Getting Started with PHP and MySQL Integration
PHP and MySQL are a match made in heaven when it comes to building dynamic, data-driven websites. This powerful combination provides an excellent platform for developers, including those looking to hire PHP developers, to create sophisticated web applications, eCommerce sites, content management systems, and much more.
Whether you’re a beginner looking to delve into the world of PHP and MySQL or an enterprise seeking to hire PHP developers for complex projects, this guide is for you. It will walk you through the basic steps to integrate PHP and MySQL and help you understand how these technologies work together to create interactive web applications.
The Basics: What Are PHP and MySQL?
Before we get into the mechanics, let’s clarify what PHP and MySQL are. PHP is a popular, open-source server-side scripting language that’s used primarily for developing web-based applications. It’s easy to mix with HTML, highly flexible and offers excellent community support.
MySQL, on the other hand, is a relational database management system (RDBMS). It is also open-source and it is known for its speed, scalability, robustness, and ease of use. In a nutshell, MySQL helps to manage large amounts of data efficiently, making it an excellent choice for websites and applications that handle vast databases.
Setup: PHP and MySQL Environment
To start working with PHP and MySQL, whether you’re learning these technologies or planning to hire PHP developers for your projects, you’ll need to set up a development environment. There are several options available, but XAMPP is one of the easiest to use. XAMPP is an open-source platform that includes PHP, MySQL, and Apache servers, making it a convenient package for our purpose. It’s a great tool not only for individuals but also for businesses that hire PHP developers, as it allows for a unified and easily navigable work environment. You can download XAMPP from the official website and install it on your local machine.
Connecting PHP to MySQL
Now, let’s move on to connecting PHP to MySQL. To communicate with the MySQL server from a PHP script, we can use PHP’s MySQLi extension (improved MySQL extension), which supports procedural and object-oriented programming.
Here’s a simple example of how you can establish a connection using MySQLi:
```php <?php $servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDatabase"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully"; ?>
The `mysqli_connect()` function tries to open a connection to the MySQL server. The ‘die()’ function will output a message and halt the script if the connection cannot be established.
Performing Basic MySQL Operations with PHP
Once you have established a connection, you can perform operations on your MySQL database. Here are a few basic tasks you might want to do:
1. Create a table
To create a new table in your database, you can use the CREATE TABLE statement. Here’s an example where we create a table named ‘users’:
```php <?php $sql = "CREATE TABLE users ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, firstname VARCHAR(30) NOT NULL, lastname VARCHAR(30) NOT NULL, email VARCHAR(50), reg_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP )"; if ($conn->query($sql) === TRUE) { echo "Table users created successfully"; } else { echo "Error creating table: " . $conn->error; } ?>
2. Insert data
To insert data into the ‘users‘ table, you use the INSERT INTO statement:
```php <?php $sql = "INSERT INTO users (firstname, lastname, email) VALUES ('John', 'Doe', 'john@example.com')"; if ($conn->query($sql) === TRUE) { echo "New record created successfully"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } ?>
3. Retrieve data
To retrieve data from the ‘users‘ table, you use the SELECT statement:
```php <?php $sql = "SELECT id, firstname, lastname FROM users"; $result = $conn->query($sql); if ($result->num_rows > 0) { // output data of each row while($row = $result->fetch_assoc()) { echo "id: " . $row["id"]. " - Name: " . $row["firstname"]. " " . $row["lastname"]. "<br>"; } } else { echo "0 results"; } ?>
4. Update data
To update data in the ‘users’ table, you use the UPDATE statement:
```php <?php $sql = "UPDATE users SET email='newemail@example.com' WHERE id=1"; if ($conn->query($sql) === TRUE) { echo "Record updated successfully"; } else { echo "Error updating record: " . $conn->error; } ?>
5. Delete data
To delete data from the ‘users’ table, you use the DELETE statement:
```php <?php $sql = "DELETE FROM users WHERE id=1"; if ($conn->query($sql) === TRUE) { echo "Record deleted successfully"; } else { echo "Error deleting record: " . $conn->error; } ?>
Remember to close the connection after you’ve finished interacting with the database:
Conclusion
Working with PHP and MySQL provides a robust and flexible environment for developing dynamic web applications. As a developer or a company looking to hire PHP developers, understanding how to harness the power of PHP and MySQL is an essential skill set. This guide covers the basics to get you started, and it’s also a useful reference for those who hire PHP developers, ensuring they have a team with strong foundations in these critical technologies. Remember, there’s so much more to learn. As you delve deeper into this world, whether you’re coding yourself or managing a team of PHP developers, you’ll find that the possibilities are virtually endless. Happy coding!
Table of Contents
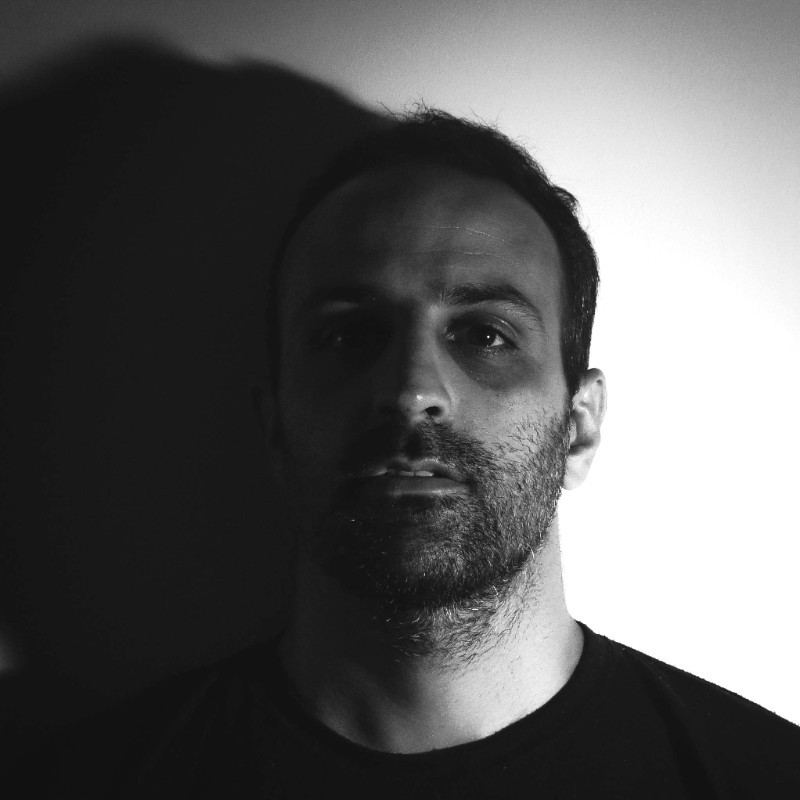
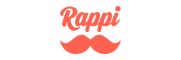