PHP’s md5() Function: Understanding Hashes
In the world of web development, security is paramount. With the constant threat of data breaches and cyberattacks, it’s essential to protect sensitive information. One of the fundamental tools in a developer’s arsenal for safeguarding data is hashing. Hashing is a technique that converts data into a fixed-size string of characters, which is typically a hexadecimal number. PHP’s md5() function is a popular choice for creating and verifying hash values. In this blog, we’ll dive deep into md5(), exploring its features, use cases, and best practices.
Table of Contents
1. What is Hashing?
Hashing is a process that takes an input (or ‘message’) and returns a fixed-length string of bytes. This string, often represented in hexadecimal format, is typically a ‘digest’ of the original data. Hashing serves various purposes, including data integrity verification and password storage.
2. How Hashing Works
At its core, hashing is a mathematical operation that takes an input (the ‘message’) and applies a hash function to produce a fixed-length output, typically a string of characters. The key characteristics of a good hash function are:
- Deterministic: The same input will always produce the same hash value.
- Fast Computation: It should be quick to compute the hash value.
- Pre-image Resistance: It should be computationally infeasible to reverse the hash and obtain the original input.
- Collision Resistance: It should be unlikely that two different inputs produce the same hash value.
Hashing is a one-way process, meaning you cannot reverse the hash value to obtain the original data. This property is useful for securely storing passwords and verifying data integrity.
3. Introduction to PHP’s md5() Function
PHP provides several built-in functions for hashing, and one of the most widely used is md5(). The md5() function generates a 32-character hexadecimal number (128 bits) as its output. It is known for its speed and simplicity, making it a popular choice for various applications.
3.1. Syntax
Here’s the basic syntax of the md5() function in PHP:
php string md5(string $str, bool $raw_output = false)
- $str: The input string to be hashed.
- $raw_output (optional): If set to true, the function returns the raw binary format of the hash.
3.2. Example
Let’s start with a simple example of using the md5() function:
php $data = "Hello, World!"; $hash = md5($data); echo "Original Data: $data<br>"; echo "MD5 Hash: $hash";
In this example, we create a variable $data containing the string “Hello, World!” and then use md5() to compute its hash. The output will look like this:
yaml Original Data: Hello, World! MD5 Hash: b10a8db164e0754105b7a99be72e3fe5
4. Use Cases for md5()
Now that we’ve covered the basics, let’s explore some common use cases for PHP’s md5() function.
4.1. Password Storage
One of the primary use cases for hashing is securely storing passwords. When a user creates an account or updates their password, you can hash the password using md5() and store the hash in your database. This way, even if the database is compromised, the actual passwords remain protected. Here’s a simplified example:
php $userPassword = "mySecurePassword123"; $hashedPassword = md5($userPassword); // Store $hashedPassword in the database
When the user attempts to log in, you can hash the entered password and compare it to the stored hash for authentication.
4.2. Data Integrity Verification
Hashing is also used to ensure data integrity during transmission or storage. By hashing the data before sending it and then verifying the hash on the receiving end, you can detect any changes or corruption in the data. Here’s a basic illustration:
php // Sender's code $data = "Important message"; $hash = md5($data); // Send $data and $hash to the receiver // Receiver's code $receivedData = // Receive data from the sender $receivedHash = // Receive hash from the sender if (md5($receivedData) === $receivedHash) { echo "Data integrity verified. Message is unchanged."; } else { echo "Data integrity compromised. Message may be tampered with."; }
4.3. Generating Unique Identifiers
Another interesting use case of md5() is generating unique identifiers (UUIDs) based on some input data. For instance, you can combine various pieces of information and hash them to create a unique identifier for a user or an object:
php $userID = "12345"; $timestamp = time(); $uniqueID = md5($userID . $timestamp); echo "Unique Identifier: $uniqueID";
This can be handy when you need to ensure that generated IDs are globally unique.
5. Best Practices for Using md5()
While md5() is a convenient and straightforward hashing function, it has some limitations and potential vulnerabilities. Here are some best practices to keep in mind when using md5():
5.1. Use Salt for Passwords
When using md5() for password storage, it’s crucial to add a ‘salt’ value to the password before hashing it. A salt is a random value that is combined with the password before hashing, making it more resistant to rainbow table attacks. Here’s an example of salting a password:
php $userPassword = "mySecurePassword123"; $salt = generateRandomSalt(); // Implement a salt generation function $hashedPassword = md5($salt . $userPassword); // Store $salt and $hashedPassword in the database
5.2. Consider Stronger Hashing Algorithms
While md5() is fast and easy to use, it is not considered secure against determined attackers with significant computational resources. For password storage, consider using stronger hashing algorithms like bcrypt or Argon2, which are specifically designed for security.
5.3. Avoid Using md5() for Cryptographic Applications
Due to its vulnerabilities, md5() should not be used for cryptographic applications, such as generating secure tokens or protecting highly sensitive data. Use dedicated cryptographic libraries and algorithms for such purposes.
5.4. Regularly Update Hashing Methods
As technology evolves, security standards change. It’s essential to stay up-to-date with the latest recommendations for hashing algorithms and update your application’s security measures accordingly.
6. Security Considerations
While md5() has served as a reliable hashing function in the past, it is essential to understand its limitations. In recent years, various vulnerabilities and collision attacks have been discovered that can compromise the security of md5()-hashed data. As a result, it is recommended to use more secure hashing algorithms like bcrypt or Argon2 for critical security applications.
Conclusion
PHP’s md5() function is a valuable tool for creating and verifying hash values in various web development scenarios. Its simplicity and speed make it a popular choice for many applications, from password storage to data integrity verification. However, it’s crucial to use md5() judiciously, understanding its limitations and vulnerabilities, and following best practices to ensure the security of your applications and data. In cases where stronger security is required, consider alternative hashing algorithms that provide better protection against modern threats.
Table of Contents
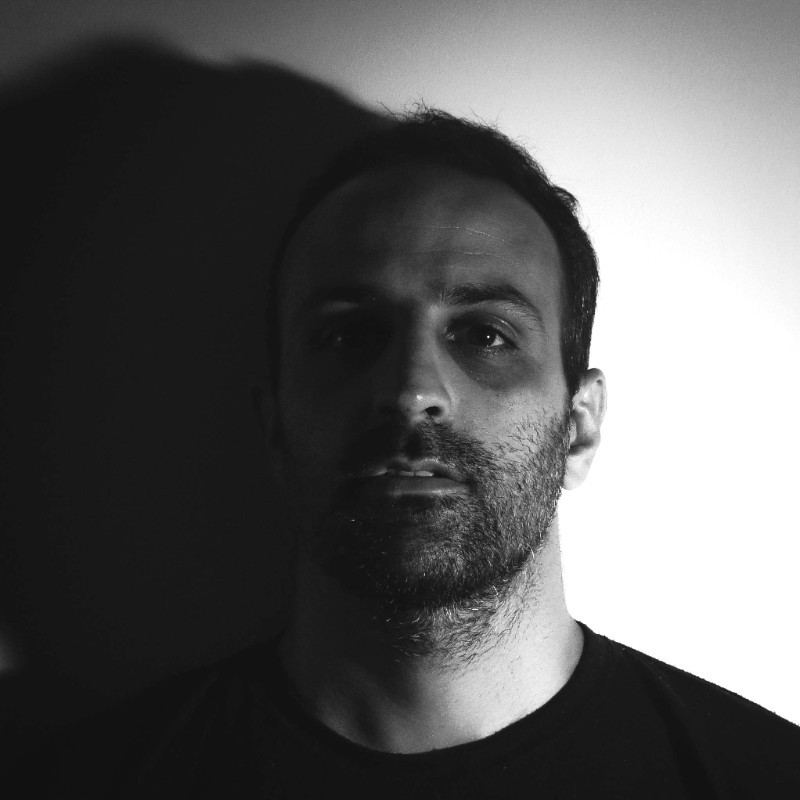
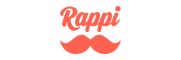