Mastering PHP Sessions: A Comprehensive Guide
If you’re a web developer, chances are you’ve worked with PHP and its built-in session management system. PHP sessions allow you to maintain state and data across multiple page requests for a single user. Understanding and mastering PHP sessions is crucial for building secure and efficient web applications. In this comprehensive guide, we’ll delve into the world of PHP sessions, covering the basics, handling, security best practices, and advanced techniques with practical code examples.
1. Introduction to PHP Sessions
1.1 What are Sessions?
In web development, sessions are a way to preserve user-specific information across multiple requests. When a user visits a website, the server assigns a unique identifier known as a “session ID” to that user. This session ID is then used to associate the user’s data with the server-side session storage. Sessions are essential for maintaining stateful behavior in stateless HTTP protocol.
1.2 How Sessions Work
When a user accesses a PHP page for the first time, a session is started automatically. PHP generates a unique session ID for that user, which is usually stored in a cookie or passed through the URL. This session ID is sent with every subsequent request from the client to the server, allowing PHP to identify the user and retrieve their stored session data.
1.3 Session ID and Cookies
By default, PHP uses cookies to store the session ID. Cookies are small pieces of data sent by the server and stored on the client’s browser. They are a convenient way to handle sessions, but they have some limitations, such as being susceptible to cross-site scripting (XSS) attacks.
2. Starting a PHP Session
2.1 Configuring Session Settings
Before starting a PHP session, it’s essential to configure session settings according to your application’s requirements. PHP provides a set of configuration options to control session behavior, such as session timeout, cookie parameters, and session storage.
php // Example: Configuring session timeout to 30 minutes session_start(['cookie_lifetime' => 1800]);
2.2 Starting a Session
Starting a PHP session is as simple as calling the session_start() function at the beginning of your script. This function initializes the session and allows you to access or set session variables.
php // Starting a PHP session session_start(); // Setting a session variable $_SESSION['username'] = 'JohnDoe'; // Retrieving the session variable echo $_SESSION['username']; // Output: JohnDoe
2.3 Session Storage Options
By default, PHP stores sessions on the server’s filesystem. However, you can customize the session storage to use databases, memcached, or other external storage systems. To do this, you need to implement a custom session handler by extending the SessionHandler class.
php // Example: Using a database for session storage class DatabaseSessionHandler extends \SessionHandler { // Implement the necessary methods for database storage // ... } // Set the custom session handler $handler = new DatabaseSessionHandler(); session_set_save_handler($handler, true); session_start();
3. Working with Session Data
3.1 Setting Session Variables
Session variables are used to store user-specific data during a session. You can assign values to session variables as follows:
php // Starting the session session_start(); // Setting session variables $_SESSION['user_id'] = 123; $_SESSION['email'] = 'john@example.com';
3.2 Retrieving Session Data
To retrieve session data, access the session variables using the $_SESSION superglobal array:
php // Starting the session session_start(); // Retrieving session data $userID = $_SESSION['user_id']; $email = $_SESSION['email'];
3.3 Unsetting Session Variables
If you want to remove specific session variables, use the unset() function:
php // Unsetting a session variable unset($_SESSION['email']);
3.4 Destroying a Session
To completely destroy a session and all its data, use the session_destroy() function:
php // Destroying the session session_start(); session_destroy();
4. Handling Session Timeouts
4.1 Setting Session Timeout
The session timeout determines how long a session remains active after the user becomes inactive. You can set the timeout using the cookie_lifetime option during session configuration.
php // Example: Setting session timeout to 1 hour session_start(['cookie_lifetime' => 3600]);
4.2 Handling Expired Sessions
Expired sessions can cause issues and may lead to unexpected behavior. To handle expired sessions gracefully, you can use the session.gc_maxlifetime configuration option to specify the maximum lifetime of a session.
php // Example: Setting session lifetime to 2 hours ini_set('session.gc_maxlifetime', 7200);
4.3 Session Garbage Collection
PHP performs session garbage collection to clean up expired session data. The frequency of garbage collection is controlled by the session.gc_probability and session.gc_divisor configuration options.
php // Example: Changing the session garbage collection probability to 1% ini_set('session.gc_probability', 1); ini_set('session.gc_divisor', 100);
5. Securing PHP Sessions
5.1 Session Fixation Attacks and Prevention
Session fixation is a type of attack where an attacker sets a user’s session ID to a known value, allowing them to hijack the user’s session. To prevent this, PHP provides a session_regenerate_id() function to regenerate the session ID after successful authentication.
php // Regenerating session ID after successful login session_start(); session_regenerate_id(true);
5.2 Session Hijacking and Mitigation
Session hijacking occurs when an attacker steals a user’s session ID to gain unauthorized access. To mitigate this risk, use HTTPS to encrypt session data during transmission and implement session validation based on user agents or IP addresses.
php // Enforcing HTTPS for secure session transmission ini_set('session.cookie_secure', 1);
5.3 Cross-Site Scripting (XSS) and Session Security
Cross-Site Scripting (XSS) vulnerabilities can compromise session security. Always sanitize and validate user input to prevent XSS attacks and avoid storing sensitive data in session variables.
6. Best Practices for PHP Sessions
6.1 Regenerating Session ID
Regularly regenerating the session ID reduces the risk of session fixation and helps prevent session hijacking.
php // Regenerating session ID every 30 minutes session_start(); if (time() - $_SESSION['last_activity'] > 1800) { session_regenerate_id(true); $_SESSION['last_activity'] = time(); }
6.2 Encrypting Session Data
Sensitive session data should be encrypted before storage to enhance security.
php // Encrypting session data using a secret key $encryptedData = openssl_encrypt($_SESSION['sensitive_data'], 'AES-256-CBC', 'secret_key');
6.3 Avoiding Overuse of Sessions
Avoid storing excessive data in sessions as it can impact server performance and scalability.
6.4 Handling Concurrent Requests
If your application handles multiple concurrent requests, ensure that your session management can handle race conditions and concurrent write access.
7. Advanced PHP Session Techniques
7.1 Flash Messages with Sessions
Flash messages are used to display temporary messages to users, such as success or error messages, and are often used with redirects.
php // Setting a flash message function setFlashMessage($message, $type = 'info') { session_start(); $_SESSION['flash_message'] = [ 'message' => $message, 'type' => $type ]; } // Displaying flash messages session_start(); if (isset($_SESSION['flash_message'])) { echo '<div class="' . $_SESSION['flash_message']['type'] . '">' . $_SESSION['flash_message']['message'] . '</div>'; unset($_SESSION['flash_message']); }
7.2 Cross-Origin Resource Sharing (CORS) and Sessions
If your application serves resources to other domains, you need to handle CORS headers carefully to ensure proper session handling.
php // Setting CORS headers header('Access-Control-Allow-Origin: https://example.com'); header('Access-Control-Allow-Credentials: true');
7.3 Session Clustering and Load Balancing
In distributed environments with load balancing, ensure sessions are shared and synchronized across all servers.
Conclusion
In conclusion, mastering PHP sessions is crucial for building robust and secure web applications. Understanding how sessions work, handling session data, and implementing security best practices are essential steps in creating a seamless user experience. By following the best practices and advanced techniques covered in this comprehensive guide, you can ensure your PHP sessions are efficient, secure, and scalable, leading to better user satisfaction and application performance. Happy coding!
Table of Contents
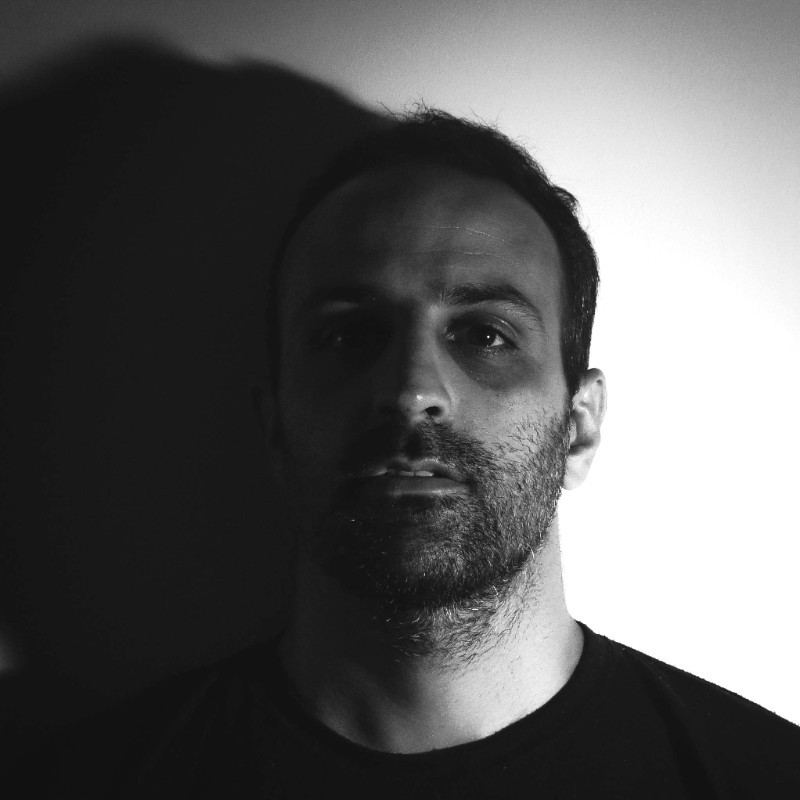
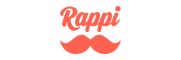