PHP 8: New Features and Changes
In the ever-evolving landscape of web development, programming languages play a pivotal role in shaping the digital world. PHP, a server-side scripting language, has been a cornerstone of web development for years. With the release of PHP 8, the language takes a significant leap forward, introducing a host of new features and improvements that promise to make development more efficient, expressive, and performant. In this blog post, we’ll delve into the exciting changes PHP 8 brings to the table, covering both syntactic enhancements and functional improvements.
1. Just-In-Time Compilation (JIT)
1.1. Boosting Performance
One of the most anticipated features in PHP 8 is the introduction of Just-In-Time (JIT) compilation. This technology allows PHP to be compiled into machine code right before execution, resulting in significantly improved performance. Traditionally, PHP has been an interpreted language, which sometimes led to execution overhead. With JIT, repetitive tasks and functions can be optimized, delivering notable speed enhancements to PHP applications.
1.2. How JIT Works
Let’s take a look at a simple code snippet to understand how JIT can impact performance:
php function calculateSum($a, $b) { return $a + $b; } $start = microtime(true); for ($i = 0; $i < 1000000; $i++) { calculateSum(5, 10); } $end = microtime(true); echo "Time taken: " . ($end - $start) . " seconds";
In PHP 7.x, the execution time for this loop might be relatively higher due to interpretation. However, in PHP 8, the JIT compiler can optimize the calculateSum function, resulting in faster execution times. This performance boost makes PHP 8 a compelling choice for applications where speed is critical.
2. Union Types
2.1. Enhancing Type Declarations
Type declarations are crucial for maintaining code integrity and preventing unexpected behavior. PHP 8 introduces union types, which allow developers to specify that a parameter or return value can accept multiple types. This enhancement adds more flexibility to type declarations, making code more robust and expressive.
2.2. Declaring Union Types
Here’s an example of using union types in function declarations:
php function displayValue(int|string $value): void { echo $value; } displayValue(42); // Output: 42 displayValue("Hello"); // Output: Hello
In this example, the displayValue function can accept either an integer or a string as its argument.
2.3. Improved Parameter Handling
Union types also enhance parameter handling, allowing functions to accept a broader range of input types. This can lead to cleaner and more versatile code without sacrificing type safety.
3. Named Arguments
3.1. Enhancing Function Invocation
PHP 8 introduces named arguments, a feature that simplifies function invocation by allowing developers to specify arguments by their parameter names, rather than their positions. This enhancement is particularly useful for functions with numerous parameters or optional values, as it eliminates confusion and reduces the likelihood of passing values in the wrong order.
3.2. Clarity in Function Calls
Consider the following function with multiple parameters:
php function createPerson($name, $age, $city) { // Create a person based on the provided details }
In PHP 7.x, calling this function might look like:
php createPerson("John", 30, "New York");
With named arguments in PHP 8, the same function call becomes much clearer:
php createPerson(name: "John", age: 30, city: "New York");
Named arguments enhance code readability and maintainability, especially when dealing with functions that have many parameters.
4. Match Expression
4.1. Evolving the Switch Statement
The switch statement has been a staple of PHP for handling multiple conditions and executing corresponding code blocks. However, its limitations have been a point of discussion among developers. PHP 8 introduces the match expression as an evolution of the switch statement, addressing some of its shortcomings and providing more flexibility in code execution.
4.2. Introducing the Match Expression
Here’s a comparison between the traditional switch statement and the new match expression:
php // Traditional switch statement $value = 2; switch ($value) { case 1: echo "One"; break; case 2: echo "Two"; break; default: echo "Other"; } // New match expression $value = 2; echo match($value) { 1 => "One", 2 => "Two", default => "Other", };
The match expression not only simplifies the syntax but also supports more advanced patterns and expressions, making code more concise and readable.
5. Attributes
5.1. Adding Metadata to Code
Attributes, also known as annotations in some other languages, allow developers to add metadata to their code. This metadata can be used by various tools, libraries, and frameworks to alter behavior or generate documentation. PHP 8 introduces attributes, enhancing code organization and enabling cleaner integration with external tools.
5.2. Applying Attributes
Here’s an example of using attributes:
php #[Route("/user/{id}", methods: ["GET"])] class UserController { // Class implementation }
In this example, the Route attribute provides information to a routing framework about how to handle requests to the specified URL. Attributes provide a more standardized and structured way of adding metadata compared to previous methods like docblocks.
Conclusion
PHP 8 brings a myriad of new features and improvements that cater to both developers’ convenience and application performance. Just-In-Time compilation revolutionizes the speed of execution, while union types and named arguments enhance code clarity and maintainability. The match expression reimagines how conditions are handled, and attributes introduce a more structured approach to metadata in code.
As you embark on your journey with PHP 8, these new features will undoubtedly streamline your development process and empower you to build more efficient and expressive web applications. Stay up-to-date with the latest changes in PHP, as the language continues to evolve and shape the digital landscape.
Incorporating these enhancements into your coding practices will not only improve your development experience but also pave the way for more efficient and powerful PHP applications. Whether you’re optimizing an existing project or starting a new one, PHP 8’s features are sure to make your development journey more exciting and productive. Happy coding!
Table of Contents
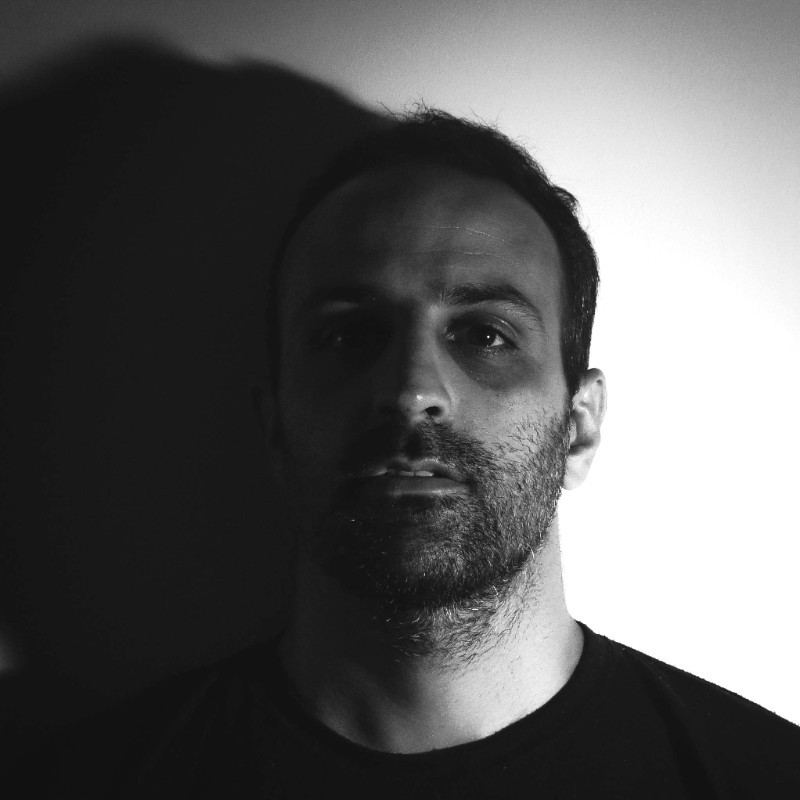
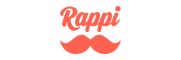