Getting to Grips with PHP Loops: The Complete Guide
If you’re a budding web developer or someone who wants to enhance their PHP skills, you’ve come to the right place. PHP loops are fundamental constructs that allow you to execute a block of code repeatedly based on a certain condition. Understanding how to use loops efficiently can significantly improve your programming capabilities. In this comprehensive guide, we’ll explore PHP loops from the ground up, providing you with a clear understanding of each type and their practical applications. So, let’s get started!
1. Introduction to Loops
In programming, loops play a crucial role in executing repetitive tasks with ease and efficiency. PHP provides several types of loops, each designed for specific use cases. The primary types of loops in PHP are the while loop, the do-while loop, the for loop, and the foreach loop. Understanding their differences and knowing when to use each loop construct is essential for writing clean and effective code.
2. The while Loop
The while loop is the most basic type of loop in PHP. It repeatedly executes a block of code as long as a given condition remains true. The syntax of the while loop is as follows:
php while (condition) { // Code to be executed }
Let’s take a practical example to demonstrate the while loop:
php // Printing numbers from 1 to 5 using a while loop $counter = 1; while ($counter <= 5) { echo $counter . " "; $counter++; } // Output: 1 2 3 4 5
3. The do-while Loop
The do-while loop is similar to the while loop, but the key difference is that it executes the block of code first and then checks the condition. This means the code inside the loop will always run at least once. The syntax of the do-while loop is as follows:
php do { // Code to be executed } while (condition);
Let’s see an example of the do-while loop:
php // Printing numbers from 1 to 5 using a do-while loop $counter = 1; do { echo $counter . " "; $counter++; } while ($counter <= 5); // Output: 1 2 3 4 5
4. The for Loop
The for loop is commonly used when you know the number of times you want to repeat a specific block of code. It consists of three parts: initialization, condition, and increment/decrement. The syntax of the for loop is as follows:
php for (initialization; condition; increment/decrement) { // Code to be executed }
Here’s an example of the for loop:
php // Printing numbers from 1 to 5 using a for loop for ($i = 1; $i <= 5; $i++) { echo $i . " "; } // Output: 1 2 3 4 5
5. The foreach Loop
The foreach loop is used exclusively with arrays to iterate through each element in the array. It simplifies the process of traversing arrays and is an excellent choice for working with collections of data. The syntax of the foreach loop is as follows:
php foreach ($array as $value) { // Code to be executed for each $value }
Let’s consider an example of the foreach loop:
php // Iterating through an array using a foreach loop $fruits = array("Apple", "Banana", "Orange"); foreach ($fruits as $fruit) { echo $fruit . " "; } // Output: Apple Banana Orange
6. Loop Control Statements
Loop control statements allow you to modify the flow of a loop. They offer more control over loop execution based on specific conditions.
6.1. break
The break statement is used to terminate a loop prematurely when a certain condition is met. It allows you to exit the loop and continue with the next section of code outside the loop. The break statement is commonly used with the while, do-while, for, and foreach loops. Let’s see an example:
php // Printing numbers from 1 to 5, but breaking the loop when 3 is encountered for ($i = 1; $i <= 5; $i++) { if ($i === 3) { break; } echo $i . " "; } // Output: 1 2
6.2. continue
The continue statement is used to skip the current iteration of the loop and move to the next iteration immediately. It allows you to bypass specific iterations without exiting the loop entirely. The continue statement is also commonly used with the while, do-while, for, and foreach loops. Let’s take an example:
php // Skipping number 3 and continuing with the loop for ($i = 1; $i <= 5; $i++) { if ($i === 3) { continue; } echo $i . " "; } // Output: 1 2 4 5
6.3. exit
The exit statement is used to terminate the entire script when a specific condition is met. It can be used within a loop to exit the script altogether. Keep in mind that using exit will stop the entire PHP script from executing. Here’s an example:
php // Printing numbers from 1 to 5, but exiting the script when 4 is encountered for ($i = 1; $i <= 5; $i++) { echo $i . " "; if ($i === 4) { exit; } } // Output: 1 2 3 4
7. Nested Loops
A nested loop is a loop inside another loop. It allows you to execute a loop within a loop, providing a way to handle complex tasks efficiently. However, be cautious with nested loops, as they can lead to performance issues if not used wisely. Here’s an example of a nested for loop:
php // Multiplication table using nested loops for ($i = 1; $i <= 5; $i++) { for ($j = 1; $j <= 5; $j++) { echo $i * $j . " "; } echo "\n"; } /* Output: 1 2 3 4 5 2 4 6 8 10 3 6 9 12 15 4 8 12 16 20 5 10 15 20 25 */
8. Best Practices for Using Loops
- Choose the appropriate loop type for your specific task.
- Avoid infinite loops by ensuring that the loop condition will eventually become false.
- Minimize the use of nested loops to maintain code readability and performance.
- Use loop control statements (break, continue) when necessary to fine-tune loop behavior.
- Keep your code clean and well-documented to facilitate future modifications.
Conclusion
In this comprehensive guide, we’ve explored PHP loops and learned how to use them effectively. Loops are essential tools for any PHP developer, enabling you to automate repetitive tasks and handle complex operations. By mastering the various types of loops and loop control statements, you can significantly enhance your PHP coding skills. Remember to practice regularly and apply what you’ve learned in real-world scenarios to become a proficient PHP developer. Happy coding!
Table of Contents
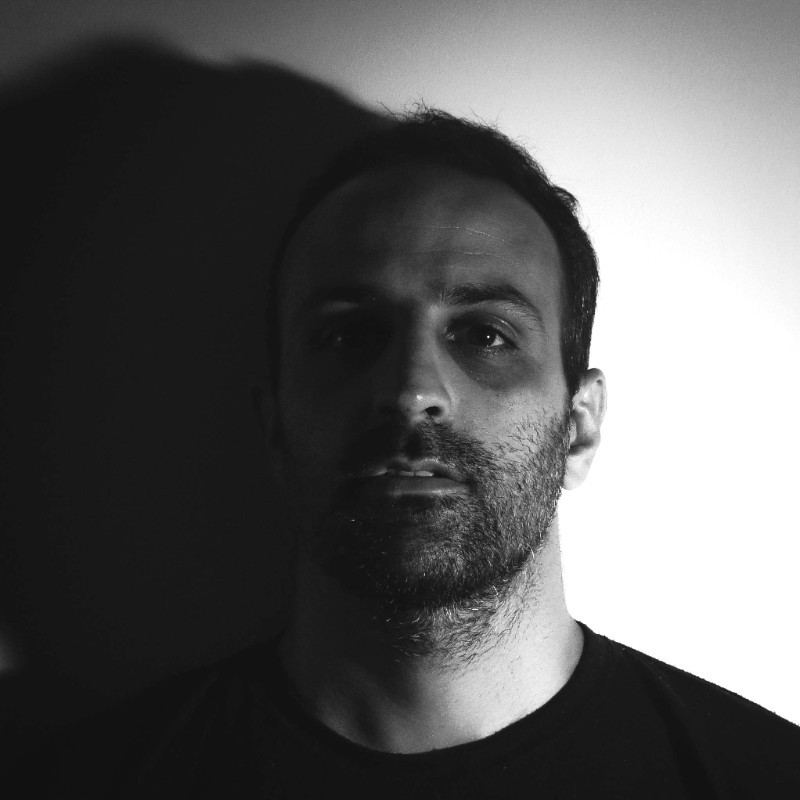
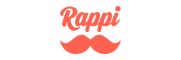