Optimizing Your PHP Code: Best Practices
PHP (Hypertext Preprocessor) is a popular server-side scripting language used for web development. It’s known for its flexibility and ease of use, but poorly optimized PHP code can lead to slow-loading web pages and inefficient server performance. In this article, we’ll dive into essential best practices for optimizing your PHP code, ensuring your web applications run smoothly and efficiently.
1. Introduction to PHP Code Optimization
1.1. Why Code Optimization Matters:
Code optimization is crucial because it directly impacts the performance and user experience of your web applications. Well-optimized code ensures faster load times, reduced server load, and improved scalability. With the ever-increasing demand for responsive and quick websites, neglecting code optimization can lead to high bounce rates and dissatisfied users.
1.2. Benefits of Optimized Code:
- Faster Loading Times: Optimized code executes faster, resulting in quicker web page load times.
- Reduced Server Load: Efficient code consumes fewer server resources, allowing your application to handle more concurrent users.
- Improved User Experience: Fast-loading pages enhance user experience, leading to higher engagement and lower bounce rates.
- Better SEO Rankings: Search engines consider page speed as a ranking factor, making optimization essential for SEO success.
2. Profiling and Performance Measurement
2.1. Identifying Bottlenecks:
Before optimizing your PHP code, it’s crucial to identify performance bottlenecks. Use profiling tools to pinpoint which parts of your code consume the most resources and contribute to slower execution times. Tools like Xdebug and Blackfire provide insights into function calls, memory usage, and execution times.
2.2. Profiling Tools:
- Xdebug: This powerful PHP extension offers profiling capabilities, generating detailed reports about function calls, memory usage, and execution times.
- Blackfire: A premium performance profiling tool that helps you visualize and analyze your application’s performance, offering recommendations for improvements.
2.3. Example of using Xdebug for profiling:
php // Enable profiling xdebug_start_trace('trace_output'); // Your PHP code here // Stop profiling xdebug_stop_trace();
3. Use the Latest PHP Version
3.1. Performance Improvements in New Versions:
PHP developers continually work on improving the language’s performance in each new release. Upgrading to the latest PHP version can provide significant performance enhancements. New versions often include optimizations, bug fixes, and new features that can positively impact your application’s speed and efficiency.
3.2. Security Benefits:
Using the latest PHP version ensures that you have access to the most up-to-date security patches, protecting your application from potential vulnerabilities.
Example:
php // PHP 7.4 $sum = array_sum([1, 2, 3, 4]); // PHP 8.0 $sum = array_sum([1, 2, 3, 4]);
4. Efficient Database Queries
4.1. Avoiding N+1 Query Problem:
When working with databases, the N+1 query problem can lead to performance issues. This occurs when you retrieve a list of items and then make an additional query for each item to fetch related data. Use eager loading or JOINs to retrieve all necessary data in a single query, reducing the overall number of queries.
4.2. Indexing for Faster Retrieval:
Ensure your database tables are properly indexed to speed up query retrieval. Indexes improve data retrieval times by creating a structured lookup mechanism.
Example of avoiding N+1 query problem:
php // Inefficient N+1 queries $posts = Post::all(); foreach ($posts as $post) { $comments = $post->comments; } // Efficient solution using eager loading $posts = Post::with('comments')->get();
5. Caching Strategies
5.1. OpCode Caching:
PHP opcode caching stores precompiled script bytecode in memory, reducing the need for PHP to recompile scripts on every request. Popular opcode caching tools include OPcache and APCu.
5.2. Data Caching with Memcached/Redis:
For frequently accessed data, use caching systems like Memcached or Redis. These tools store data in memory, providing lightning-fast access and reducing the load on your database.
Example of using Memcached for caching:
php $memcached = new Memcached(); $memcached->addServer('localhost', 11211); $key = 'user_123'; $userData = $memcached->get($key); if (!$userData) { $userData = // Fetch user data from the database $memcached->set($key, $userData, 3600); // Cache for 1 hour }
6. Minimize File I/O Operations
6.1. Include vs. Require:
Minimize file I/O operations by using require instead of include for essential files. While include is more forgiving, require ensures that your application doesn’t proceed without necessary components.
6.2. File Read/Write Optimization:
Reduce file read/write operations by caching data or using memory-based storage solutions. Frequent file operations can slow down your application, especially when dealing with large files.
6.3. Example of using require:
php // Instead of using include include 'config.php'; // Use require for essential files require 'config.php';
7. Optimize Loops and Conditionals
7.1. Loop Unrolling:
Loop unrolling involves manually expanding loop iterations to reduce overhead from loop control statements. This can lead to performance improvements, especially in loops with a fixed number of iterations.
7.2. Early Exit in Conditionals:
In situations where you can quickly determine the outcome of a conditional statement, use an early exit to prevent unnecessary code execution.
Example of loop unrolling:
php // Without loop unrolling for ($i = 0; $i < 10; $i++) { // Loop body } // With loop unrolling for ($i = 0; $i < 10; $i += 2) { // Loop body for even iterations }
8. String Manipulation Techniques
8.1. StringBuilder Pattern:
When building complex strings, use the StringBuilder pattern instead of directly concatenating strings. This reduces memory overhead and enhances performance.
8.2. Use Single Quotes for Literal Strings:
When working with literal strings that don’t require variable interpolation, use single quotes (‘) instead of double quotes (“). Single quotes are marginally faster.
Example of using the StringBuilder pattern:
php // Without StringBuilder $message = 'Hello, ' . $user->getName() . '! You have ' . count($notifications) . ' notifications.'; // With StringBuilder pattern $stringBuilder = new StringBuilder(); $stringBuilder->append('Hello, ')->append($user->getName())->append('! You have ')->append(count($notifications))->append(' notifications.'); $message = $stringBuilder->toString();
9. Memory Management
9.1. Limiting Memory Usage:
Avoid consuming excessive memory by optimizing data structures and releasing unused resources. Bloated memory usage can lead to slower application performance and even crashes.
9.2. Unsetting Variables and Objects:
Manually unset variables and objects when they’re no longer needed. This frees up memory and ensures efficient memory management.
Example of limiting memory usage:
php // Unset variables and objects when done using them $largeData = // Load large dataset processData($largeData); unset($largeData);
10. Proper Error Handling
10.1. Try-Catch Blocks:
Use try-catch blocks to handle exceptions gracefully. Catching and handling exceptions prevents your application from crashing and provides valuable insights into potential issues.
10.2. Logging and Debugging:
Implement robust logging mechanisms to track errors and exceptions. Proper logging helps you diagnose problems and fine-tune your code for better performance.
Example of using try-catch blocks:
php try { // Code that might throw an exception } catch (Exception $e) { // Handle the exception logException($e); displayErrorMessage(); }
11. Code Reusability and Modularity
11.1. Using Functions and Classes:
Promote code reusability by encapsulating logic within functions and classes. This allows you to modularize your codebase and avoid code duplication.
11.2. Autoloading:
Leverage autoloading to efficiently load classes only when they’re needed. Autoloading prevents unnecessary memory usage and speeds up the application’s startup time.
Example of using classes and autoloading:
php // MyClass.php class MyClass { // Class methods and properties } // Using autoloading spl_autoload_register(function ($className) { include $className . '.php'; }); $obj = new MyClass();
Conclusion
Optimizing your PHP code is a continuous process that involves careful consideration of various factors. By following these best practices, you can significantly enhance the performance, efficiency, and scalability of your web applications. Remember that each optimization may have a unique impact based on your application’s architecture, so regular profiling and testing are essential to ensure consistent improvements. With the right approach, your PHP applications can deliver a seamless user experience while efficiently utilizing server resources.
Table of Contents
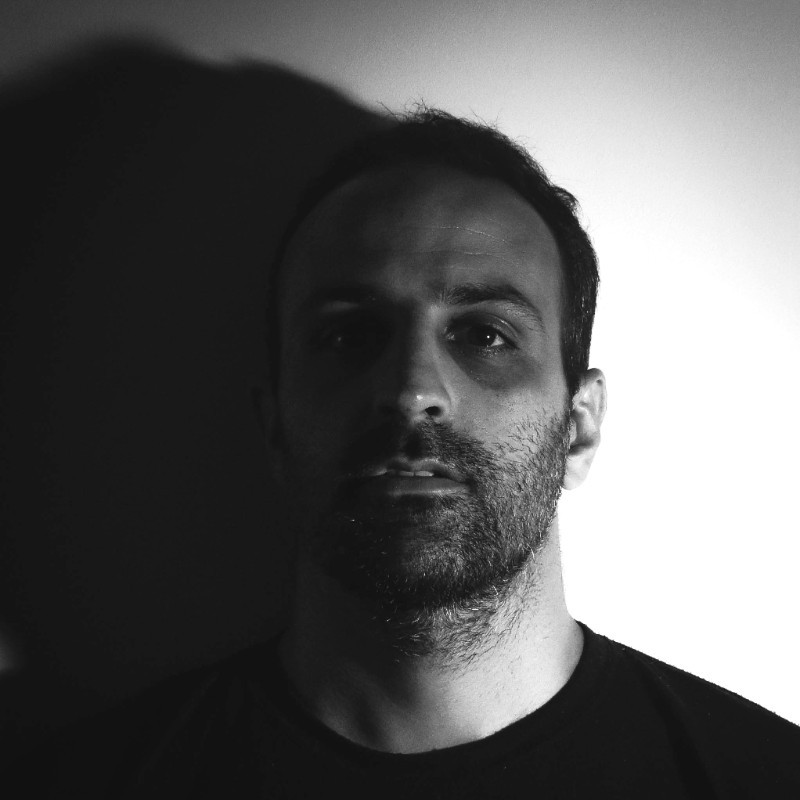
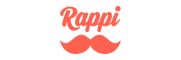