing lcfirst() and adhering to naming conventions, you can prevent such conflicts:
php function calculateInterest($principal, $rate, $time) { // Function logic here } function calculateInterest($amount, $interestRate, $years) { // Function logic here }
This reduces the chances of unintentional function name clashes and makes your code more robust.
5. Best Practices for Using lcfirst()
To harness the full power of lcfirst(), it’s essential to follow some best practices:
5.1. Consistent Naming Conventions
Adopt and adhere to consistent naming conventions for variables, functions, and methods. Combining lcfirst() with these conventions will yield the best results.
5.2. Avoid Excessive Use
While lcfirst() can be beneficial, avoid excessive use. Reserve it for cases where it genuinely improves code readability and maintainability.
5.3. Consider Locale
Be aware of locale-specific considerations when working with character case conversions. Some languages may have specific rules for capitalization.
5.4. Use in Conjunction with Other String Functions
lcfirst() often works best when combined with other string functions like ucfirst(), strtolower(), or strtoupper() to achieve the desired string manipulation.
Conclusion
In the world of programming, even the smallest details can make a significant difference in code quality. PHP’s lcfirst() function, with its ability to convert the first character of a string to lowercase, is a powerful tool for enhancing code readability, maintainability, and error prevention. By following best practices and incorporating lcfirst() into your coding workflow, you can write cleaner, more consistent, and less error-prone PHP code.
So, the next time you’re crafting PHP code, remember the hidden gem that is lcfirst(), and let it work its magic in making your codebase more elegant and maintainable. Your future self and your fellow developers will thank you for it. Happy coding!
Now that you’ve explored the power of lcfirst(), why not dive deeper into PHP’s string manipulation functions and unlock even more coding possibilities?
Table of Contents
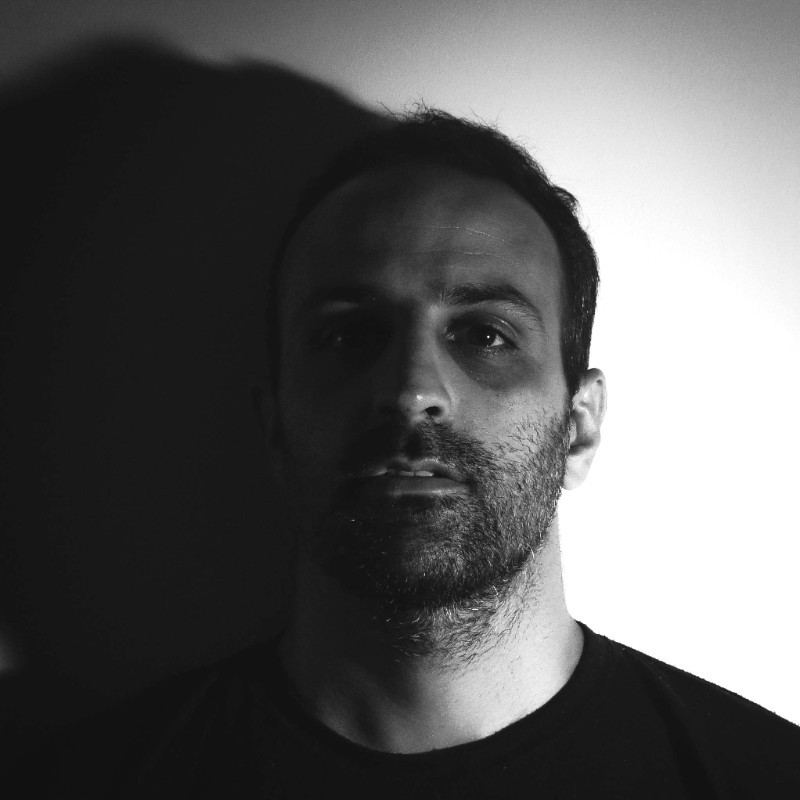
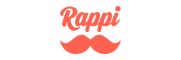