Getting Started with PHP’s number_format() Function
When working with numbers in PHP, it’s often essential to format them for better readability and presentation. The number_format() function is a valuable tool in a PHP developer’s arsenal for achieving just that. In this comprehensive guide, we’ll dive into the world of number_format(), exploring its features, usage, and providing practical examples to help you get started.
Table of Contents
1. What is number_format()?
PHP’s number_format() function is designed to format numbers in a human-readable way, making large or complex numbers more comprehensible to users. It allows you to apply thousands separators, specify decimal points, and control rounding. This is especially useful when dealing with financial data, statistical reports, or any application where presenting numbers clearly is crucial.
1.1. Basic Syntax
Before we delve into practical examples, let’s first take a look at the basic syntax of the number_format() function:
php string number_format(float $number, int $decimals = 0, string $decimal_separator = '.', string $thousands_separator = ',')
Here’s a breakdown of the parameters:
- $number (float): The number you want to format.
- $decimals (int, optional): The number of decimal places to include in the formatted number. Default is 0.
- $decimal_separator (string, optional): The character used to separate the decimal part from the integer part. Default is a period (‘.’).
- $thousands_separator (string, optional): The character used to separate thousands in the number. Default is a comma (‘,’).
1.2. Using number_format() for Basic Number Formatting
Let’s start with some simple examples to understand how number_format() works for basic number formatting.
Example 1: Formatting an Integer
php $number = 10000; $formatted_number = number_format($number); echo $formatted_number; // Output: 10,000
In this example, we format the integer 10000 using number_format(), which adds a comma as the thousands separator by default.
Example 2: Formatting a Floating-Point Number
php $number = 12345.6789; $formatted_number = number_format($number, 2); echo $formatted_number; // Output: 12,345.68
Here, we format the floating-point number 12345.6789 with two decimal places specified. The function rounds the number accordingly.
1.3. Customizing Decimal and Thousands Separators
You can easily customize the decimal and thousands separators according to your requirements.
Example 3: Custom Separators
php $number = 12345.6789; $formatted_number = number_format($number, 2, ',', '.'); echo $formatted_number; // Output: 12.345,68
In this example, we use a comma (‘,’) as the decimal separator and a period (‘.’) as the thousands separator.
2. Precision and Rounding
The number_format() function also offers control over the precision of formatted numbers. This is especially useful when dealing with financial calculations that require specific rounding behavior.
2.1. Rounding Options
Example 4: Rounding Up
php $number = 12.345; $formatted_number = number_format($number, 2, '.', ','); echo $formatted_number; // Output: 12.35
Here, we round up the number 12.345 to two decimal places.
Example 5: Rounding Down
php $number = 12.344; $formatted_number = number_format($number, 2, '.', ','); echo $formatted_number; // Output: 12.34
In this case, we round down the number 12.344 to two decimal places.
2.2. Handling Large Numbers
number_format() can handle large numbers gracefully by adding appropriate thousands separators.
Example 6: Formatting a Large Number
php $large_number = 1234567890; $formatted_large_number = number_format($large_number); echo $formatted_large_number; // Output: 1,234,567,890
Even with large numbers like 1234567890, number_format() makes the output more readable.
3. Use Cases
Now that we’ve covered the basics, let’s explore some common use cases for the number_format() function.
3.1. Financial Applications
Financial applications often require precision in formatting numbers. Whether it’s displaying currency values, interest rates, or stock prices, number_format() can help ensure that the information is presented accurately and clearly.
Example 7: Formatting Currency
php $price = 1999.99; $formatted_price = number_format($price, 2, '.', ','); echo "$" . $formatted_price; // Output: $1,999.99
In this example, we format a price of $1999.99 as a currency value with two decimal places.
3.2. Statistical Reports
When generating statistical reports or charts, it’s crucial to format large numbers to enhance their readability.
Example 8: Formatting Population Data
php $population = 78912345; $formatted_population = number_format($population); echo "World Population: " . $formatted_population; // Output: World Population: 78,912,345
Here, we format the world population figure for better presentation in a report.
3.3. E-commerce Websites
E-commerce websites often need to display product prices and quantities in a user-friendly manner.
Example 9: Formatting Product Prices
php $product_price = 49.99; $product_quantity = 5; $total_price = $product_price * $product_quantity; $formatted_product_price = number_format($product_price, 2, '.', ','); $formatted_total_price = number_format($total_price, 2, '.', ','); echo "Price per unit: $" . $formatted_product_price . "<br>"; echo "Total price for 5 units: $" . $formatted_total_price; // Output: Price per unit: $49.99 Total price for 5 units: $249.95
In this scenario, we format both the individual product price and the total price for 5 units.
Conclusion
The number_format() function in PHP is a powerful tool for enhancing the readability of numbers in various applications. Whether you’re working on financial software, statistical reports, or e-commerce websites, this function allows you to format numbers with ease. By customizing decimal and thousands separators and controlling precision, you can present numeric data in a clear and professional manner. As you continue to explore PHP, keep number_format() in your toolkit to ensure your numbers are always displayed in the most user-friendly way possible. Start using it today to make your numeric data more accessible and informative.
Table of Contents
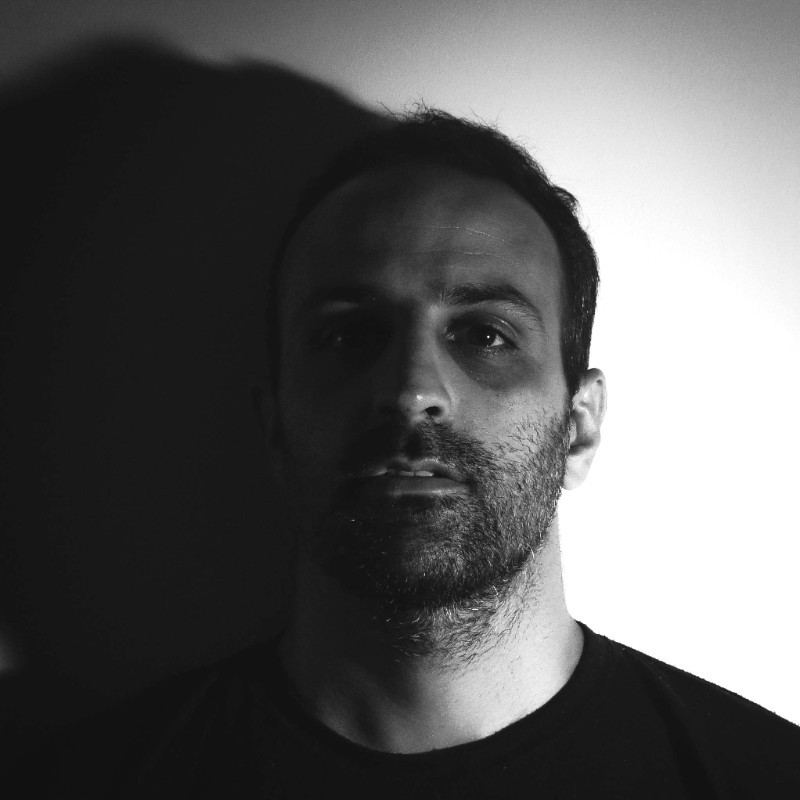
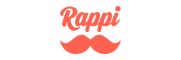