PHP Q & A
What is object-oriented programming (OOP)?
Object-oriented programming (OOP) is a programming paradigm used in PHP and many other programming languages to model real-world entities and organize code into reusable and structured components. In PHP, OOP involves creating classes and objects to encapsulate data (properties) and behaviors (methods) related to a particular entity or concept.
Key Concepts in OOP in PHP:
- Classes: Classes are blueprints or templates for creating objects. They define the structure and behavior of objects. In PHP, classes are declared using the `class` keyword.
- Objects: Objects are instances of classes. They represent specific instances of the data and behavior defined by a class. You can create multiple objects from a single class.
- Properties: Properties (also known as attributes or member variables) are variables that hold data specific to an object. They define the characteristics of an object.
- Methods: Methods are functions defined within a class. They encapsulate the behavior of the class and can manipulate the class’s properties.
- Encapsulation: Encapsulation is the principle of bundling data (properties) and methods (functions) that operate on that data within a single unit (class). It hides the internal implementation details of a class from external code.
- Inheritance: Inheritance allows one class (subclass or child class) to inherit properties and methods from another class (superclass or parent class). It promotes code reuse and establishes an “is-a” relationship between classes.
- Polymorphism: Polymorphism enables objects of different classes to be treated as objects of a common parent class. It allows for flexibility and dynamic behavior based on the specific object type.
Example:
```php class Animal { public $name; public function speak() { return "Animal speaks"; } } class Dog extends Animal { public function speak() { return "Woof!"; } } $dog = new Dog(); $dog->name = "Buddy"; echo $dog->speak(); // Outputs: "Woof!" ```
In PHP, OOP enhances code organization, promotes reusability, and facilitates the modeling of complex systems by representing real-world entities as objects. It’s a fundamental concept for building maintainable and scalable applications.
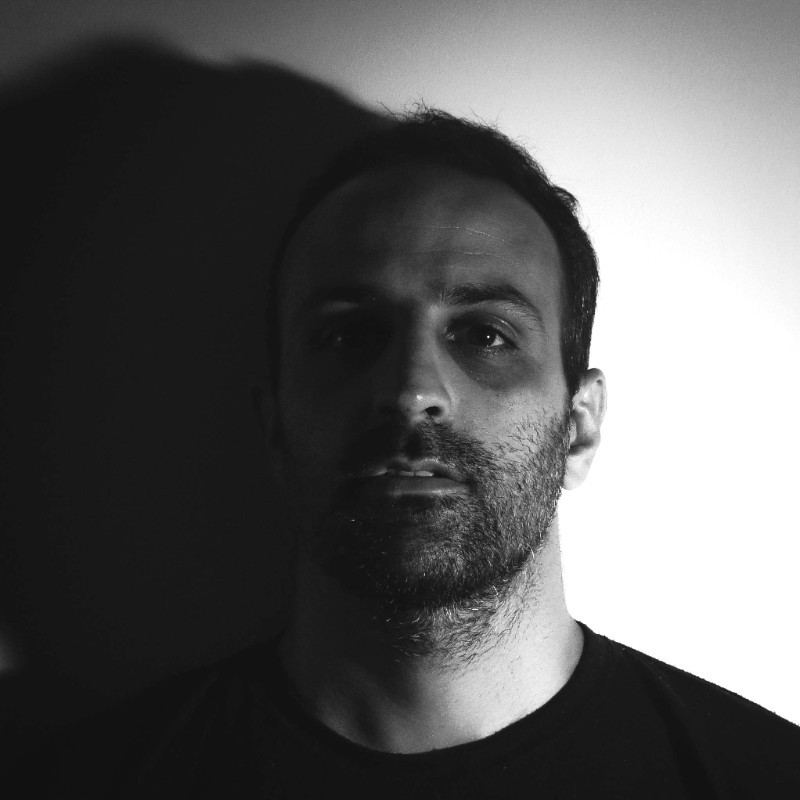
Previously at
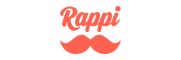
Full Stack Engineer with extensive experience in PHP development. Over 11 years of experience working with PHP, creating innovative solutions for various web applications and platforms.