Working with PHP’s list() Function: A Comprehensive Guide
PHP is a versatile and widely-used programming language, known for its web development capabilities. It offers a plethora of functions and features that simplify tasks for developers. Among these is the list() function, which provides a convenient way to assign variables from arrays and has numerous applications in various programming scenarios. In this comprehensive guide, we will dive deep into the list() function, exploring its syntax, applications, and providing practical examples to help you master its usage.
Table of Contents
1. Understanding the Basics
1.1. Syntax of list()
The list() function is a unique construct in PHP that allows you to assign variables to the elements of an array in a single line of code. It follows this basic syntax:
php list($var1, $var2, ...) = array;
Here’s how it works:
- The list of variables on the left side of the assignment operator (=) will be assigned values from the array on the right side.
- The order of variables on the left must match the order of elements in the array.
2. Assigning Variables from an Indexed Array
Let’s start with a simple example. Consider an indexed array:
php $fruits = ['apple', 'banana', 'cherry'];
You can use the list() function to assign values from this array to individual variables:
php list($firstFruit, $secondFruit, $thirdFruit) = $fruits;
Now, $firstFruit will hold ‘apple’, $secondFruit will hold ‘banana’, and $thirdFruit will hold ‘cherry’.
3. Assigning Variables from an Associative Array
The list() function also works with associative arrays. Consider the following associative array:
php $person = ['name' => 'John', 'age' => 30, 'city' => 'New York'];
You can assign values from this array to variables by specifying the array keys:
php list($name, $age, $city) = $person;
Now, $name will hold ‘John’, $age will hold 30, and $city will hold ‘New York’.
4. Practical Applications
4.1. Swapping Variables
One of the most common use cases for the list() function is swapping the values of two variables without needing a temporary variable. Here’s an example:
php $a = 5; $b = 10; list($a, $b) = [$b, $a]; // Now, $a is 10, and $b is 5
This code efficiently swaps the values of $a and $b by using list() with an array on the right side of the assignment.
4.2. Exploding Strings
The list() function is extremely useful when working with data from delimited strings. Suppose you have a comma-separated string of values like this:
php $csv = 'John,Doe,30';
You can split this string into variables using the explode() function and list():
php $csv = 'John,Doe,30'; list($firstName, $lastName, $age) = explode(',', $csv);
Now, $firstName holds ‘John’, $lastName holds ‘Doe’, and $age holds 30.
4.3. Fetching Data from a Database
When working with databases, you often fetch rows as associative arrays. The list() function can help you easily extract values from these arrays for further processing. Consider a database query that retrieves a user’s information:
php $userData = [ 'id' => 123, 'username' => 'johndoe', 'email' => 'john@example.com', ]; list($id, $username, $email) = $userData;
Now, you have individual variables holding each piece of user data, making it more convenient to work with.
4.4. Dealing with Multidimensional Arrays
You can also use the list() function to extract values from multidimensional arrays. Suppose you have an array representing a student’s information:
php $studentInfo = [ 'name' => 'Alice', 'grades' => [ 'math' => 95, 'science' => 88, 'history' => 92, ], ];
You can extract specific grades using list():
php list('math' => $mathGrade, 'science' => $scienceGrade, 'history' => $historyGrade) = $studentInfo['grades'];
Now, you have individual variables ($mathGrade, $scienceGrade, and $historyGrade) containing the grades for the student.
5. Advanced Usage
5.1. Skipping Values
In some cases, you might not need to assign values to all variables. You can skip variables by leaving placeholders in the list. For example:
php list($first, , $third) = [1, 2, 3];
Here, the second value is skipped, and $first will be 1, while $third will be 3.
5.2. Using the list() Function with Function Returns
You can also use the list() function to directly assign values from a function return. For instance, if you have a function that returns an array of data:
php function getPersonData() { return ['John', 'Doe', 30]; } list($firstName, $lastName, $age) = getPersonData();
This way, you can easily extract and work with the returned data.
5.3. Combining list() with foreach
list() can be combined with foreach loops to iterate through arrays and extract values conveniently. Consider an array of users’ data:
php $users = [ ['John', 'Doe', 30], ['Alice', 'Smith', 25], ['Bob', 'Johnson', 35], ]; foreach ($users as list($firstName, $lastName, $age)) { // Process each user's data echo "$firstName $lastName is $age years old.<br>"; }
This code snippet iterates through the array of users, assigning each user’s data to variables for processing within the loop.
6. Handling Errors and Caveats
While the list() function is a powerful tool, there are some caveats and error-handling considerations to keep in mind:
6.1. Mismatched Variables and Array Elements
If the number of variables on the left side of the list() function doesn’t match the number of elements in the array, PHP will generate an error. For example:
php list($a, $b, $c) = [1, 2];
In this case, you’ll get a “Notice: Undefined offset” error because there are more variables than elements in the array.
6.2. Indexed Arrays vs. Associative Arrays
When working with indexed arrays, the order of variables in the list() function should match the order of elements in the array. For associative arrays, the keys must match. Failing to do so will result in incorrect variable assignment.
6.3. Undefined Variables
If you try to access a variable that hasn’t been assigned a value through list(), PHP will raise an “Undefined variable” notice. Always ensure that you have assigned values to variables before using them.
Conclusion
The list() function in PHP is a valuable tool for simplifying variable assignment and manipulation, especially when dealing with arrays. By understanding its syntax and applications, you can enhance your coding efficiency and readability. Whether you’re swapping values, processing data from databases, or working with complex arrays, the list() function offers a concise and elegant solution.
Incorporate the knowledge gained from this comprehensive guide into your PHP projects, and you’ll find yourself writing more efficient and concise code. Experiment with various scenarios to master the versatility of the list() function and unlock its full potential in your development endeavors.
Table of Contents
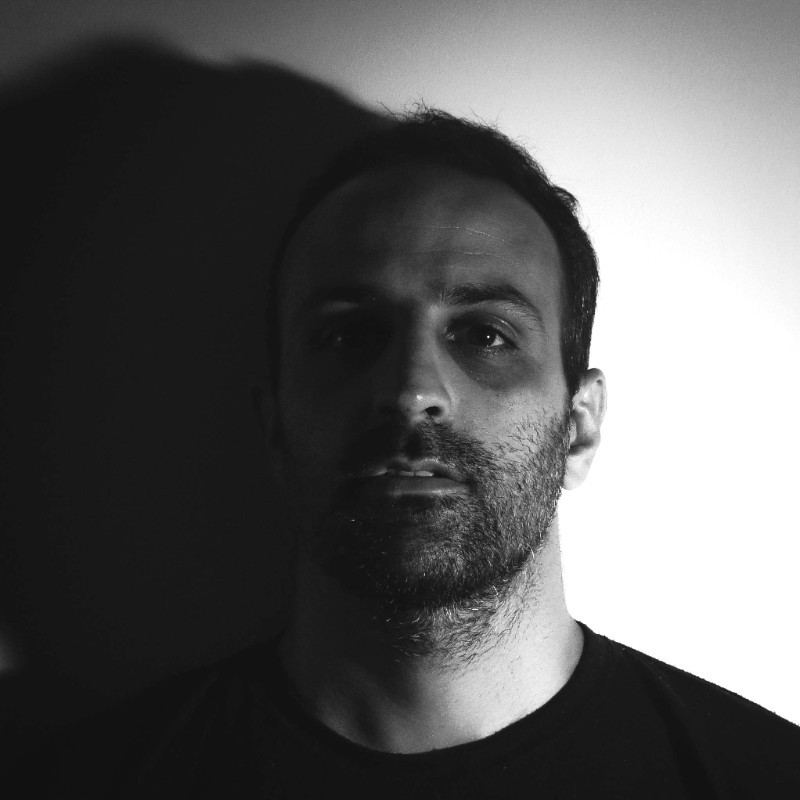
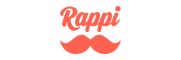