Exploring Object-Oriented Programming in PHP
Object-Oriented Programming (OOP) is a powerful paradigm that allows developers to organize code into reusable and modular components. PHP, a popular server-side scripting language, provides extensive support for OOP. Whether you are new to PHP or an experienced developer looking to level up your skills, this comprehensive guide will help you understand the principles of OOP and demonstrate how to implement them effectively in PHP.
Understanding Object-Oriented Programming
Object-Oriented Programming is a programming paradigm that focuses on organizing code into objects, which are instances of classes. It revolves around the concepts of encapsulation, inheritance, and polymorphism. OOP enables developers to create reusable and modular code by defining classes that encapsulate data and behavior.
Classes and Objects
In PHP, classes are the blueprints for creating objects. They define the properties (variables) and methods (functions) that objects of that class will possess. Here’s an example of a simple class in PHP:
php class Car { public $brand; public $model; public function startEngine() { echo "Engine started!"; } } To create an object from the class, we use the new keyword: php $myCar = new Car(); We can then access the properties and methods of the object using the arrow operator (->): bash $myCar->brand = "Toyota"; $myCar->model = "Camry"; $myCar->startEngine();
Encapsulation
Encapsulation is the practice of hiding internal implementation details and exposing only the necessary interfaces to interact with an object. In PHP, we can achieve encapsulation by using access modifiers like public, private, and protected to control the visibility of properties and methods within a class. For example:
php class Person { private $name; protected $age; public function setName($name) { $this->name = $name; } public function getName() { return $this->name; } public function setAge($age) { $this->age = $age; } public function getAge() { return $this->age; } }
In the above example, the $name property is private and can only be accessed within the class, while the $age property is protected and can be accessed within the class and its subclasses.
Inheritance
Inheritance allows classes to inherit properties and methods from other classes. In PHP, we can achieve inheritance using the extends keyword. Let’s consider an example:
php class Animal { protected $name; public function eat() { echo "The animal is eating."; } } class Dog extends Animal { public function bark() { echo "Woof! Woof!"; } }
In the above example, the Dog class extends the Animal class, inheriting its $name property and eat() method. Additionally, the Dog class introduces its own bark() method.
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. This enables us to write code that can work with different types of objects interchangeably. Here’s an example:
php class Shape { public function draw() { echo "Drawing a shape."; } } class Circle extends Shape { public function draw() { echo "Drawing a circle."; } } class Square extends Shape { public function draw() { echo "Drawing a square."; } }
In the above example, both the Circle and Square classes inherit from the Shape class and override the draw() method. We can then treat objects of these classes as objects of the Shape class:
scss function drawShape(Shape $shape) { $shape->draw(); } $circle = new Circle(); $square = new Square(); drawShape($circle); // Outputs: Drawing a circle. drawShape($square); // Outputs: Drawing a square.
Abstract Classes and Interfaces
Abstract classes and interfaces are used to define common behavior that classes should implement. An abstract class can provide both concrete and abstract methods, while an interface can only define method signatures. Here’s an example:
php abstract class Animal { abstract public function makeSound(); } interface Flyable { public function fly(); } class Dog extends Animal { public function makeSound() { echo "Woof! Woof!"; } } class Bird extends Animal implements Flyable { public function makeSound() { echo "Chirp! Chirp!"; } public function fly() { echo "The bird is flying."; } }
In the above example, the Animal class is an abstract class that defines the makeSound() method. The Bird class implements both the makeSound() method from the Animal class and the fly() method from the Flyable interface.
Namespaces
Namespaces are used to organize classes, functions, and constants into logical groups, preventing naming conflicts. They provide a way to group related code together and make it easier to maintain and reuse. Here’s an example:
csharp namespace MyNamespace; class MyClass { // Class definition } function myFunction() { // Function implementation } const MY_CONSTANT = 42;
In the above example, the MyClass, myFunction(), and MY_CONSTANT are all part of the MyNamespace namespace.
Autoloading Classes
PHP provides autoloading mechanisms to automatically load classes when they are first accessed. This eliminates the need for manually including class files. The spl_autoload_register() function is commonly used to register autoloading functions. Here’s a simple autoloader example:
bash spl_autoload_register(function ($className) { $filePath = str_replace('\\', '/', $className) . '.php'; if (file_exists($filePath)) { require_once($filePath); } });
In the above example, the autoloader function takes the class name, converts it to a file path, and includes the file if it exists.
Traits
Traits provide a way to reuse methods in multiple classes without using inheritance. They allow developers to horizontally share methods across unrelated classes. Here’s an example:
php trait Logger { public function log($message) { echo "Logging: $message"; } } class User { use Logger; public function register() { // Registration logic $this->log('User registered.'); } }
In the above example, the Logger trait provides the log() method, which can be used in the User class through the use statement.
Best Practices for Object-Oriented Programming in PHP
Follow the Single Responsibility Principle (SRP): Each class should have a single responsibility.
- Use meaningful and descriptive class and method names to improve code readability.
- Use type hints and doc comments to enhance code documentation and maintainability.
- Apply dependency injection to decouple classes and improve testability.
- Strive for loose coupling and high cohesion between classes.
- Avoid using global state and static methods excessively.
- Utilize design patterns to solve common problems in object-oriented code.
Conclusion
Object-Oriented Programming in PHP offers a robust and efficient way to develop modular and reusable code. By understanding the principles and concepts of OOP, you can build maintainable and scalable applications. In this blog, we explored the fundamental aspects of OOP in PHP, including classes, objects, encapsulation, inheritance, polymorphism, abstract classes, interfaces, namespaces, autoloading classes, and traits.
By applying these concepts and following best practices, you’ll be well-equipped to leverage the power of OOP and develop high-quality PHP applications. So, go ahead, dive into the world of Object-Oriented Programming, and unlock the true potential of PHP!
Table of Contents
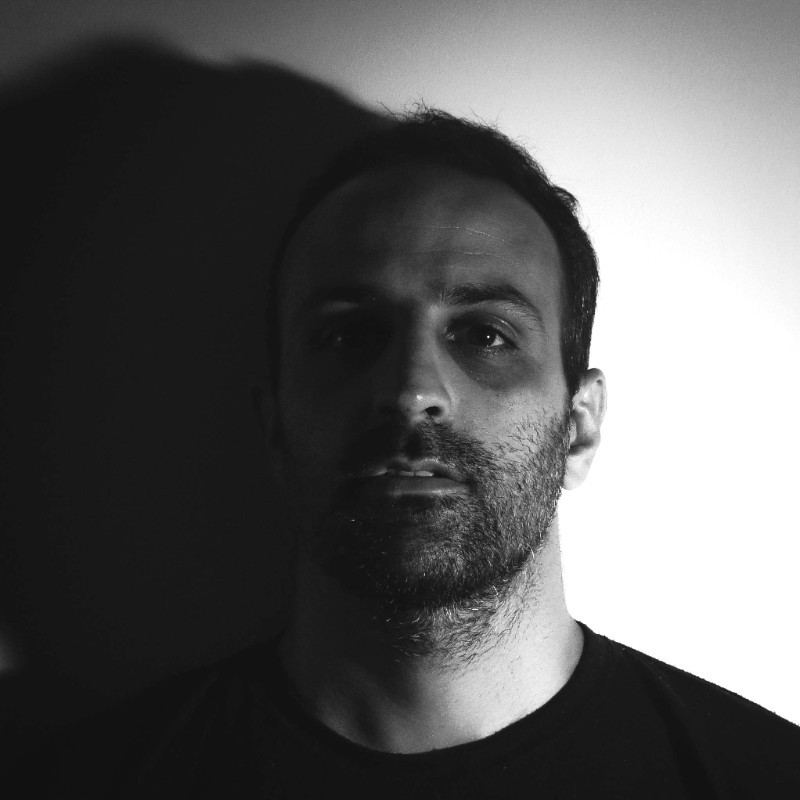
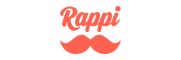