How to perform database queries?
Performing database queries in PHP is a fundamental skill for building dynamic web applications that interact with databases like MySQL. Here’s a step-by-step guide on how to execute database queries in PHP:
- Establish a Database Connection:
– Before performing queries, establish a connection to your database as explained in the previous response. Use the `mysqli_connect()` function to connect to the database server.
- Write SQL Queries:
– Write SQL queries to perform the desired database operations. For example, to retrieve data from a table, you can use a SELECT query:
```php $sql = "SELECT * FROM users WHERE username='john_doe'"; ```
- Execute Queries:
– Use the `mysqli_query()` function to execute your SQL queries. Pass the database connection and the SQL query as arguments.
```php $result = mysqli_query($conn, $sql); ```
- Handling Query Results:
– Depending on the type of query, you may receive different types of results. For SELECT queries, you typically get a result set that needs to be processed. Use functions like `mysqli_fetch_assoc()` or `mysqli_fetch_array()` to retrieve rows from the result set.
```php while ($row = mysqli_fetch_assoc($result)) { // Process data here } ```
- Error Handling:
– Always check for errors after executing a query. Use functions like `mysqli_error()` to retrieve error messages and handle them gracefully.
```php if (!$result) { die("Query failed: " . mysqli_error($conn)); } ```
- Closing the Connection:
– After executing all required queries, it’s essential to close the database connection using `mysqli_close($conn)` to release server resources.
By following these steps, you can effectively perform database queries in PHP, allowing you to interact with your database, retrieve data, update records, and perform other essential operations needed to build dynamic web applications.
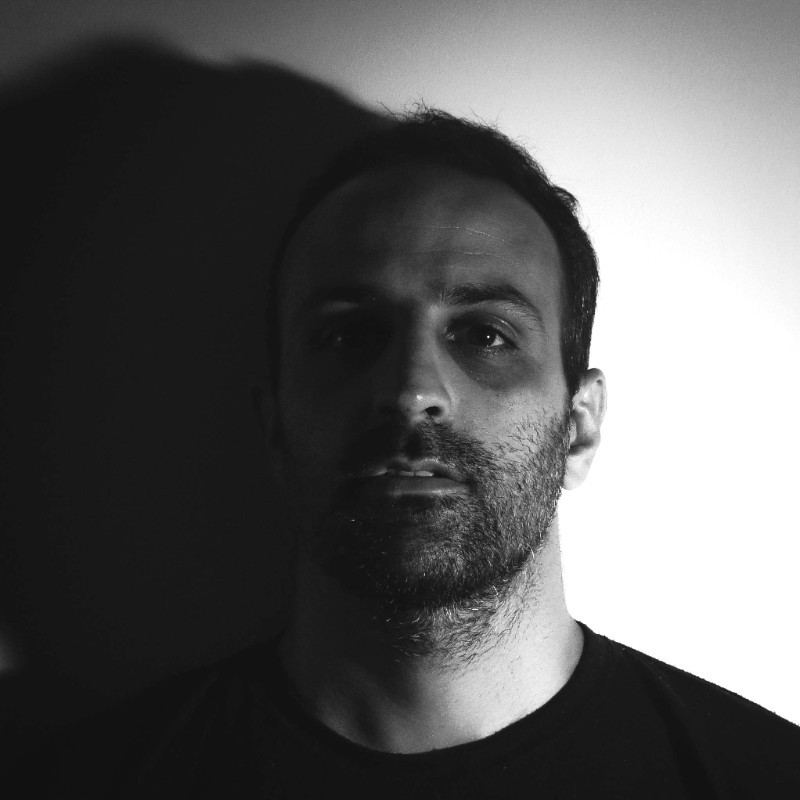
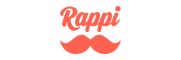