Understanding the PHP’s pathinfo() Function
PHP is a versatile scripting language used for web development. It provides a rich set of functions and libraries to work with files and directories. One such function that often comes in handy is pathinfo(). This function allows developers to extract valuable information from file paths effortlessly. In this blog post, we will dive deep into understanding PHP’s pathinfo() function, its syntax, use cases, and provide practical examples to demonstrate its power.
Table of Contents
1. What is the pathinfo() Function?
The pathinfo() function in PHP is a built-in function that extracts information about a file path. It takes a file path as input and returns an associative array containing various pieces of information about that path. This information includes the directory name, base name (file name with extension), file name without extension, and file extension.
Here’s the basic syntax of the pathinfo() function:
php pathinfo($path, $options);
- $path: This is the input file path from which you want to extract information.
- $options (optional): An integer value that can be used to specify what information you want to retrieve. This parameter is available in PHP 7.2 and later versions.
2. Components of the Returned Array
The pathinfo() function returns an associative array with the following keys:
- dirname: The directory name of the file path.
- basename: The base name of the file path (including the file extension).
- filename: The base name of the file path (excluding the file extension).
- extension: The file extension of the file path (if present).
Now, let’s break down each of these components and explore how you can use them.
3. Extracting the Directory Name
To extract the directory name from a file path using the pathinfo() function, you can access the dirname key in the returned array. Here’s an example:
php <?php $path = '/path/to/your/file.txt'; $info = pathinfo($path); $directoryName = $info['dirname']; echo "Directory Name: $directoryName"; ?>
In this example, the value of $directoryName will be /path/to/your.
4. Extracting the Base Name
The base name is the file name along with its extension. You can obtain this information using the basename key in the array returned by pathinfo():
php <?php $path = '/path/to/your/file.txt'; $info = pathinfo($path); $baseName = $info['basename']; echo "Base Name: $baseName"; ?>
The value of $baseName in this example will be file.txt.
5. Extracting the File Name Without Extension
To get the file name without its extension, you can use the filename key:
php <?php $path = '/path/to/your/file.txt'; $info = pathinfo($path); $fileNameWithoutExtension = $info['filename']; echo "File Name Without Extension: $fileNameWithoutExtension"; ?>
The value of $fileNameWithoutExtension will be file.
6. Extracting the File Extension
If you need to extract the file extension, you can use the extension key:
php <?php $path = '/path/to/your/file.txt'; $info = pathinfo($path); $fileExtension = $info['extension']; echo "File Extension: $fileExtension"; ?>
In this example, the value of $fileExtension will be txt.
7. Use Cases for pathinfo()
Now that you understand the basic components of the pathinfo() function, let’s explore some practical use cases where this function can be incredibly useful.
7.1. File Uploads
When building a web application that allows users to upload files, you often need to analyze the uploaded file’s information. The pathinfo() function can be handy for extracting details like the file’s name and extension, which can then be used for further processing or storage.
php <?php if ($_FILES['uploaded_file']['error'] === UPLOAD_ERR_OK) { $uploadedFile = $_FILES['uploaded_file']['tmp_name']; $info = pathinfo($_FILES['uploaded_file']['name']); $fileName = $info['filename']; $fileExtension = $info['extension']; // Process the uploaded file based on its name and extension. } ?>
7.2. Building Custom URLs
In web applications, you might need to create custom URLs based on file names. Using pathinfo(), you can easily extract the file name without the extension and use it to generate user-friendly URLs.
php <?php $path = '/path/to/your/file.txt'; $info = pathinfo($path); $fileNameWithoutExtension = $info['filename']; $customURL = "/articles/{$fileNameWithoutExtension}"; // $customURL will be '/articles/file' ?>
7.3. Organizing Files
If you’re working with a large number of files, organizing them into directories based on their extensions can be a practical approach. The pathinfo() function helps you classify files by extracting their extensions.
php <?php $files = ['/path/to/file1.jpg', '/path/to/file2.png', '/path/to/file3.txt']; foreach ($files as $file) { $info = pathinfo($file); $fileExtension = $info['extension']; // Move the file to the corresponding directory based on its extension. } ?>
7.4. Generating Dynamic Content
When building a content management system or a blogging platform, you might want to generate dynamic content based on file names. pathinfo() can assist in extracting the necessary information from the file path to display content appropriately.
php <?php $requestPath = '/articles/my-article'; // Extract the requested article name from the path. $info = pathinfo($requestPath); $articleName = $info['filename']; // Retrieve and display content based on the article name. ?>
8. Additional Options Parameter
As mentioned earlier, the pathinfo() function accepts an optional $options parameter that allows you to specify which components you want to retrieve. This parameter was introduced in PHP 7.2. The $options parameter can take one of the following constants:
- PATHINFO_DIRNAME: Returns only the directory name.
- PATHINFO_BASENAME: Returns only the base name (including the extension).
- PATHINFO_FILENAME: Returns only the base name (excluding the extension).
- PATHINFO_EXTENSION: Returns only the extension.
Here’s how you can use the $options parameter:
php <?php $path = '/path/to/your/file.txt'; // Get only the directory name. $directoryName = pathinfo($path, PATHINFO_DIRNAME); // Get only the base name (including the extension). $baseName = pathinfo($path, PATHINFO_BASENAME); // Get only the base name (excluding the extension). $fileNameWithoutExtension = pathinfo($path, PATHINFO_FILENAME); // Get only the extension. $fileExtension = pathinfo($path, PATHINFO_EXTENSION); ?>
By specifying the desired option, you can retrieve specific information without having to access the individual keys in the returned array.
9. Handling Edge Cases
While the pathinfo() function is incredibly useful for extracting information from typical file paths, it’s important to be aware of potential edge cases and issues you might encounter.
9.1. Missing Components
In some cases, a file path might not have all the components that pathinfo() expects. For example, if you provide a path that consists only of a file name without a directory, the dirname component will be an empty string.
php <?php $path = 'file.txt'; $info = pathinfo($path); echo "Directory Name: {$info['dirname']}"; // Output: Directory Name: ?>
9.2. Non-Standard File Paths
pathinfo() assumes a standard file path format, where the directory separator is / on Unix-like systems and \ on Windows. If you’re working with file paths that use a different separator, you might not get the expected results.
php <?php $path = 'C:\path\to\your\file.txt'; $info = pathinfo($path); echo "Directory Name: {$info['dirname']}"; // Output: Directory Name: C:\path\to\your ?>
9.3. Unicode and Multibyte Characters
pathinfo() may not behave as expected when working with file paths that contain Unicode characters or multibyte character encodings. You may need to handle character encoding issues separately if you encounter such paths.
Conclusion
PHP’s pathinfo() function is a valuable tool for working with file paths, allowing you to easily extract information like directory names, file names, extensions, and more. Whether you’re building file upload functionality, generating custom URLs, or organizing files, pathinfo() simplifies the process by providing a structured way to access file path components. Just keep in mind the potential edge cases and issues discussed in this guide to ensure robust handling of file paths in your PHP applications.
Incorporating pathinfo() into your PHP development toolkit can streamline your code and enhance the efficiency and maintainability of your web applications. So, the next time you find yourself working with file paths, remember this versatile function and how it can simplify your tasks.
Table of Contents
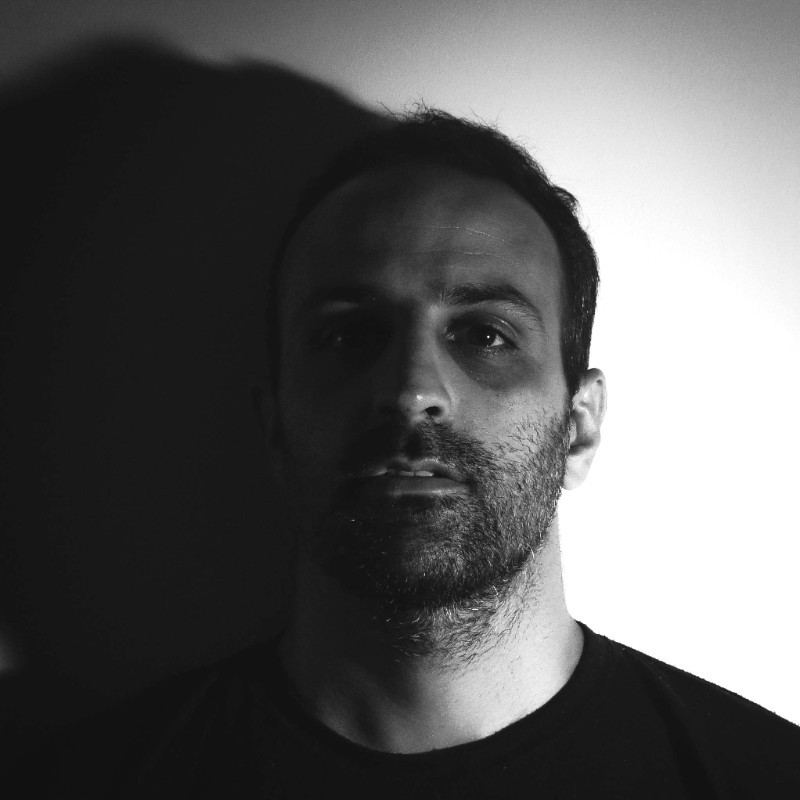
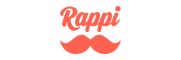