The Art of PHP’s nl2br() Function: Converting Newlines
In the realm of web development, presenting information in a clear and organized manner is paramount. One common challenge developers face is handling newlines within text, especially when it comes to displaying user-generated content. Fortunately, PHP offers a versatile solution: the nl2br() function. In this comprehensive guide, we’ll explore the power of nl2br() and how it can enhance the formatting of your web content.
Table of Contents
1. Understanding the Need for Newline Conversion
Before delving into the details of nl2br(), let’s first understand why newline conversion is crucial in web development. Newlines, represented as “\n” or “\r\n” in text, are typically used to separate paragraphs, lines of code, or any content requiring a visual break. However, web browsers do not interpret these newlines as line breaks; they treat them as regular whitespace characters. As a result, text that appears well-formatted in code can appear jumbled and unreadable on the web.
Consider a scenario where you have a user-generated comment with multiple lines. Without newline conversion, the comment would render as a single, chaotic block of text, making it challenging for users to read and comprehend.
This is where the nl2br() function comes to the rescue, allowing you to effortlessly convert newlines into HTML line breaks, which web browsers interpret correctly. Let’s dive into the details of using nl2br() effectively.
2. The Anatomy of the nl2br() Function
The nl2br() function in PHP is simple yet powerful. Its primary purpose is to locate newline characters (\n or \r\n) within a given string and replace them with the HTML line break tag, <br>. Here’s the basic syntax of the nl2br() function:
php string nl2br ( string $string [, bool $is_xhtml = true ] ) : string
- $string (required): The input string containing newline characters that you want to convert to <br> tags.
- $is_xhtml (optional): A boolean parameter indicating whether to use XHTML-compliant <br> tags (true by default). If set to false, it will use the non-XHTML <br> tag, which is simply <br>.
3. Simple Usage of nl2br()
Let’s start with a basic example to demonstrate how to use nl2br() for converting newlines in a string. Assume you have a string containing newlines:
php $originalText = "This is the first line.\nThis is the second line.";
Now, you want to convert the newlines into HTML line breaks and display the formatted text on a web page. Here’s how you can achieve that using nl2br():
php $formattedText = nl2br($originalText);
With this single line of code, you’ve transformed the original text into:
arduino This is the first line.<br>This is the second line.
When this formatted text is rendered in a web browser, it will display as:
This is the first line.
This is the second line.
As you can see, the nl2br() function has effortlessly converted the newline characters into <br> tags, resulting in proper line breaks.
4. Handling Newlines in User-Generated Content
One of the most common use cases for nl2br() is dealing with user-generated content, such as comments, forum posts, or messages. Users often input text with newlines to create well-structured content, but without proper conversion, it won’t display correctly. Let’s explore how nl2br() can be applied to user-generated content:
php $userComment = "This is a user-generated comment.\nIt spans multiple lines."; $commentWithLineBreaks = nl2br($userComment);
By applying nl2br() to the user’s comment, you ensure that the text is formatted correctly when displayed on your website:
csharp This is a user-generated comment.<br>It spans multiple lines.
Now, when the comment is rendered on the web page, it will maintain the intended formatting, making it more readable and user-friendly.
5. Controlling XHTML Compatibility
As mentioned earlier, the nl2br() function supports an optional parameter, $is_xhtml, which determines whether to use XHTML-compliant <br> tags. By default, $is_xhtml is set to true, meaning it uses <br />. However, you can set it to false if you’re working in an environment where XHTML compatibility is not a concern.
Here’s an example of how to use the $is_xhtml parameter:
php $text = "This is some text with newlines.\nAnd more newlines."; $formattedTextXHTML = nl2br($text); // Uses XHTML-compliant <br /> $formattedTextNonXHTML = nl2br($text, false); // Uses non-XHTML <br>
The resulting $formattedTextXHTML will use <br />, while $formattedTextNonXHTML will use <br>.
It’s important to note that modern web development tends to favor XHTML-compliant HTML for cleaner and more consistent code. Therefore, unless you have specific reasons to do otherwise, sticking with the default XHTML-compliant setting is a good practice.
6. Dealing with Different newline Characters
In PHP, newlines can be represented in two main ways: \n and \r\n. The former is commonly used in Unix-based systems, while the latter is prevalent in Windows environments. The nl2br() function is versatile enough to handle both types of newline characters seamlessly.
Consider a situation where your application processes user input from various sources, including both Unix and Windows users. You want to ensure that newlines are correctly converted regardless of the source. nl2br() can handle this scenario effortlessly:
php $userInputUnix = "This is a Unix-style newline.\nAnother line."; $userInputWindows = "This is a Windows-style newline.\r\nAnother line."; $formattedUnix = nl2br($userInputUnix); $formattedWindows = nl2br($userInputWindows);
In both cases, the nl2br() function will produce the correct output, ensuring that newlines are consistently converted into line breaks:
arduino This is a Unix-style newline.<br>Another line. arduino This is a Windows-style newline.<br>Another line.
This flexibility makes nl2br() a reliable choice for handling diverse sources of user-generated content.
7. Advanced Usage: Customizing the Output
While the basic usage of nl2br() is straightforward, there may be situations where you need more control over the output. For example, you might want to wrap the converted text within a <p> (paragraph) tag for better styling and readability. To achieve this, you can use nl2br() in combination with other string manipulation functions.
Here’s an example of how to customize the output:
php $userText = "This is some text with newlines.\nAnd more newlines."; // Convert newlines and wrap the result in a <p> tag $formattedText = '<p>' . nl2br($userText) . '</p>';
The $formattedText variable now contains the user’s text wrapped within a <p> tag:
html <p>This is some text with newlines.<br>And more newlines.</p>
By using this approach, you have greater control over the HTML structure and styling of the converted text.
8. Common Pitfalls and Best Practices
While nl2br() is a valuable tool for newline conversion, it’s essential to be aware of potential pitfalls and follow best practices when using it:
8.1. Input Validation:
Always validate and sanitize user-generated content before applying nl2br(). This helps prevent security vulnerabilities and ensures that only safe and well-formed content is processed.
8.2. Avoid Double Conversion:
Be cautious not to apply nl2br() twice to the same text, as it can result in excessive line breaks and formatting issues.
8.3. Consistent Formatting:
Maintain a consistent formatting style throughout your web application. Decide whether to use XHTML-compliant or non-XHTML <br> tags and stick with your choice for uniformity.
8.4. Performance Considerations:
While nl2br() is efficient for most use cases, avoid using it excessively within loops or on extremely large strings, as it may impact performance. Use it judiciously where needed.
Conclusion
In the world of web development, presenting content in a clean and organized manner is essential for an enjoyable user experience. PHP’s nl2br() function simplifies the task of converting newlines into HTML line breaks, ensuring that user-generated content displays correctly and coherently on your web pages.
By mastering the art of nl2br(), you empower yourself to handle a wide range of text formatting challenges with ease. Whether you’re building a blog platform, a social networking site, or any application that involves user-generated content, nl2br() is a valuable tool in your toolkit for achieving impeccable text formatting.
So, the next time you encounter the need to convert newlines, remember the elegance and efficiency of PHP’s nl2br() function. It’s a small yet powerful function that can make a big difference in how your web content appears to your users.
Start harnessing the power of nl2br() today, and elevate the readability and aesthetics of your web applications!
Table of Contents
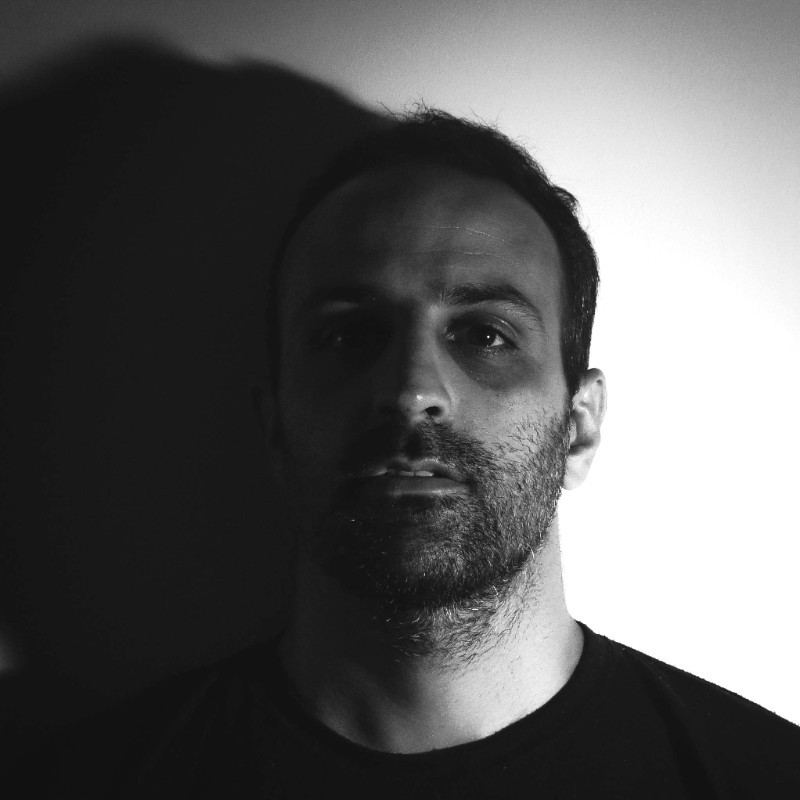
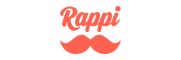