PHP Operators: A Complete Breakdown
As a versatile scripting language, PHP offers a wide range of operators that allow developers to perform various operations on variables, values, and expressions. Understanding these operators is crucial for writing efficient and effective PHP code. In this blog, we will dive deep into the world of PHP operators, exploring each category, and providing code samples along the way.
1. Arithmetic Operators
Arithmetic operators are used to perform basic mathematical operations in PHP. They work on numeric values and variables. Let’s explore each one with code examples:
1.1. Addition (+)
The addition operator is used to add two numeric values together.
php $sum = 5 + 3; // $sum will be 8
1.2. Subtraction (-)
The subtraction operator is used to subtract one numeric value from another.
php $difference = 10 - 5; // $difference will be 5
1.3. Multiplication (*)
The multiplication operator is used to multiply two numeric values.
php $product = 2 * 4; // $product will be 8
1.4. Division (/)
The division operator is used to divide one numeric value by another.
php $quotient = 20 / 4; // $quotient will be 5
1.5. Modulus (%)
The modulus operator is used to find the remainder of the division of one number by another.
php $remainder = 17 % 3; // $remainder will be 2
1.6. Increment (++)
The increment operator is used to increase the value of a variable by 1.
php $counter = 5; $counter++; // $counter will be 6
1.7. Decrement (–)
The decrement operator is used to decrease the value of a variable by 1.
php $counter = 5; $counter--; // $counter will be 4
2. Assignment Operators
Assignment operators are used to assign values to variables. They combine arithmetic operations with variable assignment. Let’s examine each one:
2.1. Assignment (=)
The assignment operator is used to assign a value to a variable.
php $name = "John"; // $name is now "John"
2.2. Addition Assignment (+=)
The addition assignment operator is used to add a value to the variable and assign the result back to the variable.
php $counter = 10; $counter += 5; // $counter is now 15
2.3. Subtraction Assignment (-=)
The subtraction assignment operator is used to subtract a value from the variable and assign the result back to the variable.
php $counter = 20; $counter -= 8; // $counter is now 12
2.4. Multiplication Assignment (*=)
The multiplication assignment operator is used to multiply the variable by a value and assign the result back to the variable.
php $factor = 3; $number = 7; $number *= $factor; // $number is now 21
2.5. Division Assignment (/=)
The division assignment operator is used to divide the variable by a value and assign the result back to the variable.
php $total = 100; $parts = 4; $total /= $parts; // $total is now 25
2.6. Modulus Assignment (%=)
The modulus assignment operator is used to find the remainder of the division of the variable by a value and assign the result back to the variable.
php $number = 15; $divider = 4; $number %= $divider; // $number is now 3
3. Comparison Operators
Comparison operators are used to compare two values or variables. They return a Boolean value (true or false) based on the comparison. Let’s explore each one:
3.1. Equal (==)
The equal operator checks if two values are equal.
php $num1 = 10; $num2 = "10"; if ($num1 == $num2) { echo "Equal"; } else { echo "Not Equal"; }
Output:
mathematica Equal
3.2. Identical (===)
The identical operator checks if two values are equal and of the same data type.
php $num1 = 10; $num2 = "10"; if ($num1 === $num2) { echo "Identical"; } else { echo "Not Identical"; }
Output:
mathematica Not Identical
3.3. Not Equal (!=)
The not equal operator checks if two values are not equal.
php $age = 25; if ($age != 18) { echo "Not 18 years old"; } else { echo "18 years old"; }
Output:
mathematica Not 18 years old
3.4. Not Identical (!==)
The not identical operator checks if two values are not equal or not of the same data type.
php $num1 = 5; $num2 = "5"; if ($num1 !== $num2) { echo "Not Identical"; } else { echo "Identical"; }
Output:
mathematica Not Identical
3.5. Greater Than (>)
The greater than operator checks if the value on the left is greater than the value on the right.
php $score = 85; if ($score > 80) { echo "Excellent!"; } else { echo "Keep it up!"; }
Output:
Excellent!
3.6. Less Than (<)
The less than operator checks if the value on the left is less than the value on the right.
php $temperature = 25; if ($temperature < 30) { echo "It's not too hot."; } else { echo "It's quite hot!"; }
Output:
rust It's not too hot.
3.7. Greater Than or Equal To (>=)
The greater than or equal to operator checks if the value on the left is greater than or equal to the value on the right.
php $marks = 75; if ($marks >= 70) { echo "Passed with distinction!"; } else { echo "Keep working hard!"; }
Output:
csharp Passed with distinction!
3.8. Less Than or Equal To (<=)
The less than or equal to operator checks if the value on the left is less than or equal to the value on the right.
php $quantity = 8; if ($quantity <= 10) { echo "Available in stock"; } else { echo "Out of stock"; }
Output:
Available in stock
4. Logical Operators
Logical operators are used to perform logical operations on conditional statements. They return a Boolean value based on the conditions evaluated. Let’s explore each one:
4.1. And (&&)
The “and” operator returns true if both conditions on the left and right are true.
php $age = 25; $location = "USA"; if ($age > 21 && $location == "USA") { echo "You are eligible."; } else { echo "You are not eligible."; }
Output:
sql You are eligible.
4.2. Or (||)
The “or” operator returns true if at least one of the conditions on the left or right is true.
php $day = "Saturday"; if ($day == "Saturday" || $day == "Sunday") { echo "It's the weekend!"; } else { echo "It's a weekday."; }
Output:
rust It's the weekend!
4.3. Not (!)
The “not” operator returns the opposite Boolean value of the condition.
php $isSunny = false; if (!$isSunny) { echo "It's a cloudy day."; } else { echo "It's a sunny day!"; }
Output:
css It's a cloudy day.
5. Bitwise Operators
Bitwise operators perform operations on individual bits of numeric values. They are rarely used in everyday programming but can be valuable in certain situations.
5.1. And (&)
The bitwise “and” operator performs a bitwise AND operation on two integers.
php $bit1 = 5; // Binary: 101 $bit2 = 3; // Binary: 011 $result = $bit1 & $bit2; // Binary: 001 (Decimal: 1)
5.2. Or (|)
The bitwise “or” operator performs a bitwise OR operation on two integers.
php $bit1 = 5; // Binary: 101 $bit2 = 3; // Binary: 011 $result = $bit1 | $bit2; // Binary: 111 (Decimal: 7)
5.3. Xor (^)
The bitwise “xor” operator performs a bitwise exclusive OR operation on two integers.
php $bit1 = 5; // Binary: 101 $bit2 = 3; // Binary: 011 $result = $bit1 ^ $bit2; // Binary: 110 (Decimal: 6)
5.4. Not (~)
The bitwise “not” operator performs a bitwise NOT operation on a single integer.
php $bit = 5; // Binary: 101 $result = ~$bit; // Binary: 11111111111111111111111111111010 (Decimal: -6)
5.5. Left Shift (<<)
The left shift operator shifts the bits of the left operand to the left by the number of positions specified by the right operand.
php $bit = 5; // Binary: 101 $result = $bit << 2; // Binary: 10100 (Decimal: 20)
5.6. Right Shift (>>)
The right shift operator shifts the bits of the left operand to the right by the number of positions specified by the right operand.
php $bit = 16; // Binary: 10000 $result = $bit >> 2; // Binary: 100 (Decimal: 4)
6. Conditional (Ternary) Operator
The conditional operator is a shorthand way of writing if-else statements. It evaluates an expression and returns one of two values based on whether the expression is true or false.
6.1. Syntax
php $variable = (condition) ? value_if_true : value_if_false;
6.2. Example
php $age = 20; $ticketType = ($age < 18) ? "Child" : "Adult"; echo $ticketType;
Output:
Adult
Conclusion
PHP operators are the building blocks of any PHP script, enabling developers to manipulate data, perform comparisons, and make decisions. By mastering these operators, you gain the ability to write more efficient and robust PHP code. We hope this comprehensive breakdown has provided you with valuable insights and a deeper understanding of PHP operators.
Remember to practice and experiment with these operators to solidify your knowledge and enhance your PHP programming skills. Happy coding!
Table of Contents
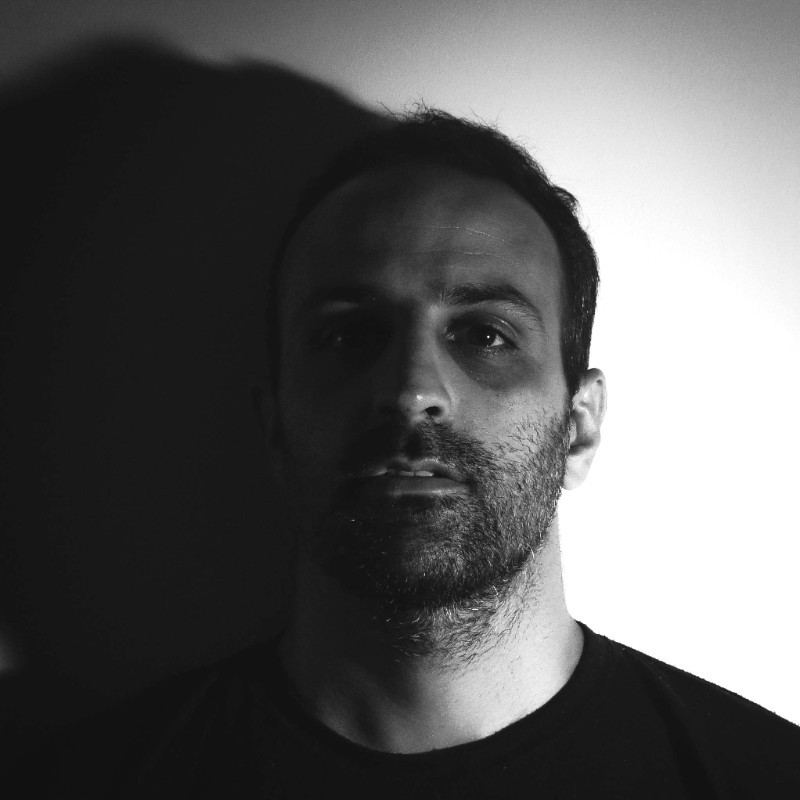
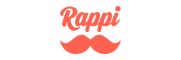