Demystifying PHP’s Magic Methods: A Deep Dive
PHP, a popular server-side scripting language, is known for its flexibility and versatility. One of the intriguing features that set PHP apart is its “magic methods.” These seemingly enchanting methods enable developers to perform extraordinary actions behind the scenes without explicitly defining them in the class. In this blog, we will embark on a journey to demystify PHP’s magic methods, unveiling their purpose, applications, and best practices. Whether you are a novice or an experienced PHP developer, this deep dive will enrich your understanding of the language’s mystical capabilities.
1. What are Magic Methods?
In PHP, magic methods are special methods that start with the prefix “__” (double underscore). These methods are automatically called by the PHP interpreter under specific circumstances. Magic methods allow developers to implement certain behaviors in classes without having to write explicit code for those actions. By leveraging these methods, you can create classes that respond dynamically to various events, such as property access, method calls, instantiation, and more.
2. The Magic Behind Magic Methods
2.1. __construct() – The Constructor Magic
The __construct() magic method is perhaps the most commonly used magic method in PHP. It is automatically invoked when an object of the class is created, allowing you to initialize properties or perform setup tasks.
php class MyClass { public function __construct() { // Initialization code here } }
2.2. __destruct() – The Destructor Magic
The __destruct() magic method is the counterpart of the constructor. It gets called automatically when an object is no longer referenced or explicitly destroyed using the unset() function. It is often used to clean up resources or perform actions before the object is removed from memory.
php class MyClass { public function __destruct() { // Clean-up code here } }
2.3. __get() and __set() – Property Access Magic
These magic methods come into play when accessing inaccessible or non-existing properties of an object. __get() is called when trying to read a property that is not accessible, and __set() is called when trying to assign a value to a non-existent or inaccessible property.
php class MyClass { private $data = array(); public function __get($name) { return $this->data[$name]; } public function __set($name, $value) { $this->data[$name] = $value; } }
2.4. __isset() and __unset() – Property Existence Magic
The __isset() magic method is triggered when using the isset() or empty() functions on inaccessible or non-existent properties. The __unset() method is called when the unset() function is used on an inaccessible property.
php class MyClass { private $data = array(); public function __isset($name) { return isset($this->data[$name]); } public function __unset($name) { unset($this->data[$name]); } }
2.5. __call() and __callStatic() – Method Invocation Magic
The __call() magic method is invoked when trying to call a non-existent or inaccessible method on an object. Similarly, __callStatic() is called when attempting to call a non-existent or inaccessible static method on a class.
php class MyClass { public function __call($name, $arguments) { // Handle the method call dynamically } public static function __callStatic($name, $arguments) { // Handle the static method call dynamically } }
2.6. __toString() – Object Conversion Magic
The __toString() magic method allows you to define how an object should be represented as a string when used in a context where a string is expected. This is especially useful for debugging or displaying custom representations of objects.
php class MyClass { public function __toString() { return 'Custom representation of the object'; } }
3. When to Use Magic Methods
While magic methods can be incredibly handy, they should be used judiciously. Here are some guidelines on when to use them:
- Constructor and Destructor: Utilize __construct() and __destruct() for initializing and cleaning up resources, respectively.
- Property Access: Use __get() and __set() to intercept read and write access to properties. This can be helpful in implementing dynamic property behavior.
- Property Existence: Implement __isset() and __unset() if you want to control the behavior of isset() and unset() on object properties.
- Method Invocation: Leverage __call() and __callStatic() when you want to handle method calls dynamically, especially when dealing with a wide variety of method names.
- Object Conversion: Implement __toString() if you want to define a custom representation of an object when it is used in a string context.
4. Avoiding Magic Abuse
While magic methods provide a great deal of flexibility, it’s essential not to overuse them. Overreliance on magic methods can lead to code that is difficult to understand and maintain. Here are some tips to avoid magic abuse:
- Document Your Magic: When you use magic methods, make sure to document them thoroughly. Explain what each method does and when it is triggered.
- Clear Naming Conventions: Choose meaningful names for your magic methods to make their purpose obvious and consistent.
- Use Regular Methods When Appropriate: Sometimes, it might be more straightforward and clearer to use regular methods instead of magic methods, especially for commonly used functionality.
- Be Consistent: If you decide to use magic methods, stick to a consistent pattern throughout your codebase. This will make it easier for other developers to understand and maintain your code.
Conclusion
In this deep dive into PHP’s magic methods, we have unveiled the wizardry behind these enchanting functions. Magic methods empower PHP developers to create dynamic and flexible classes, responding to various events without explicitly defining each action. By understanding their purpose and best practices, you can wield the power of magic methods effectively and responsibly. Remember, with great power comes great responsibility – use magic methods wisely, and they will serve as a valuable addition to your PHP programming arsenal. Happy coding!
Table of Contents
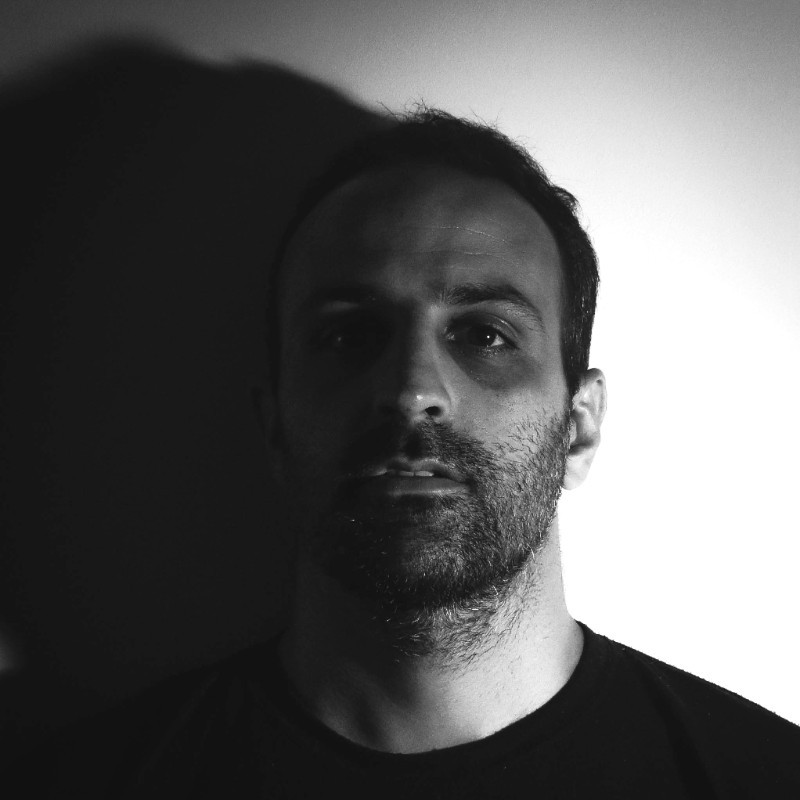
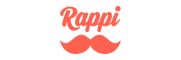